Script to Install / Update Java
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on
09-28-2018
06:26 AM
- last edited on
03-04-2025
01:57 AM
by
kh-richa_mig
Disregard... as soon as I posted this, I found a script that appears to work.
I know this has been discussed several times, but I wanted to throw my 2 cents in... I wrote a script to install and update Java. This version only does the JRE, although I'm sure you could expand it to do the JDK also.
#!/bin/sh
# Get the latest version of Java
javaver=`curl -s http://javadl-esd-secure.oracle.com/update/baseline.version | grep 1.8.0_`
minorver=`echo $javaver | grep -o '...$'`
# Get build number (counting down from 20), since I can't find anywhere that Oracle publishes this in an easily-findable place
b=20
while [ $b -gt 1 ] ; do
echo "Build number $b"
filename="jre-8i$minorver-macosx-x64.dmg"
curl -j -k -L -H "Cookie: oraclelicense=accept-securebackup-cookie" http://download.oracle.com/otn-pub/java/jdk/8u$minorver-b$b/96a7b8442fe848ef90c96a2fad6ed6d1/jre-8u$minorver-macosx-x64.dmg > $filename
# Check the file size of the download
minsize="500000"
filesize=$(wc -c <"$filename")
# If the file is larger than 500kb, assume it's the real dmg
if [ $filesize -ge $minsize ]; then
echo "Build number: $b succeeded"
break
fi
b=`expr $b - 1`
done
# Attach the DMG
hdiutil attach jre-8i$minorver-macosx-x64.dmg
# Install
sudo installer -pkg /Volumes/Java 8 Update $minorver/Java 8 Update $minorver.app/Contents/Resources/JavaAppletPlugin.pkg -target /
# Remove DMG
rm jre-8i$minorver-macosx-x64.dmg
# Unmount DMG
diskutil umount /Volumes/Java 8 Update $minorver
I'm positive it can be dramatically improved, but even though there are dozens of threads about it, I couldn't find a script that worked for me. So far, it's worked on a couple of my test systems, both without Java installed at all, and with an older version (1.8.0_131). It successfully installed/upgraded both.
- Labels:
-
Scripts
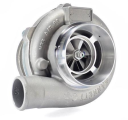
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-21-2018 06:04 AM
I tried testing your script out. I have a solid JDK solution but I'm looking for a JRE option. It errors out with the following after the download. I do see it writing this file to the directory the script is executed in jre-8i191-macosx-x64.dmg .
hdiutil: attach failed - image not recognized
installer: Error - the package path specified was invalid: '/Volumes/Java 8 Update 191/Java 8 Update 191.app/Contents/Resources/JavaAppletPlugin.pkg'.
Unmount failed for /Volumes/Java 8 Update 191
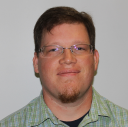
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 02-15-2019 10:10 AM
For anybody that cares, I put together this script that checks the latest version of Java against the currently installed one, if there is. If they don't match, it downloads the latest version of Java and installs it.
It's a mash up of these other scripts plus the ideas that @omatsei had in the OP:
https://www.jamf.com/jamf-nation/third-party-products/files/764/firefox-install-update
https://lew.im/2017/03/auto-update-chrome/
#!/bin/bash
#############################################################################################################
## Information ##
#############################################################################################################
## Created by Clay Nelson on 2/15/19 ##
## Sources: ##
## Shannon Johnson - https://www.jamf.com/jamf-nation/discussions/29555/script-to-install-update-java ##
## Lewis Lebentz - https://lew.im/2017/03/auto-update-chrome/ ##
## Joe Farage - https://www.jamf.com/jamf-nation/third-party-products/files/764/firefox-install-update ##
#############################################################################################################
# Variables
dmgfile="javaUpdate.dmg"
logfile="/Library/Logs/JavaInstallScript.log"
# Get the latest version number of Java available.
latestver=`/usr/bin/curl -s http://javadl-esd-secure.oracle.com/update/baseline.version | grep "1.8.0"`
latestJavaVer=`echo "$latestver" | awk -F "." '{print $2}'`
latestMinorVer=`echo "$latestver" | awk -F "_" '{print $2}'`
/bin/echo "Latest Version is: $latestver"
/bin/echo "`date`: Latest Version is: $latestver" >> ${logfile}
# Get the version number of the currently-installed Java, if any.
if [ -e "/Library/Internet Plug-Ins/JavaAppletPlugin.plugin" ]; then
currentInstalledVer=`/usr/bin/defaults read "/Library/Internet Plug-Ins/JavaAppletPlugin.plugin/Contents/Home/lib/deploy/JavaControlPanel.prefPane/Contents/Info.plist" CFBundleShortVersionString`
/bin/echo "Current installed version is: $currentInstalledVer"
/bin/echo "`date`: Current installed version is: $currentInstalledVer" >> ${logfile}
currentJavaVer=`echo "$currentInstalledVer" | awk -F "." '{print $2}'`
currentMinorVer=`echo "$currentInstalledVer" | awk -F "_" '{print $2}'`
if [ ${latestver} = ${currentInstalledVer} ]; then
/bin/echo "Java is already up to date, running Java ${currentJavaVer} Update ${currentMinorVer}."
/bin/echo "`date`: Java is already up to date, running Java ${currentJavaVer} Update ${currentMinorVer}." >> ${logfile}
/bin/echo "--" >> ${logfile}
exit 0
fi
else
currentInstalledVer="none"
/bin/echo "Java is not installed"
/bin/echo "`date`: Java is not installed" >> ${logfile}
fi
# Getting the latest version of the url for Java download
latestSite=`/usr/bin/curl -s https://www.oracle.com/technetwork/java/javase/downloads/index.html | grep "/technetwork/java/javase/downloads/jre8-downloads-" | awk -F '"' '{print $4}'`
url=`/usr/bin/curl -s https://www.oracle.com${latestSite} | grep "macosx-x64.dmg" | grep "${latestJavaVer}u${latestMinorVer}" | awk -F, '{print $3}' | awk -F '"' '{print $4}'`
# Downloading and Installing
/bin/echo "Latest version of the URL is: $url"
/bin/echo "`date`: Download URL: $url" >> ${logfile}
/bin/echo "Downloading latest version..."
/bin/echo "`date`: Downloading latest version." >> ${logfile}
/usr/bin/curl -s -j -k -L -H "Cookie: oraclelicense=accept-securebackup-cookie" ${url} > /tmp/${dmgfile}
/bin/echo "Mounting installer disk image..."
/bin/echo "`date`: Mounting installer disk image." >> ${logfile}
/usr/bin/hdiutil attach /tmp/${dmgfile} -nobrowse -quiet
/bin/echo "Installing..."
/bin/echo "`date`: Installing..." >> ${logfile}
sudo installer -pkg /Volumes/Java ${latestJavaVer} Update ${latestMinorVer}/Java ${latestJavaVer} Update ${latestMinorVer}.app/Contents/Resources/JavaAppletPlugin.pkg -target /
/bin/sleep 10
/bin/echo "Unmounting installer disk image..."
/bin/echo "`date`: Unmounting installer disk image." >> ${logfile}
/usr/bin/hdiutil detach $(/bin/df | /usr/bin/grep "Java" | awk '{print $1}') -quiet
/bin/sleep 10
/bin/echo "Deleting disk image..."
/bin/echo "`date`: Deleting disk image." >> ${logfile}
/bin/rm /tmp/${dmgfile}
# Double check to see if the new version got updated
newlyInstalledVer=`/usr/bin/defaults read "/Library/Internet Plug-Ins/JavaAppletPlugin.plugin/Contents/Home/lib/deploy/JavaControlPanel.prefPane/Contents/Info.plist" CFBundleShortVersionString`
newlyInstalledJavaVer=`/bin/echo "${newlyInstalledVer}" | awk -F "." '{print $2}'`
newlyInstalledMinorVer=`/bin/echo "${newlyInstalledVer}" | awk -F "_" '{print $2}'`
if [ "${latestver}" = "${newlyInstalledVer}" ]; then
/bin/echo "SUCCESS: Java has been updated to: Java ${newlyInstalledJavaVer} Update ${newlyInstalledMinorVer}."
/bin/echo "`date`: SUCCESS: Java has been updated to: Java ${newlyInstalledJavaVer} Update ${newlyInstalledMinorVer}." >> ${logfile}
/bin/echo "--" >> ${logfile}
else
/bin/echo "ERROR: Java update unsuccessful, version remains at: Java ${currentJavaVer} Update ${currentMinorVer}."
/bin/echo "`date`: ERROR: Java update unsuccessful, version remains at: Java ${currentJavaVer} Update ${currentMinorVer}." >> ${logfile}
/bin/echo "--" >> ${logfile}
exit 1
fi
exit 0
I have a hard time with Patch Management Policies because I manage labs and there is no way to set a maintenance window like in normal policies. I can't have these things go off in the middle of class.
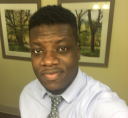
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 03-07-2019 01:32 PM
@cnelson Good work! Worked like a charm.
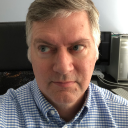
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 03-26-2019 10:42 AM
Thanks. Java JRE updating has always been a pain and these scripts have greatly reduced that pain.
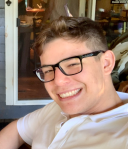
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 05-01-2019 12:33 PM
@cnelson Is this script still working in your environment? Mine is erroring.
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 05-01-2019 12:37 PM
@jared_f Just speculating, but with the changes in license requirements for JRE 8.x, the script may need to be revised. (JRE no longer authorized for commercial use without a license). I'm unable to manually download it without logging into an Oracle account first.
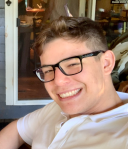
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 05-01-2019 04:31 PM
That is most likely the issue. Ugh... Java...
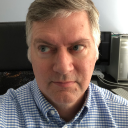
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 05-02-2019 09:46 AM
Just confirming that after I got things working using this script in late February it is now broken. I've confirmed—as best I can—that it is a login issue. Without being logged in you cannot download the packages/installers so the scripted system no longer works. We are experimenting with changes and I'm reading the fine print from Oracle to see how we might move forward (although there's nothing in their license that seems to prevent distributing Java SE yourself as long as you don't modify it).
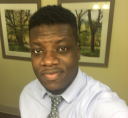
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 05-20-2019 11:34 AM
Getting the below error when running this policy. Anything changed?
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 07-16-2019 11:51 AM
Nice work! Also for what it is worth I cleaned up some syntax in the sctipt, tested on my 10.14.6 (beta) system and it ran very well.
This is the script output from my system:
I wanted to post the script but having a hard time, sorry.
- dmgfile=javaUpdate.dmg
- logfile=/Library/Logs/JavaInstallScript.log + /usr/bin/curl -s http://javadl-esd-secure.oracle.com/update/baseline.version + grep 1.8.0
- latestver=1.8.0_221 + echo 1.8.0_221 + awk -F . '{print $2}'
- latestJavaVer=8 + echo 1.8.0_221 + awk -F _ '{print $2}'
- latestMinorVer=221
- /bin/echo 'Latest Version is: 1.8.0_221' Latest Version is: 1.8.0_221
- /bin/echo 'date: Latest Version is: 1.8.0_221'
- '[' -e '/Library/Internet Plug-Ins/JavaAppletPlugin.plugin' ']' ++ /usr/bin/defaults read '/Library/Internet Plug-Ins/JavaAppletPlugin.plugin/Contents/Home/lib/deploy/JavaControlPanel.prefPane/Contents/Info.plist' CFBundleShortVersionString
- currentInstalledVer=1.8.0_221
- /bin/echo 'Current installed version is: 1.8.0_221' Current installed version is: 1.8.0_221
- /bin/echo 'date: Current installed version is: 1.8.0_221' + echo 1.8.0_221 + awk -F . '{print $2}'
- currentJavaVer=8 + echo 1.8.0_221 + awk -F _ '{print $2}'
- currentMinorVer=221
- '[' 1.8.0_221 = 1.8.0_221 ']'
- /bin/echo 'Java is already up to date, running Java 8 Update 221.' Java is already up to date, running Java 8 Update 221.
- /bin/echo 'date: Java is already up to date, running Java 8 Update 221.'
- /bin/echo --
- exit 0
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 07-16-2019 12:28 PM
Your right there is some login to the site that prevents the package from being downloaded.
I tested part of the url and latest site parts of the scipt there is a login that is needed.
Oh well.
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 07-16-2019 12:40 PM
^^ Java 8 is no longer free to use. Hasn't been for some time. Covered earlier in thread.
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 07-22-2019 10:28 AM
dvasquez, can you copy the cleaned as plain txt up script into the response window. I'm getting the same error messages as the others but curious to see how your script was written.
Does anyone else have a recommended method for pushing out/updating Java?
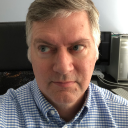
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 08-05-2019 08:26 AM
We evaluated our needs for Java JRE and found that it is no longer required for anything that our users do (at least not that is required for their work). Java is only required for either use by IT staff or backend servers so our solution was to completely uninstall the Java JRE from all user systems and we are able to easily manually manage the remaining systems. For us this put a nail in the coffin for Java JRE on the user side and it is also a big security win as it reduces vectors for exploits/malware/viruses.
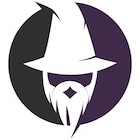
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 05-07-2020 10:21 AM
This command will fetch the latest download link:
/usr/bin/curl -s https://www.oracle.com$(/usr/bin/curl -s https://www.oracle.com/java/technologies/javase-downloads.html | /usr/bin/grep 'javase-jre8-downloads' | /usr/bin/cut -d' -f2) | /usr/bin/grep 'macosx-x64.dmg' | /usr/bin/cut -d' -f6 | /usr/bin/sed 's/otn/otn-pub/'
Output: //download.oracle.com/otn-pub/java/jdk/8u251-b08/3d5a2bb8f8d4428bbe94aed7ec7ae784/jre-8u251-macosx-x64.dmg
Then this command will download it:/usr/bin/curl -s -OjkL -H 'Cookie: oraclelicense=accept-securebackup-cookie' https://download.oracle.com/otn-pub/java/jdk/8u251-b08/3d5a2bb8f8d4428bbe94aed7ec7ae784/jre-8u251-macosx-x64.dmg
No login required for now...
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 05-29-2020 08:13 AM
@mberger
Sorry for the late reply Let me go back and find it. If not needed let me know, I took a gander and tested @atlas and that worked well.
Thanks.
D
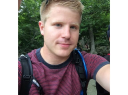
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-13-2020 10:11 AM
@atlas I haven't been able to get this to work on Catalina. An issue with the curl version maybe. Has anyone had success?
