Script - log to text file
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 10-16-2019 03:51 AM
I am trying something quite easy, but cannot find the command where it works
So like following command
find /library/etc -name '*.rtf" - type -f delete
I want to see deleted files in log file. So I tried with > /path/txt.log also | tee path etc
Logfiles are created, but they are just empty
Any one has the secret what I am missing ?
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 10-16-2019 06:00 AM
A little brute force, but I use the following when I want all output in a log: set -xv; exec 1> $LOGFILE 2>&1
The variable $LOGFILE
points to whatever log file I want to save to.
Showing the running command in a bash script with "set -x"
The exec 1>$LOGFILE 2>&1
bit redirects stdout (1) to the logfile and stderr (2) to stdout.
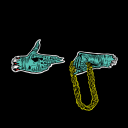
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 10-16-2019 10:55 AM
You should build a logger, and then code logic and error handling in it to send data to a log file
zsh example:
#!/bin/zsh
# example log function
LOGFILE="/var/log/mylog.log"
logger() {
echo $(date "+%Y-%m-%d %H:%M:%S ") $1 >> "${LOGFILE}"
}
logger "this is a test of the emergency broadcast system"
logger "[ERROR] non zero exit status has occurred..."
logger "[WARN] non standard config detected..."
output:
% sudo zsh -x logger.sh
+logger.sh:5> LOGFILE=/var/log/mylog.log
+logger.sh:12> logger 'this is a test of the emergency broadcast system'
+logger:1> date '+%Y-%m-%d %H:%M:%S '
+logger:1> echo 2019-10-16 10:49:05 'this is a test of the emergency broadcast system'
+logger.sh:13> logger '[ERROR] non zero exit status has occurred...'
+logger:1> date '+%Y-%m-%d %H:%M:%S '
+logger:1> echo 2019-10-16 10:49:05 '[ERROR] non zero exit status has occurred...'
+logger.sh:14> logger '[WARN] non standard config detected...'
+logger:1> date '+%Y-%m-%d %H:%M:%S '
+logger:1> echo 2019-10-16 10:49:05 '[WARN] non standard config detected...'
The log file:
% cat /var/log/mylog.log
2019-10-16 10:46:49 this is a test of the emergency broadcast system
2019-10-16 10:49:05 this is a test of the emergency broadcast system
2019-10-16 10:49:05 [ERROR] non zero exit status has occurred...
2019-10-16 10:49:05 [WARN] non standard config detected...
Python Example:
# function that contains logging info
def run_jamf_policy(run_list):
"""run through all jamf policies that need to be done"""
write_to_dnlog("Status: Installing software...")
number_of_policies = len(run_list)
write_to_dnlog("Command: DeterminateManual: %s" % number_of_policies)
for index, policy in enumerate(run_list, 1):
# get the vanity name from the MAIN_POLICY_DICT
name = MAIN_POLICY_DICT[policy]
write_to_dnlog("Status: Deploying %s" % name)
write_to_dnlog("Command: DeterminateManualStep:")
logging.info("starting %s policy..." % policy)
cmd = ["jamf", "policy", "-event", str(policy)]
proc = subprocess.Popen(cmd, stdout=subprocess.PIPE, stderr=subprocess.PIPE)
out, errors = proc.communicate()
if proc.returncode != 0:
error_msg = "%s code %s" % (errors.strip(), proc.returncode)
logging.error("jamf binary failed to install %s, %s", policy, error_msg)
write_to_dnlog("Status: %s policy failed, please see logs..." % policy)
elif proc.returncode == 0:
logging.info("jamf policy %s returned successful.." % policy)
write_to_dnlog("Status: %s was successfully installed..." % name)
write_to_dnlog("Command: DeterminateOff:")
write_to_dnlog("Command: DeterminateOffReset:")
# function to write to the DEP Notify log
def write_to_dnlog(text):
"""function to modify the DEP log and send it commands"""
depnotify = "/private/var/tmp/depnotify.log"
with open(depnotify, "a+") as log:
log.write(text + "
")
You definitely want to build logic around your logging and check exit status and capture things like stderr
