Current Network Extension Attribute
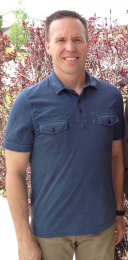
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 05-01-2012 02:22 PM
Does anyone have a good solution to returning the current AirPort network as an extension attribute that works on MacBook Pro and MacBook Air hardware?
The trouble I'm running into is that on a Lion machine, I can't get "AirPort" information from the "networksetup -getairportnetwork" if I don't designate en0 or en1 instead of using the common name of AirPort.
On the MBP and everything else, asking it to return results on en1 works fine because that generally is the AirPort interface. However, in MBAs (or other hardware that has the AirPort interface set to something else), all it will do is return an error.
Here's what I've been working with. And, yes, I did borrow most of it from an XML file I found in another post on JAMF Nation. :^)
#!/bin/sh
# Determine the OS version since the networksetup command differs on OS
OS=/usr/bin/sw_vers -productVersion | /usr/bin/colrm 5
if [[ "$OS" < "10.5" ]]; then
# Ensure that the networksetup link exists on 10.4 and earlier
if [ -f /System/Library/CoreServices/RemoteManagement/ARDAgent.app/Contents/Support/networksetup ];then
result=/System/Library/CoreServices/RemoteManagement/ARDAgent.app/Contents/Support/networksetup -getairportnetwork | sed 's/Current AirPort Network: //g'
else
result=The networksetup binary is not present on this machine.
fi
elif [ "$OS" == "10.5" ]; then
result=/usr/sbin/networksetup -getairportnetwork | sed 's/Current AirPort Network: //g'
else
result=/usr/sbin/networksetup -getairportnetwork en0 | sed 's/Current AirPort Network: //g'
fi
# Ensure that AirPort was found
hasen0=echo "$result" | grep "Error"
# Report the result
if [ "$hasAirPort" == "" ]; then
echo "<result>$result</result>"
else
echo "<result>No AirPort Device Found.</result>"
fi
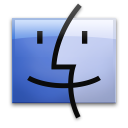
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 05-01-2012 02:29 PM
This can likely be handled a few different ways, but one way might be to also check the model of the Mac its being run on and adjust the "en" accordingly.
Try this to get model info quickly without using system_profiler:
ioreg -c IOPlatformExpertDevice | awk '/model/{print $NF}' | sed -e 's/"//g' -e 's/[<>]//g'
You can drop that into a $var and grep for "Air" for example to see if the script is being run against a MacBook Air and then do an if/then/else in your script to set the Ethernet to check against to "en0" or "en1", depending on what it finds.
As I said though, there are most likely better ways to figure out what the active Airport port actually is. Someone else may know that and chime in here.
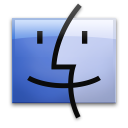
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 05-01-2012 02:42 PM
Ok, so here's another possible way to get what Airport is assigned to. I don't necessarily like invoking system_profiler since its a bit slow, but this may help.
Also, I don't have a MacBook Air handy I can test this against, so I have no idea if this will work on those. Try it out though.
system_profiler SPNetworkDataType | grep -A 1 "AirPort" | awk '/BSD/{ print $4 }'
On my MacBook Pro it returns "en1" I assume it would return "en0" on an Air.
Edit: Aaaand, yet a 3rd way! This one doesn't use system_profiler, it uses 'networksetup' instead, which may be a little more efficient. Pick your poison :)
/usr/sbin/networksetup -listallhardwareports | grep -A 1 Wi-Fi | awk '/Device/{ print $2 }'
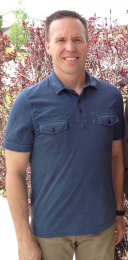
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 08-13-2013 08:36 AM
I got this to work.
#!/bin/sh
# Determine the OS version since the networksetup command differs on OS
OS=/usr/bin/sw_vers -productVersion | /usr/bin/colrm 5
# Determine the WiFi interface since different hardware has different EN designation
EN=/usr/sbin/networksetup -listallhardwareports | grep -A 1 Wi-Fi | awk '/Device/{ print $2 }'
if [[ "$OS" < "10.5" ]]; then
# Ensure that the networksetup link exists on 10.4 and earlier
if [ -f /System/Library/CoreServices/RemoteManagement/ARDAgent.app/Contents/Support/networksetup ];then
result=/System/Library/CoreServices/RemoteManagement/ARDAgent.app/Contents/Support/networksetup -getairportnetwork | sed 's/Current AirPort Network: //g'
else
result=The networksetup binary is not present on this machine.
fi
elif [ "$OS" == "10.5" ]; then
result=/usr/sbin/networksetup -getairportnetwork | sed 's/Current AirPort Network: //g'
else
result=/usr/sbin/networksetup -getairportnetwork "$EN" | sed 's/Current AirPort Network: //g'
fi
# Ensure that AirPort was found
hasen1=echo "$result" | grep "Error"
# Report the result
if [ "$hasAirPort" == "" ]; then
echo "<result>$result</result>"
else
echo "<result>No AirPort Device Found.</result>"
fi
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 01-06-2015 01:33 PM
I know this an older discussion.
Not sure if this helps anyone but I changed my EA to display all interface detection on Mac OS 10.10.1. I simply adjusted the following:
I left this in the script: #!/bin/sh
OS=/usr/bin/sw_vers -productVersion | /usr/bin/colrm 5
I adjusted this:
if [[ "$OS" < "10" ]]; then
That ended up working good for me. I am using that in both my active network interface and network service list EA. I am sure you can add to display any interface you want specifically. I needed this to work from my clients running 10.10 and 10.10.1. It had been blank for some time displaying the error "The networksetup binary is not present on this machine."
Now all is good.
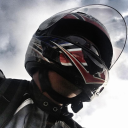
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 02-26-2015 01:14 PM
@dvasquez thanks for sharing your fix it worked great for me.
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 02-26-2015 01:24 PM
Very cool glad to help.
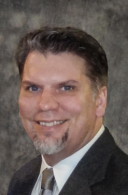
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 05-01-2015 11:21 AM
This works exactly as stated. Edit your current EA if your scripts results are "The networksetup binary is not present on this machine."
Edit this line:
if [[ "$OS" < "10" ]]; then
Thanks again for posting.
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 05-01-2015 12:29 PM
@k12techman — Hope you don't mind, but I took the liberty of cleaning up the bash script you posted slightly. Here's what I did:
- Set env to bash.
- More robust OS X version detection.
- Using
$(..)
to assign the output of a command to a variable. - Tabbing and whitespace cleanup to make it more readable.
Revised "Current wi-fi SSID" bash script
#!/bin/bash
# Determine the OS version since the networksetup command differs on OS
OS_major=$(/usr/bin/sw_vers -productVersion | awk -F . '{print $1}')
OS_minor=$(/usr/bin/sw_vers -productVersion | awk -F . '{print $2}')
# Determine the WiFi interface since different hardware has different EN designation
EN=$(/usr/sbin/networksetup -listallhardwareports | grep -A 1 Wi-Fi | awk '/Device/{ print $2 }')
if [[ "$OS_major" -eq 10 && "$OS_minor" -lt 5 ]]; then
# Ensure that the networksetup link exists on 10.4 and earlier
if [[ -f /System/Library/CoreServices/RemoteManagement/ARDAgent.app/Contents/Support/networksetup ]]; then
result=$(/System/Library/CoreServices/RemoteManagement/ARDAgent.app/Contents/Support/networksetup -getairportnetwork | sed 's/Current AirPort Network: //g')
else
result="The networksetup binary is not present on this machine."
fi
elif [[ "$OS_major" -eq 10 && "$OS_minor" -eq 5 ]]; then
# 10.5
result=$(/usr/sbin/networksetup -getairportnetwork | sed 's/Current AirPort Network: //g')
else
# 10.6+
result=$(/usr/sbin/networksetup -getairportnetwork "$EN" | sed 's/Current AirPort Network: //g')
fi
# Ensure that AirPort was found
hasen1=$(echo "$result" | grep "Error")
# Report the result
if [ "$hasen1" == "" ]; then
echo "<result>$result</result>"
else
echo "<result>No AirPort Device Found.</result>"
fi
exit 0
The revised bash script seems to work well for me in limited testing.
However, we actually use a Python script on our production servers to pull this information. Here's the script we use:
"Current wi-fi SSID" python script
#!/usr/bin/python
import re
import subprocess
AIRPORT = '/System/Library/PrivateFrameworks/Apple80211.framework/Versions/Current/Resources/airport'
NETWORK_SETUP = '/usr/sbin/networksetup'
def get_wireless_interface():
'''Returns the wireless interface device name, (e.g., en0 or en1).'''
hardware_ports = subprocess.check_output([NETWORK_SETUP,
'-listallhardwareports'])
match = re.search("(AirPort|Wi-Fi).*?(en\d)", hardware_ports, re.S)
if match:
wireless_interface = match.group(2)
return wireless_interface
def get_current_wlan(interface):
'''Returns the currently connected WLAN name.'''
wireless_status = subprocess.check_output([AIRPORT,
'-I',
interface]).split('
')
current_wlan_name = None
for line in wireless_status:
match = re.search(r' +SSID:s(.*)', line)
if match:
current_wlan_name = match.group(1)
return current_wlan_name
def print_current_wlan():
'''Prints the currently connected WLAN in a <result></result> tag.'''
wireless_interface = get_wireless_interface()
current_wlan = get_current_wlan(wireless_interface)
if current_wlan:
print '<result>%s</result>' % current_wlan
if __name__ == '__main__':
print_current_wlan()
The Python script has been working well for us for a while now. Open to suggestions if anybody sees a way to make it even better/more reliable.
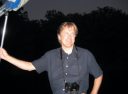
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 10-21-2015 03:27 PM
I had a script I was using as an extension attribute for this that broke with 10.10.
I tried this one : https://jamfnation.jamfsoftware.com/viewProductFile.html?id=135&fid=750
But I could not get it to work. I tried the Python one posted by @elliotjordan above and it works very well. I even tested it with a 10.11 machine and that worked fine as well. Thanks @elliotjordan
