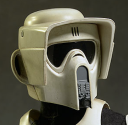
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-20-2015 10:05 AM
Hey guys,
I am writing a script for use as an extension attribute which will check for the existence of Sublime Text.app, check that the console user isn't a local admin or root, and then go an check both Sublime Text 2 and also Sublime Text 3 and report back on whether either or is licensed. The script seems to be working for the most part, but I am getting a failure on the defaults read even though it should not be running when Sublime isn't installed (the script should just return "NA" to the JSS and exit). Any ideas?
#!/bin/sh
thisUser=`stat -f '%u %Su' /dev/console | awk '{ print $2 }'`
STV=`defaults read /Applications/Sublime Text.app/Contents/Info.plist CFBundleIdentifier`
if test -e /Applications/Sublime Text.app; then
if [ $thisUser = "root" ]; then
echo "User is root, bailing."
exit
elif [[ $thisUser == *admin* ]]; then
echo "User is the local admin, bailing."
exit
else
echo "User is not root or the local admin, checking Sublime Text."
if [ "$STV" == "com.sublimetext.2" ]; then
if test -e /Users/"$thisUser"/Library/Application Support/Sublime Text 2/Local/License.sublime_license; then
echo "<result>ST2 Licensed</result>"
else
echo "<result>ST2 Unlicensed</result>"
fi
fi
if [ "$STV" == "com.sublimetext.3" ]; then
if test -e /Users/"$thisUser"/Library/Application Support/Sublime Text 3/Local/License.sublime_license; then
echo "<result>ST3 Licensed</result>"
else
echo "<result>ST3 Unlicensed</result>"
fi
fi
fi
exit
else
echo "<result>NA</result>"
exit
fi
Heres what I get:
<result>NA</result>
2015-11-20 13:07:39.667 defaults[42900:4252084]
The domain/default pair of (/Applications/Sublime Text.app/Contents/Info.plist, CFBundleIdentifier) does not exist
Solved! Go to Solution.
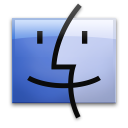
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-20-2015 10:20 AM
You're seeing the error because this line:
STV=`defaults read /Applications/Sublime Text.app/Contents/Info.plist CFBundleIdentifier`
is being evaluated by the script, meaning run by it, prior to the actual check to see if Sublime Text is installed. You can try either moving the whole defaults line into the section after the echo "User is not root or the local admin, checking Sublime Text." section, or, put it into a function that gets called by the script.
BTW, you can shorten the thisUser command to only stat, no need to pass through awk, like this:
stat -f%Su /dev/console
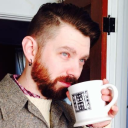
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-20-2015 10:25 AM
Maybe replace
#!/bin/sh
STV=`defaults read /Applications/Sublime Text.app/Contents/Info.plist CFBundleIdentifier`
if test -e /Applications/Sublime Text.app; then
with
#!/bin/sh
if [ -f /Applications/Sublime Text.app/Contents/Info.plist ] ; then
STV=$( defaults read /Applications/Sublime Text.app/Contents/Info.plist CFBundleIdentifier )
I think the logic there is right...
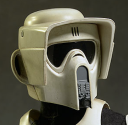
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-20-2015 10:06 AM
Gawd that formatting got butchered! Let me try to fix it.
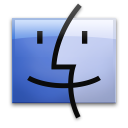
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-20-2015 10:20 AM
You're seeing the error because this line:
STV=`defaults read /Applications/Sublime Text.app/Contents/Info.plist CFBundleIdentifier`
is being evaluated by the script, meaning run by it, prior to the actual check to see if Sublime Text is installed. You can try either moving the whole defaults line into the section after the echo "User is not root or the local admin, checking Sublime Text." section, or, put it into a function that gets called by the script.
BTW, you can shorten the thisUser command to only stat, no need to pass through awk, like this:
stat -f%Su /dev/console
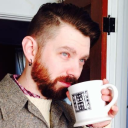
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-20-2015 10:25 AM
Maybe replace
#!/bin/sh
STV=`defaults read /Applications/Sublime Text.app/Contents/Info.plist CFBundleIdentifier`
if test -e /Applications/Sublime Text.app; then
with
#!/bin/sh
if [ -f /Applications/Sublime Text.app/Contents/Info.plist ] ; then
STV=$( defaults read /Applications/Sublime Text.app/Contents/Info.plist CFBundleIdentifier )
I think the logic there is right...
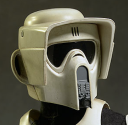
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-20-2015 10:25 AM
You da man Mike! Thanks that did the trick!
I do have to rework the test for the .app though, because Sublime Text 2 and 3 have different app names: Sublime Text 2.app and Sublime Text.app (for 3).
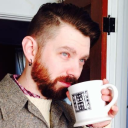
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-20-2015 10:35 AM
wildcard?
#!/bin/sh
if [ -f /Applications/Sublime Text.app/Contents/Info.plist ] ; then
STV=$( defaults read /Applications/Sublime Text*.app/Contents/Info.plist CFBundleIdentifier )
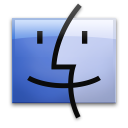
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-20-2015 11:00 AM
Yes, a wildcard should work for that, but you'll need to test it. There's also mdfind
, which can help you locate the path and full name of the Sublime application installed.
mdfind -onlyin /Applications/ 'kMDItemFSName == "Sublime Text*.app"'
That would return something like the following (for Sublime Text 2 as an example)
/Applications/Sublime Text 2.app
You can also try using the kMDItemDisplayName flag with mdfind. Not sure how it actually shows up, but the display name may just be "Sublime Text" for both versions.
mdfind -onlyin /Applications/ 'kMDItemDisplayName == "Sublime Text"'
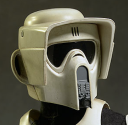
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-20-2015 11:21 AM
Im wondering if this wouldn't be better accomplished with two extension attributes: one for Sublime Text 3 and one for Sublime Text 2, as a user might have either or both...
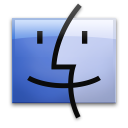
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-20-2015 11:37 AM
If there's a possibility of both being installed at the same time, then yes, it would complicate things for an EA, trying to get details on either one. Maybe they should be split up as you suggested.
OTOH, I'm pretty sure its possible to build an EA script that could report on the licensing for one or both, if both happen to be installed.
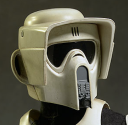
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-20-2015 11:59 AM
I decided to go with two extension attributes to make the script(s) less complicated:
Sublime Text 2:
#!/bin/bash
thisUser=`stat -f%Su /dev/console`
# Test for user not being root or local admin
if [ $thisUser = "root" ]; then
echo "User is root, bailing."
exit
elif [[ $thisUser == *admin* ]]; then
echo "User is the local admin, bailing."
exit
else
echo "User is not root or the local admin, checking Sublime Text 2."
fi
# Test for existance of Sublime Text 2
if test -e /Applications/Sublime Text 2.app; then
if test -e /Users/"$thisUser"/Library/Application Support/Sublime Text 2/Settings/License.sublime_license; then
echo "<result>Licensed</result>"
else
echo "<result>Unlicensed</result>"
fi
else echo "<result>NA</result>"
exit
fi
Sublime Text 3:
#!/bin/bash
thisUser=`stat -f%Su /dev/console`
# Test for user not being root or local admin
if [ $thisUser = "root" ]; then
echo "User is root, bailing."
exit
elif [[ $thisUser == *admin* ]]; then
echo "User is the local admin, bailing."
exit
else
echo "User is not root or the local admin, checking Sublime Text 3."
fi
# Test for existance of Sublime Text 3
if test -e /Applications/Sublime Text.app; then
if test -e /Users/"$thisUser"/Library/Application Support/Sublime Text 3/Local/License.sublime_license; then
echo "<result>Licensed</result>"
else
echo "<result>Unlicensed</result>"
fi
else echo "<result>NA</result>"
exit
fi
