Need help creating script for changing computer name before AD binding
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-12-2013 08:52 AM
Hello! I'm trying to create a script that will allow me to change the computer name to "OX" followed by the serial number of the machine.
I know that I can user the system_profiler | grep "r (system)" to bring up the serial number, but how would I need to append "OX" before the serial number?
Any help would be appreciated! Thanks!
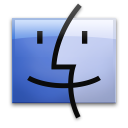
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-12-2013 09:21 AM
You would use either a variable for "OX", or simply create a new Computer Name variable with OX + the variable for the Serial #.
So here's a better way to grab the serial number (because system_profiler is a bit slow):
Serial=$(ioreg -rd1 -c IOPlatformExpertDevice | awk -F'["|"]' '/IOPlatformSerialNumber/{print $4}')
Putting "OX" together with the above should be pretty simple (create a "MacName" variable for example)-
MacName="OX$Serial"
If you wanted something between the two, like a dash, then just add that as well, like this-
MacName="OX-$Serial"
So now use that in your rename script. Something like (just an example)-
scutil --set ComputerName "$MacName"
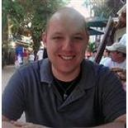
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-12-2013 09:22 AM
If you use pre-stage imaging in the JSS you can setup OX- to be the prefix to the serial number as the computer name. Very easy to do and requires no scripting. If you need a script to do this and would prefer not to use in the pre-stage feature I can give you an example of what we have done when I have some available time later today.
*EDIT* Looks like mm2270 beat me to it. :)
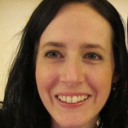
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-12-2013 09:53 AM
You might also want to think about setting these too:
scutil --set LocalHostName
scutil --set HostName

- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-12-2013 10:03 AM
This is what we use:
#!/bin/sh
# Rename Computer.sh
#
# Renames the computer to the $4, as entered by the script parameter
# The computer will be unbound from Active Directory, renamed, then rebound.
# Created by Barnes, Walter on 11/6/12.
#
# Set computername to the parameter entered by the script
computername=$4
# if there is no name set exit with an error
if [ "$computername" == "" ]; then
echo "Error: No computername is specified. Please specify a Computer Name"
exit 1
fi
# AD user information for removing the machine from Active Directory
username=""
password=""
# Unbind from Active Directory
echo "Unbinding the computer from Active Directory..."
/usr/sbin/dsconfigad -r -u "$username" -p "$password"
/usr/bin/killall DirectoryService
# Rename the computer
echo "Renaming computer to $4"
/usr/sbin/scutil --set ComputerName "$4"
/usr/sbin/scutil --set LocalHostName "$4"
/usr/sbin/scutil --set HostName "$4"
# Update Inventory in JSS
echo "Updating inventory..."
/usr/sbin/jamf recon
# Rebind to AD by triggering the AD Bind Policy
#echo "Rebinding the computer to Active Directory..."
jamf policy -trigger bind
exit 0
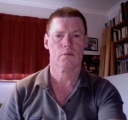
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-12-2013 03:02 PM
Is there a way to grab the last 6 characters of the serial number and enter it into the naming script?
Wanting to use a script with only the last 6 characters as part of the computer name.
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-12-2013 03:56 PM
Here you go:
Serial=$(ioreg -rd1 -c IOPlatformExpertDevice | awk -F'["|"]' '/IOPlatformSerialNumber/{print $4}') echo ${Serial:(-6)}
or even make it a variable to use in your naming:
last6=echo ${Serial:(-6)}
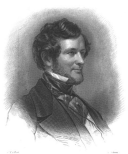
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-12-2013 05:13 PM
Be aware... scutil can behave strangely and slowly from time to time for reasons unknown (to us). You may not always get what you expect WHEN you expect it.
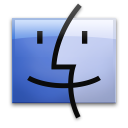
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-13-2013 06:59 AM
@dmw3][/url][/url][/url][/url - You could also do this to get the last 6 characters-
Serial=$(ioreg -rd1 -c IOPlatformExpertDevice | awk -F'["|"]' '/IOPlatformSerialNumber/{print $4}' | cut -c 6-)
@lisacherie][/url][/url][/url][/url - good point! You definitely would want to set the other 2 names to the new one as well.
@yellow][/url][/url][/url][/url - if you get dodgy results with scutil, there are other options. For example, with systemsetup:
/usr/sbin/systemsetup -setcomputername "$MacName"
or with the jamf binary:
jamf setComputerName -name" $MacName"
In fact, you could actually accomplish the entire renaming directly with the jamf binary.
sudo jamf setComputerName -useSerialNumber -prefix "OX"
But that would give you the full Serial Number, not just the last 6 characters, or any other variation.
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-13-2013 07:14 AM
Thanks for the responses guys! This definitely helped me out and gave me some ideas!
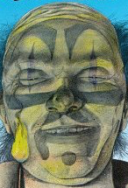
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 08-16-2016 03:48 PM
Note:
/usr/sbin/networksetup
&
/usr/sbin/systemsetup
will error if your computer name is > 31 characters.
For example...
/usr/sbin/networksetup -setcomputername "01234567890123456789012345678901"
01234567890123456789012345678901 is NOT a valid computer name.
** Error: The parameters were not valid.
/usr/sbin/systemsetup -setcomputername "01234567890123456789012345678901"
2016-08-16 16:42:31.348 systemsetup[3368:55826] ### authenticateUsingAuthorizationSync error:Error Domain=com.apple.systemadministration.authorization Code=-60007 "(null)"
setcomputername: 01234567890123456789012345678901
See blog post for example...
OS X Bonjour Naming Guidelines
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 10-26-2017 09:17 AM
I do like this script... Is there a way to have it set so that there's a dialog box to 1) Enter the computer name (through policy) and 2) Request credentials to do the unbinding and rebinding?
Unfortunately, I havn't done much scripting...
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 10-30-2017 01:04 PM
Anybody?
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 10-30-2017 01:58 PM
@boanes Others may have different answers, but I've always found that interacting with a. dialogue box was a limitation of JAMF. It had to get called by something else. Many would suggest cocoa dialog, but that's not been updated in a. long time, and for our environment, any tool/app we'd install as a helper would have to go through a certification process. I never found it worth it. When I've needed it, I've leveraged AppleScript to do most of that heavy lifting, and then just export that out as an app. I did have an AppleScript that I'd written LONG ago (like 6 years) but through a few computer changes, when I was first messing with Apple Script. It's VERY rudimentary, and is totally different than what I'd do today, but feel free to leverage it.
In this case, it was for a bunch of minis, which we designated with the prefix MKMM and the last string of their serial.
#Get user ID
set getusername to the text returned of (display dialog "Enter your Admin user id here..." default answer "")
set userid to getusername
set userpass to the text returned of (display dialog "Enter your password.." default answer "" with hidden answer)
set adminpass to the text returned of (display dialog "Enter your local admin password.." default answer "" with hidden answer)
set theserial to (do shell script "system_profiler |grep 'r (system)' | awk '{print $4}'")
set count1 to count theserial
if count1 = 11 then set cutserial to (do shell script "system_profiler |grep 'r (system)' | awk '{print $4}' |cut -c 4-11")
if count1 = 12 then set cutserial to (do shell script "system_profiler |grep 'r (system)' | awk '{print $4}' |cut -c 5-12")
if count1 = 13 then set cutserial to (do shell script "system_profiler |grep 'r (system)' | awk '{print $4}' |cut -c 6-13")
if count1 = 14 then set cutserial to (do shell script "system_profiler |grep 'r (system)' | awk '{print $4}' |cut -c 7-14")
set computername to "MKMM" & cutserial
#display dialog computername
#display dialog cutserial
#do shell script "scutil --set ComputerName " & computername with administrator privileges
#do shell script "scutil --set LocalHostName " & computername with administrator privileges
do shell script "diskutil rename disk0s2 " & computername
tell application "Terminal"
tell window 1
activate
tell application "System Events"
set terminal to application process "Terminal"
set frontmost of terminal to true
tell application "System Events"
delay "3"
keystroke "sudo -i"
key code 36
delay "1"
keystroke adminpass
key code 36
delay "1"
keystroke "scutil --set ComputerName " & computername
key code 36
delay "2"
keystroke "scutil --set LocalHostName " & computername
key code 36
delay "2"
keystroke userpass
key code 36
delay "75"
tell application "Terminal"
quit
end tell
end tell
end tell
end tell
end tell
wow... looking over this I'm almost too embarrassed to share... almost. good luck. if I have some time upcoming, maybe I'll clean this up.
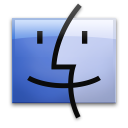
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 10-30-2017 02:26 PM
@boanes You're looking for a way to input a name manually and then name the computer to that?
If so, Applescript calls can be used there, but the trouble you run into often these days is the OS doesn't like popping up interactive user level dialogs from scripts run under a root context.
But so you know, something like the following would be used to ask for user input, would loop if no text is entered (so it forces something to be entered to dismiss the dialog)
This is a basic example with not much in the way of error checking, checking character length, etc, all of which you'd probably want to do to ensure the name entered won't cause problems when used for the computer name.
#!/bin/bash
function getResponse ()
{
response=$(/usr/bin/osascript << EOD
tell application "System Events"
set response to text returned of (display dialog "Enter a computer name" default answer "" buttons {"Enter"} default button 1)
end tell
EOD)
if [[ "$response" == "" ]]; then
getResponse
fi
}
getResponse
## Put computer naming commands here
Again though, YMMV regarding whether this will actually pop up a dialog when it's run from a Jamf Pro policy, or if it will register a failure due to not being allowed user interaction. I have posted elsewhere on here some examples of how to get Applescripts like this to run as the user (to get input) and then use the response in the remainder of the script. So there are ways to do it.
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 10-30-2017 02:49 PM
We used this script to rename, unbind, then rebind to AD. (AD will not automatically update the records when you change the name)
#!/bin/sh
# HARDCODED VALUES ARE SET HERE
Pass=""
# CHECK TO SEE IF VALUES WERE PASSED FOR $4, AND IF SO, ASSIGN THEM
if [ "$4" != "" ] && [ "$Pass" == "" ]; then
Pass=$4
fi
# Check to make sure Pass variable was passed down from Casper
if [ "$Pass" == "" ]; then
echo "Error: The parameter 'Pass' is blank. Please specify a value."
exit 1
fi
# Set Variables
CompName=$(dsconfigad -show | awk '/Computer Account/{print $NF}' | sed 's/$$//')
# Get current OU of Computer Object
OU="OU=General Business Computers,OU=####,DC=#####,DC=#####,DC=####"
echo "$OU"
# get machine serial number
MAC_SERIAL_NUMBER=`ioreg -l | grep IOPlatformSerialNumber|awk '{print $4}' | cut -d " -f 2`
echo MAC_SERIAL_NUMBER $MAC_SERIAL_NUMBER
serial=$MAC_SERIAL_NUMBER
# set name to machine serial number
scutil --set ComputerName $serial
scutil --set HostName $serial
scutil --set LocalHostName $serial
sleep 2
## Begin rebinding process
#Basic variables
computerid=`scutil --get LocalHostName`
domain=c##
udn=#
#Advanced variables
alldomains="disable"
localhome="enable"
protocol="smb"
mobile="enable"
mobileconfirm="disable"
user_shell="/bin/bash"
admingroups="CorpHelpdesk Operator"
namespace="domain"
packetsign="allow"
packetencrypt="allow"
useuncpath="disable"
passinterval="30"
# Bind to AD
dsconfigad -add $domain -alldomains $alldomains -username $udn -password $Pass -computer $computerid -ou "$OU" -force -packetencrypt $packetencrypt
sleep 1
echo "Rebinding to AD and setting advanced options"
#set advanced options
dsconfigad -localhome $localhome
sleep 1
dsconfigad -groups "$admingroups"
sleep 1
dsconfigad -mobile $mobile
sleep 1
dsconfigad -mobileconfirm $mobileconfirm
sleep 1
dsconfigad -alldomains $alldomains
sleep 1
dsconfigad -useuncpath "$useuncpath"
sleep 1
dsconfigad -protocol $protocol
sleep 1
dsconfigad -shell $user_shell
sleep 1
dsconfigad -passinterval $passinterval
sleep 1
#dsconfigad adds "All Domains"
# Set the search paths to "custom"
dscl /Search -create / SearchPolicy CSPSearchPath
dscl /Search/Contacts -create / SearchPolicy CSPSearchPath
sleep 1
# Add the "corpXXXXXX" search paths
dscl /Search -append / CSPSearchPath "/Active Directory/CORP/#####"
dscl /Search/Contacts -append / CSPSearchPath "/Active Directory/CORP/#####"
sleep 1
# Restart opendirectoryd
killall opendirectoryd
sleep 5
exit 0
