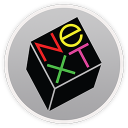
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 02-06-2024 02:27 PM
Can anyone tell me what I'm doing wrong? I'm trying to write a script that checks the version of macOS so I can have it trigger a Jamf policy based on the version of macOS that the computer is running. I wanted to account for all versions of Sonoma and all versions of Ventura. I keep getting "integer expression expected" errors. I used shellcheck.net and it didn't find any problems. I'm testing this in CodeRunner. I also tested it in Terminal. I thought I knew how to do this! I have even looked at some other scripts that I have that use similar syntax. They work.
#!/bin/zsh
macOSVersion=$(/usr/bin/sw_vers | grep "ProductVersion" | /usr/bin/awk '{ print $2 }')
echo "$macOSVersion"
if [ "$macOSVersion" -ge 14 ] && [ "$macOSVersion" -lt 15 ]; then
echo "This Mac is running macOS Sonoma"
elif [ "$macOSVersion" -ge 13 ] && [ "$macOSVersion" -lt 14 ]; then
echo "This Mac is running macOS Ventura"
else
echo "This Mac is running a macOS earlier than Ventura"
fi
Solved! Go to Solution.
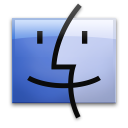
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 02-06-2024 02:46 PM
When checking if a value is greater than or less than in zsh, you need to use double brackets in your test. So something like this.
if [[ "$macOSVersion" -ge 14 ]] && [[ "$macOSVersion" -lt 15 ]]; then
Do the same for the elif line. Try it then. It shouldn't generate that error. The single brackets can only be used for string checks, not integer ones.
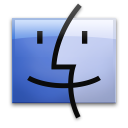
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 02-06-2024 02:46 PM
When checking if a value is greater than or less than in zsh, you need to use double brackets in your test. So something like this.
if [[ "$macOSVersion" -ge 14 ]] && [[ "$macOSVersion" -lt 15 ]]; then
Do the same for the elif line. Try it then. It shouldn't generate that error. The single brackets can only be used for string checks, not integer ones.
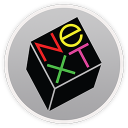
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 02-06-2024 03:26 PM
Thanks! I later changed my variable to just the major version of macOS "14", "13", etc. that worked. I will also try your suggestion. This was driving me nucking futs!
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 02-07-2024 06:49 AM
Just curious about why you would use the script in order to run a policy based on macOS version instead of scoping the policy to a smart group based on macOS version?
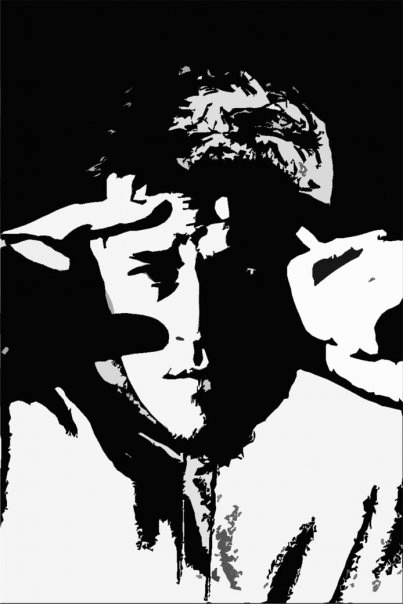
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 02-07-2024 11:55 AM
I've used a function like this inside of a script that I use to apply Dock settings. It has to determine the OS version so that it knows which version of System Settings (or System Preferences) is needed. It's easier to use a function like this rather than create 3 different versions of the same script.
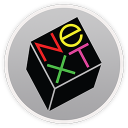
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 02-08-2024 12:13 PM
The reason is because this is for a policy that deploys our screen savers. I don't want to maintain two different policies. The policy works by first checking what macOS version is running, then it runs the appropriate script for that OS. We still have many Macs running Ventura. Most of our Macs are running Sonoma. I found a good solution for screen savers for Sonoma. Obviously there are more ways than one to do things.
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 02-07-2024 10:23 AM
Instead of grepping for ProductVersion, you can just run:
sw_vers -productVersion
