Extension Attribute to report install JDK versions in OS X
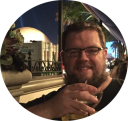
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 05-22-2013 12:03 PM
So I ran into an interesting issue on my system with Java. When the 1.0.7_21 JDK was installed and I attempted to revert the Internet Plugin to leverage Apples 1.6.x CrashPlan and Self Service would crash. That issue seems a bit more in depth then I really want to mess with. So I made this Extension Attribute to check if the JDK was installed and what version. That way I can avoid deploying to these systems which would really put a damper on my day.
Enjoy it! Improve it! Use it!
#!/bin/sh
# exta_jdkCheck.sh
#
#
# Created by Andrew Seago on 05/22/13.
# set -x # DEBUG. Display commands and their arguments as they are executed
# set -v # VERBOSE. Display shell input lines as they are read.
# set -n # EVALUATE. Check syntax of the script but dont execute
## Variables
####################################################################################################
jdk6=`ls /Library/Java/JavaVirtualMachines | grep "jdk" | grep "1.6" | sed 's/.jdk//' | sed 's/jdk//'`
jdk7=`ls /Library/Java/JavaVirtualMachines | grep "jdk" | grep "1.7" | sed 's/.jdk//' | sed 's/jdk//'`
## Script
####################################################################################################
if [ "$jdk6" != "" ] || [ "$jdk7" != "" ]; then
echo "<result>$jdk6 $jdk7</result>"
else
echo "<result>NoJDK</result>"
fi
exit 0
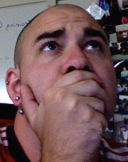
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 08-04-2015 08:01 AM
@andrewseago didn't notice you had this up here and recently put this together with @elliotjordan on slack. He made it into the sweet one-liner. Thought I'd share.
Dan
https://gist.github.com/dderusha/bd06694dbec35470e2db
###############
### Intention is to figure out what JDK the user has installed.
### our current Ext atty reports the JRE, we need to know they have the JDK
### thanks to elliotjordon
### -00 is just a number to say that JDK is not installed. makes for better smartgroup searches
###############
if JDKver=$(ls "/Library/Java/JavaVirtualMachines" | awk -F "_|.jdk" '/.jdk/{print $2}' | tail -1)
then
echo "<result>$JDKver</result>"
# else
# echo "<result>-00</result>"
fi
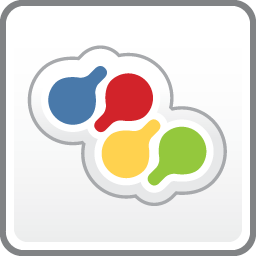
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 08-04-2015 12:39 PM
Thanks for @dderusha and @elliotjordan's example above, I've also written a couple of JDK-related extension attributes.
This one should tell you if a machine's JDK vendor was Oracle or Apple:
#!/bin/bash
osvers=$(sw_vers -productVersion | awk -F. '{print $2}')
if [[ ${osvers} -lt 6 ]]; then
echo "<result>Unaffected</result>"
fi
if [[ ${osvers} -ge 6 ]]; then
jdk_installed=`/usr/libexec/java_home 2>/dev/null`
if [[ "$jdk_installed" == "" ]]; then
result="Java JDK Not Installed"
fi
if [[ "$jdk_installed" != "" ]]; then
javaJDKVendor=`defaults read $(/usr/libexec/java_home | sed 's/Home//g' | sed s'/.$//')/Info CFBundleIdentifier | grep -o "apple|oracle"`
if [[ "$javaJDKVendor" = "oracle" ]]; then
result="Oracle"
elif [[ "$javaJDKVendor" = "apple" ]]; then
result="Apple"
fi
fi
echo "<result>$result</result>"
fi
This one should tell you if you have Oracle's JDK installed or not. If you do, it'll give you the version number. If you don't, it'll tell you Oracle's JDK is not installed:
#!/bin/bash
osvers=$(sw_vers -productVersion | awk -F. '{print $2}')
if [[ ${osvers} -lt 6 ]]; then
echo "<result>Unaffected</result>"
fi
if [[ ${osvers} -ge 6 ]]; then
jdk_installed=`/usr/libexec/java_home 2>/dev/null`
if [[ "$jdk_installed" == "" ]]; then
result="Oracle Java JDK Not Installed"
fi
if [[ "$jdk_installed" != "" ]]; then
javaJDKVendor=`defaults read $(/usr/libexec/java_home | sed 's/Home//g' | sed s'/.$//')/Info CFBundleIdentifier | grep -o "oracle"`
if [[ "$javaJDKVendor" = "oracle" ]]; then
javaJDKVersion=`defaults read $(/usr/libexec/java_home | sed 's/Home//g' | sed s'/.$//')/Info CFBundleVersion`
result="$javaJDKVersion"
else
result="Oracle Java JDK Not Installed"
fi
fi
echo "<result>$result</result>"
fi
