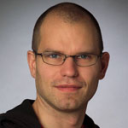
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-02-2015 02:48 AM
Hi Folks,
I would like to create have a script, that checks if 10.9, 10.10 or 10.11 is used so syadminctl or dscl is invoked for creating a user on a machine. As you can see, the script is checking the OS Version and checking the next available UserID. After that, the script jumps to the end and gives error of unexpected end of file. What is missing here?
Cheers,
Holger
#!/bin/sh
# create_user_1.2.sh
#
#
#
if [ "$(id -u)" != "0" ]; then
echo "Sorry, you are not root. Please run with sudo!"
exit 1
fi
# === For creating a User we need some input! ===
echo "Enter your desired user name: "
read USERNAME
echo "Enter a full name for this user: "
read FULLNAME
echo "Enter a password for this user: "
read -s PASSWORD
# ====
# A list of groups the user should belong to
# This makes the difference between admin and non-admin users.
echo "Is this an administrative user? (y/n)"
read GROUP_ADD
if [ "$GROUP_ADD" = n ] ; then
SECONDARY_GROUPS="staff" # for a non-admin user
elif [ "$GROUP_ADD" = y ] ; then
SECONDARY_GROUPS="admin _lpadmin _appserveradm _appserverusr" # for an admin user
else
echo "Please make a selection!"
fi
# ====
# Create a UID that is not currently in use
echo "Creating an unused UID for new user..."
# Find out the next available user ID
MAXID=$(dscl . -list /Users UniqueID | awk '{print $2}' | sort -ug | tail -1)
USERID=$((MAXID+1))
# check the OS X Version
OSXVERSION=$(sw_vers -productVersion | awk -F '.' '{print $1 "." $2}')
#if osx 10.10 then run
if [[ "$OSXVERSION" == "10.11" ]]; then
sysadminctl -addUser $USERNAME -fullName "$FULLNAME" -UID=$USERID -password $PASSWORD
else
#if osx 10.10 then run
if [[ "$OSXVERSION" == "10.10" ]]; then
sysadminctl -addUser $USERNAME -fullName "$FULLNAME" -UID=$USERID -password $PASSWORD
else
#if osx 10.9 then run
if [[ "$OSXVERSION" == "10.9" ]]; then
# Create the user account by running dscl
echo "Creating necessary files..."
dscl . -create /Users/$USERNAME
dscl . -create /Users/$USERNAME UserShell /bin/bash
dscl . -create /Users/$USERNAME RealName "$FULLNAME"
dscl . -create /Users/$USERNAME UniqueID "$USERID"
dscl . -create /Users/$USERNAME PrimaryGroupID 20
dscl . -create /Users/$USERNAME NFSHomeDirectory /Users/$USERNAME
dscl . -passwd /Users/$USERNAME $PASSWORD
# Create the home directory
echo "Creating home directory..."
createhomedir -c 2>&1 | grep -v "shell-init"
fi
# Add user to any specified groups
echo "Adding user to specified groups..."
for GROUP in $SECONDARY_GROUPS ; do
dseditgroup -o edit -t user -a $USERNAME $GROUP
done
echo "Created user #$USERID: $USERNAME ($FULLNAME)"
exit 0
Solved! Go to Solution.
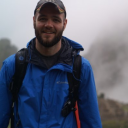
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-02-2015 04:02 AM
It's likely your usage of if and else. It should be more to the form:
if [ ]; then
elif [ ]; then
elif [ ]; then
else
fi
I would also recommend combining the call for 10.10 and 10.11(perhaps with a -gt "10" comparison) into one line as well as adding in checks for Domain Accounts.

- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-02-2015 04:17 AM
Just tested it and edited as follows. Works fine on my 10.11 Mac now...
#!/bin/sh
# create_user_1.2.sh
#
#
#
if [ "$(id -u)" != "0" ]; then
echo "Sorry, you are not root. Please run with sudo!"
exit 1
fi
# === For creating a User we need some input! ===
echo "Enter your desired user name: "
read USERNAME
echo "Enter a full name for this user: "
read FULLNAME
echo "Enter a password for this user: "
read -s PASSWORD
# ====
# A list of groups the user should belong to
# This makes the difference between admin and non-admin users.
echo "Is this an administrative user? (y/n)"
read GROUP_ADD
if [ "$GROUP_ADD" = n ] ; then
SECONDARY_GROUPS="staff" # for a non-admin user
elif [ "$GROUP_ADD" = y ] ; then
SECONDARY_GROUPS="admin _lpadmin _appserveradm _appserverusr" # for an admin user
else
echo "Please make a selection!"
fi
# ====
# Create a UID that is not currently in use
echo "Creating an unused UID for new user..."
# Find out the next available user ID
MAXID=$(dscl . -list /Users UniqueID | awk '{print $2}' | sort -ug | tail -1)
USERID=$((MAXID+1))
# check the OS X Version
OSXVERSION=$(sw_vers -productVersion | awk -F '.' '{print $1 "." $2}')
#if osx 10.10 then run
if [[ "$OSXVERSION" == "10.11" ]]; then
echo "OS is 10.11"
sysadminctl -addUser $USERNAME -fullName "$FULLNAME" -UID=$USERID -password $PASSWORD
#if osx 10.10 then run
elif [[ "$OSXVERSION" == "10.10" ]]; then
echo "OS is 10.10"
sysadminctl -addUser $USERNAME -fullName "$FULLNAME" -UID=$USERID -password $PASSWORD
#if osx 10.9 then run
elif [[ "$OSXVERSION" == "10.9" ]]; then
# Create the user account by running dscl
echo "Creating necessary files..."
dscl . -create /Users/$USERNAME
dscl . -create /Users/$USERNAME UserShell /bin/bash
dscl . -create /Users/$USERNAME RealName "$FULLNAME"
dscl . -create /Users/$USERNAME UniqueID "$USERID"
dscl . -create /Users/$USERNAME PrimaryGroupID 20
dscl . -create /Users/$USERNAME NFSHomeDirectory /Users/$USERNAME
dscl . -passwd /Users/$USERNAME $PASSWORD
# Create the home directory
echo "Creating home directory..."
createhomedir -c 2>&1 | grep -v "shell-init"
fi
# Add user to any specified groups
echo "Adding user to specified groups..."
for GROUP in $SECONDARY_GROUPS ; do
dseditgroup -o edit -t user -a $USERNAME $GROUP
done
echo "Created user #$USERID: $USERNAME ($FULLNAME)"
exit 0
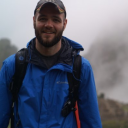
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-02-2015 08:44 AM
You should strip out the min version and do the comparison that way.
OSVERSION=$(sw_vers -productVersion | awk -F. '{print $2}')
if [[ $OSVERSION -ge 10 ]]; then
- covers all OS's 10.10 and later
elif [[ $OSVERSION -lt 10 && $OSVERSION -ge 7 ]]; then
- covers 10.9-10.7
else
- some sort of tough luck message
fi
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-02-2015 08:53 AM
@davidacland I believe the last bit in your code is what led to older jamf scripts not working with 10.10.
You should do this instead:
OSXVERSION=$(sw_vers -productVersion | awk -F '.' '{print $1 "." $2}' | cut -d . -f 2)
The relevant change is at the end where d signifies a delimiter of "." to separate the values and f says to use field 2.
Not saying it should be a problem, but if Apple somehow gets to OS X 10.100.X......
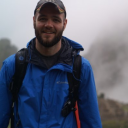
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-02-2015 04:02 AM
It's likely your usage of if and else. It should be more to the form:
if [ ]; then
elif [ ]; then
elif [ ]; then
else
fi
I would also recommend combining the call for 10.10 and 10.11(perhaps with a -gt "10" comparison) into one line as well as adding in checks for Domain Accounts.

- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-02-2015 04:17 AM
Just tested it and edited as follows. Works fine on my 10.11 Mac now...
#!/bin/sh
# create_user_1.2.sh
#
#
#
if [ "$(id -u)" != "0" ]; then
echo "Sorry, you are not root. Please run with sudo!"
exit 1
fi
# === For creating a User we need some input! ===
echo "Enter your desired user name: "
read USERNAME
echo "Enter a full name for this user: "
read FULLNAME
echo "Enter a password for this user: "
read -s PASSWORD
# ====
# A list of groups the user should belong to
# This makes the difference between admin and non-admin users.
echo "Is this an administrative user? (y/n)"
read GROUP_ADD
if [ "$GROUP_ADD" = n ] ; then
SECONDARY_GROUPS="staff" # for a non-admin user
elif [ "$GROUP_ADD" = y ] ; then
SECONDARY_GROUPS="admin _lpadmin _appserveradm _appserverusr" # for an admin user
else
echo "Please make a selection!"
fi
# ====
# Create a UID that is not currently in use
echo "Creating an unused UID for new user..."
# Find out the next available user ID
MAXID=$(dscl . -list /Users UniqueID | awk '{print $2}' | sort -ug | tail -1)
USERID=$((MAXID+1))
# check the OS X Version
OSXVERSION=$(sw_vers -productVersion | awk -F '.' '{print $1 "." $2}')
#if osx 10.10 then run
if [[ "$OSXVERSION" == "10.11" ]]; then
echo "OS is 10.11"
sysadminctl -addUser $USERNAME -fullName "$FULLNAME" -UID=$USERID -password $PASSWORD
#if osx 10.10 then run
elif [[ "$OSXVERSION" == "10.10" ]]; then
echo "OS is 10.10"
sysadminctl -addUser $USERNAME -fullName "$FULLNAME" -UID=$USERID -password $PASSWORD
#if osx 10.9 then run
elif [[ "$OSXVERSION" == "10.9" ]]; then
# Create the user account by running dscl
echo "Creating necessary files..."
dscl . -create /Users/$USERNAME
dscl . -create /Users/$USERNAME UserShell /bin/bash
dscl . -create /Users/$USERNAME RealName "$FULLNAME"
dscl . -create /Users/$USERNAME UniqueID "$USERID"
dscl . -create /Users/$USERNAME PrimaryGroupID 20
dscl . -create /Users/$USERNAME NFSHomeDirectory /Users/$USERNAME
dscl . -passwd /Users/$USERNAME $PASSWORD
# Create the home directory
echo "Creating home directory..."
createhomedir -c 2>&1 | grep -v "shell-init"
fi
# Add user to any specified groups
echo "Adding user to specified groups..."
for GROUP in $SECONDARY_GROUPS ; do
dseditgroup -o edit -t user -a $USERNAME $GROUP
done
echo "Created user #$USERID: $USERNAME ($FULLNAME)"
exit 0
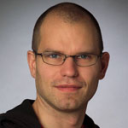
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-02-2015 08:18 AM
Thanks! It works now.
How would you put the Yosemite and ElCapitan-Check into one line?
Best,
Holger
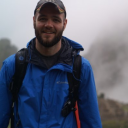
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-02-2015 08:44 AM
You should strip out the min version and do the comparison that way.
OSVERSION=$(sw_vers -productVersion | awk -F. '{print $2}')
if [[ $OSVERSION -ge 10 ]]; then
- covers all OS's 10.10 and later
elif [[ $OSVERSION -lt 10 && $OSVERSION -ge 7 ]]; then
- covers 10.9-10.7
else
- some sort of tough luck message
fi

- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-02-2015 08:49 AM
You could just add a cheeky cut -c 4-5
onto the end of your OSXVERSION variable and then use if [[ $OSXVERSION -ge 9 ]]; then...
Something like OSXVERSION=$(sw_vers -productVersion | awk -F '.' '{print $1 "." $2}' | cut -c 4-5)
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-02-2015 08:53 AM
@davidacland I believe the last bit in your code is what led to older jamf scripts not working with 10.10.
You should do this instead:
OSXVERSION=$(sw_vers -productVersion | awk -F '.' '{print $1 "." $2}' | cut -d . -f 2)
The relevant change is at the end where d signifies a delimiter of "." to separate the values and f says to use field 2.
Not saying it should be a problem, but if Apple somehow gets to OS X 10.100.X......

- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-02-2015 08:55 AM
@bpavlov good point!
Wouldn't be the first time cut has backfired on me!
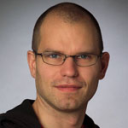
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-02-2015 09:35 AM
Nice, thank you all! Great script for efficient work...
so, here is a 'final' (what is final in the Apple-World? :) one:
#!/bin/sh
#
##############################
# create_user.sh
##############################
#
if [ "$(id -u)" != "0" ]; then
echo "Sorry, you are not root."
exit 1
fi
# === For creating a User we need some input! ===
echo "Enter your desired user name: "
read USERNAME
echo "Enter a full name for this user: "
read FULLNAME
echo "Enter a password for this user: "
read -s PASSWORD
# ====
# A list of (secondary) groups the user should belong to
# This makes the difference between admin and non-admin users.
echo "Is this an administrative user? (y/n)"
read GROUP_ADD
if [ "$GROUP_ADD" = n ] ; then
SECONDARY_GROUPS="staff" # for a non-admin user
elif [ "$GROUP_ADD" = y ] ; then
SECONDARY_GROUPS="admin _lpadmin _appserveradm _appserverusr" # for an admin user
else
echo "You did not make a valid selection!"
fi
# ====
# check the OS X Version
OSXVERSION=$(sw_vers -productVersion | awk -F '.' '{print $2}')
# Create a UID that is not currently in use
echo "Creating an unused UID for new user..."
# Find out the next available user ID
MAXID=$(dscl . -list /Users UniqueID | awk '{print $2}' | sort -ug | tail -1)
USERID=$((MAXID+1))
#if 10.10 or more then run
if [[ "$OSXVERSION" -ge 10 ]]; then
echo "Your installation is 10.10+. Using sysadminctl. "
sysadminctl -addUser $USERNAME -fullName "$FULLNAME" -UID=$USERID -password $PASSWORD
#if 10.7 to 10.9 then run
elif [[ "$OSXVERSION" -ge 7 ]]; then
echo "Mac OS 10.7 - to 10.9 is installed, using dscl."
# Create the user account by running dscl (normally you would have to do each of these commands one
# by one in an obnoxious and time consuming way.
echo "Creating necessary files..."
dscl . -create /Users/$USERNAME
dscl . -create /Users/$USERNAME UserShell /bin/bash
dscl . -create /Users/$USERNAME RealName "$FULLNAME"
dscl . -create /Users/$USERNAME UniqueID "$USERID"
dscl . -create /Users/$USERNAME PrimaryGroupID 20
dscl . -create /Users/$USERNAME NFSHomeDirectory /Users/$USERNAME
dscl . -passwd /Users/$USERNAME $PASSWORD
# Create the home directory
echo "Creating home directory..."
createhomedir -c 2>&1 | grep -v "shell-init"
fi
# Add user to any specified groups
echo "Adding user to specified groups..."
for GROUP in $SECONDARY_GROUPS ; do
dseditgroup -o edit -t user -a $USERNAME $GROUP
done
echo "Created user #$USERID: $USERNAME ($FULLNAME)"
exit 0
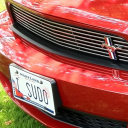
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-10-2015 06:37 AM
I have one edit to recommend.
line 36 of the script will find the highest current UID.
MAXID=$(dscl . -list /Users UniqueID | awk '{print $2}' | sort -ug | tail -1)
LDAP and AD users have UIDs above 1000 (over 1B for AD).
If you have an LDAP or AD user, the UID is too high for a local user.
I would suggest searching for the highest UID below 1000.
MAXID=$(dscl . -list /Users UniqueID | awk '{if($2<999)print $2}' | sort -ug | tail -1)
This will give you a UID suitable for a local user account.
