Trying to create a script to change computer with user's Building and Department from Jamf Pro
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 04-29-2023 02:00 PM
Hello,
I am new to Jamf Pro and scripting and would like to know if there is a way for me to auto-name the computer by Building-Department-AssetTag#, Example: NY-LAB-01 the Asset# has to start from 1 and up for each department, not sure how to accomplish this, thank you for all the help in advance.
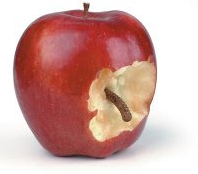
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 05-01-2023 06:03 AM
Yes, it is possible however, I suggest waiting a while before tackling this one as you will need JAMF API. I recommend keeping it simple and just using something like the Serial Number to name the device. If you needed something more human readable, set what you are wanting as the asset tag and stick it on the device somewhere.
If you want to follow the location specific naming convention your best option is to use API.
- You would need a script to gather the information you want from JAMF with API (Building, Department AssetTag)
- You will need a specific work flow to generate/count the # as JAMF has no way of knowing how many devices are already in a given lab. You would need to reference a table or database of some kind, or manually set this in JAMF somewhere.
- You will also need a work flow to set the Building, Department, and Asset Tag with API unless you want to do that manually.
- Depending on how many subnets you have, you may be able to have different scripts targeted to different subnets to accomplish some of this.
This is the script I use to set device names to the SN. This should be a good starting point for whatever you want to do.
#!/usr/bin/env bash
#*=============================================================================
#*=============================================================================
#* GLOBAL VARIABLES
#*=============================================================================
computerName=$(scutil --get ComputerName)
hostName=$(scutil --get HostName)
localHost=$(scutil --get LocalHostName)
serialNumber=$(system_profiler SPHardwareDataType | awk '/Serial/ {print $4}')
serialNumberII=$(system_profiler SPHardwareDataType | awk '/Serial/ {print $4}' | tr -d '"')
#*=============================================================================
#* FUNCTIONS
#*=============================================================================
#*=============================================================================
#* SCRIPT BODY
#*=============================================================================
userInfo
## Check & Update Computer Name
if [ "$computerName" == "$serialNumber" ]
then
echo "Computer name matches serial number, $serialNumber"
else
echo "Current Computer Name: $computerName"
echo "Computer Name does not meet standards"
echo "Changing Computer Name to match Serial Number"
scutil --set ComputerName "$serialNumber"
fi; $DIV2
## Check & Update Host Name
if [ "$hostName" == "$serialNumber" ]
then
echo "Host Name matches serial number, $serialNumber"
else
echo "Current Host Name: $hostName"
echo "Host Name does not meet standards"
echo "Changing Host Name to match Serial Number"
scutil --set HostName "$serialNumber"
fi; $DIV2
## Check & Update Local Host
if [ "$localHost" == "$serialNumber" ]
then
echo "Local Host matches serial number, $serialNumber"
else
echo "Current Local Host: $localHost"
echo "Local Host does not meet standards"
echo "Changing Local Host to match Serial Number"
scutil --set LocalHostName "$serialNumber"
fi; $DIV2
## Final Check
computerNameII=$(scutil --get ComputerName)
hostNameII=$(scutil --get HostName)
localHostII=$(scutil --get LocalHostName)
echo "Results:"; $DIV3
echo "Serial number: $serialNumber"
echo "Computer Name: $computerNameII"
echo "Host Name: $hostNameII"
echo "Local Host: $localHostII"
if [[ "$computerNameII" == "$serialNumber" ]] && [[ "$hostNameII" == "$serialNumber" ]] && [[ "$localHostII" == "$serialNumber" ]]
then
echo "Computer Name satisfies naming standards"
$DIV1; exit 0
else
echo "Computer does not meet naming standards"
echo "More troubleshooting will be necessary."
$DIV1; exit 1
fi
## Perform final Recon to update computer name on Jamf
sudo jamf recon
#*=============================================================================
#* END OF SCRIPT
#*=============================================================================
