Create arbitrary files of a specified size
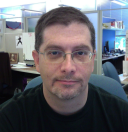
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-01-2023 05:54 AM
This is an odd one but I figured I'd share the fruits of my labors. I was faced with a testing situation where I needed to fill up hard drives to reduce the amount of free space. I had to test pre-install conditions where there might not be enough free space on a hard drive - I can't test that unless a drive is almost full. I couldn't figure out how to quickly fill up a drive so I wrote a script to do it. As written it can create files of 1GB, 5GB, 10GB, 50GB, and 100GB, but you can modify this to meet your specific needs. In the AppleScript selector window, I have added the approximate time it takes to create each size (CodeRunner is great for this). Obviously that will depend on the hardware it's run on. The script will create files on the desktop of the currently logged in user with the size in the filename and append a number to the end if there are duplicates. These generated files could also be used for the purpose of testing upload/download times to and from servers.
#!/bin/bash
CurrentUser=`ls -l /dev/console | cut -d " " -f4`
cd /Users/$CurrentUser/Desktop/
# Function to check for existing file and modify the filename if needed
check_existing_file() {
local file="$1"
local count=1
local original_file="$file"
while [ -e "$file" ]; do
filename="${original_file%.*}" # Get filename without extension
extension="${original_file##*.}" # Get file extension (if any)
if [ -z "$extension" ]; then
file="${filename}_${count}"
else
file="${filename}_${count}.${extension}"
fi
((count++))
done
echo "$file"
}
# Prompt the user for file size selection using AppleScript
file_size=$(osascript -e 'choose from list {"1GB - 0:05", "5GB - 0:20", "10GB - 0:45", "50GB - 3:30", "100GB - 7:15"} with prompt "Select file size"')
case $file_size in
"1GB - 0:05")
size="1g"
name="1GB file.txt"
;;
"5GB - 0:20")
size="5g"
name="5GB file.txt"
;;
"10GB - 0:45")
size="10g"
name="10GB file.txt"
;;
"50GB - 3:30")
size="50g"
name="50GB file.txt"
;;
"100GB - 7:15")
size="100g"
name="100GB file.txt"
;;
*)
echo "Invalid selection"
exit 1
;;
esac
# Define the output file name
output_file=$name
# Check for existing file and modify filename if needed
output_file=$(check_existing_file "$output_file")
# Create the chosen size file
mkfile -v "$size" /Users/$CurrentUser/Desktop/"$output_file"
echo "File created: $output_file"
