JSS REST API C# example?
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on
03-03-2016
04:38 PM
- last edited on
03-04-2025
03:03 AM
by
kh-richa_mig
Does anyone have working JSS REST API code in c#? I am trying to write an application that can grab basic computer information but I cannot find any good examples of C# and REST API.
If you have any sample code, sharing is greatly appreciated.
Thanks.
Edit:
I figured it out.
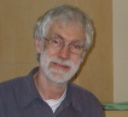
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 03-05-2016 09:04 PM
How about sharing. I would be interested too :-)
Regards
Graeme
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-19-2018 10:54 AM
@Graeme Sorry it took so long, for some reason I never saw your request.
public class Casper
{
public string casperUsr = GlobalVar.CasperUsername;
public string casperPaswrd = GlobalVar.CasperPassword;
public bool computerExist = false;
public bool computerDeleteSuccess = false;
public bool computerCreateSuccess = false;
public string confirmMessage = "";
public string macDTMUrl = "https://<your URL>:8443/JSSResource/computers/name/";
public string macDTMUrlAdd = "https://<your URL>:8443/JSSResource/computers/id/0";
public string macDTMUrlAddPath = "JSSResource/computers/id/0";
public void GetCasperInfo(string computerName)
{
var client = new RestClient(macDTMUrl + computerName);
client.Authenticator = new HttpBasicAuthenticator(casperUsr, casperPaswrd);
var request = new RestRequest(Method.GET);
request.AddHeader("content-type", "application/x-www-form-urlencoded");
IRestResponse response = client.Execute(request);
if (response.StatusCode == HttpStatusCode.OK)
{
computerExist = true;
//MessageBox.Show("OK Was recevied");
}
else if (response.StatusCode == HttpStatusCode.NotFound)
{
//MessageBox.Show("Not Found Received");
computerExist = false;
}
else if (response.StatusCode == HttpStatusCode.Unauthorized)
{
MessageBox.Show("Your account lacks sufficient privleges: " + response.StatusCode);
computerExist = false;
}
else
{
MessageBox.Show("Unhandled Error: " + response.StatusCode);
computerExist = false;
}
}
public void DeleteCasperComputer(string computerName)
{
var client = new RestClient(macDTMUrl + computerName);
client.Authenticator = new HttpBasicAuthenticator(casperUsr, casperPaswrd);
var request = new RestRequest(Method.DELETE);
request.AddHeader("content-type", "application/x-www-form-urlencoded");
IRestResponse response = client.Execute(request);
if (response.StatusCode == HttpStatusCode.OK)
{
//MessageBox.Show("OK on Delete recevied");
computerDeleteSuccess = true;
}
else if (response.StatusCode == HttpStatusCode.NotFound)
{
computerDeleteSuccess = false;
MessageBox.Show("Computer not found so it could not be deleted");
}
else if (response.StatusCode == HttpStatusCode.Unauthorized)
{
MessageBox.Show("Your account lacks sufficient privleges: " + response.StatusCode);
computerDeleteSuccess = false;
}
else
{
MessageBox.Show("Unhandled Error: " + response.StatusCode);
computerDeleteSuccess = false;
}
}
public void addComputer(string computerName, string MACAddress, string serialNumber, string siteID)
{
string addString = "<?xml version ="1.0" encoding="ISO-8859-1"?><computer><general><name>" + computerName + "</name><mac_address>" + MACAddress + "</mac_address><serial_number>" + serialNumber + "</serial_number><site><id>" + siteID + "</id></site></general></computer>";
RestClient client = new RestClient(macDTMUrlAdd);
client.Authenticator = new HttpBasicAuthenticator(casperUsr, casperPaswrd);
RestRequest request = new RestRequest(Method.POST);
request.AddHeader("content-type", "application/xml");
request.AddParameter("application/xml", addString, ParameterType.RequestBody);
IRestResponse response = client.Execute(request);
if (response.StatusCode == HttpStatusCode.Created)
{
computerCreateSuccess = true;
}
else if (response.StatusCode == HttpStatusCode.Unauthorized)
{
MessageBox.Show("Your account lacks sufficient privleges: " + response.StatusCode);
computerCreateSuccess = false;
}
else
{
MessageBox.Show("Add computer failed: " + response.StatusCode);
computerCreateSuccess = false;
}
}
public void Message()
{
confirmMessage = "Casper: ";
if (computerExist && computerDeleteSuccess)
{
confirmMessage += GlobalVar.computerName + " has been removed from Casper " + Environment.NewLine;
}
else if (computerExist && !computerDeleteSuccess)
{
confirmMessage += GlobalVar.computerName + " could not be removed from Casper " + Environment.NewLine;
}
else
{
confirmMessage += GlobalVar.computerName + " was not in Casper" + Environment.NewLine;
}
}
}
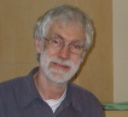
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-20-2018 03:16 PM
Many thanks
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-26-2018 05:49 AM
@Graeme You are welcome. I actually had to update it last week to use TLS 1.2 explicitly because we upgraded to 10.8. Here is the new code:
public class Casper
{
public string casperUsr = GlobalVar.CasperUsername;
public string casperPaswrd = GlobalVar.CasperPassword;
public bool computerExist = false;
public bool computerDeleteSuccess = false;
public bool computerCreateSuccess = false;
public string confirmMessage = "";
public string macDTMUrl = "https://<your url>:8443/JSSResource/computers/name/";
public string macDTMUrlAdd = "https://<your url>:8443/JSSResource/computers/id/0";
public string macDTMUrlAddPath = "JSSResource/computers/id/0";
public void GetCasperInfo(string computerName)
{
ServicePointManager.SecurityProtocol = SecurityProtocolType.Tls12;
var client = new RestClient(macDTMUrl + computerName);
client.Authenticator = new HttpBasicAuthenticator(casperUsr, casperPaswrd);
var request = new RestRequest(Method.GET);
request.AddHeader("content-type", "application/x-www-form-urlencoded");
IRestResponse response = client.Execute(request);
if (response.StatusCode == HttpStatusCode.OK)
{
computerExist = true;
//MessageBox.Show("OK Was recevied");
}
else if (response.StatusCode == HttpStatusCode.NotFound)
{
//MessageBox.Show("Not Found Received");
computerExist = false;
}
else if (response.StatusCode == HttpStatusCode.Unauthorized)
{
MessageBox.Show("Your account lacks sufficient privleges: " + response.StatusCode);
computerExist = false;
}
else
{
MessageBox.Show("Unhandled Error: " + response.StatusCode);
computerExist = false;
}
}
public void DeleteCasperComputer(string computerName)
{
ServicePointManager.SecurityProtocol = SecurityProtocolType.Tls12;
var client = new RestClient(macDTMUrl + computerName);
client.Authenticator = new HttpBasicAuthenticator(casperUsr, casperPaswrd);
var request = new RestRequest(Method.DELETE);
request.AddHeader("content-type", "application/x-www-form-urlencoded");
IRestResponse response = client.Execute(request);
if (response.StatusCode == HttpStatusCode.OK)
{
//MessageBox.Show("OK on Delete recevied");
computerDeleteSuccess = true;
}
else if (response.StatusCode == HttpStatusCode.NotFound)
{
computerDeleteSuccess = false;
MessageBox.Show("Computer not found so it could not be deleted");
}
else if (response.StatusCode == HttpStatusCode.Unauthorized)
{
MessageBox.Show("Your account lacks sufficient privleges: " + response.StatusCode);
computerDeleteSuccess = false;
}
else
{
MessageBox.Show("Unhandled Error: " + response.StatusCode);
computerDeleteSuccess = false;
}
}
public void addComputer(string computerName, string MACAddress, string serialNumber, string siteID)
{
ServicePointManager.SecurityProtocol = SecurityProtocolType.Tls12;
string addString = "<?xml version ="1.0" encoding="ISO-8859-1"?><computer><general><name>" + computerName + "</name><mac_address>" + MACAddress + "</mac_address><serial_number>" + serialNumber + "</serial_number><site><id>" + siteID + "</id></site></general><extension_attributes><extension_attribute><id>68</id><name>BuildComputerName</name><value>" + computerName + "</value></extension_attribute></extension_attributes></computer>";
//string addString = "<?xml version ="1.0" encoding="ISO-8859-1"?><computer><general><name>" + computerName + "</name><mac_address>" + MACAddress + "</mac_address><serial_number>" + serialNumber + "</serial_number><site><id>" + siteID + "</id></site></general></computer>";
RestClient client = new RestClient(macDTMUrlAdd);
client.Authenticator = new HttpBasicAuthenticator(casperUsr, casperPaswrd);
RestRequest request = new RestRequest(Method.POST);
request.AddHeader("content-type", "application/xml");
request.AddParameter("application/xml", addString, ParameterType.RequestBody);
IRestResponse response = client.Execute(request);
if (response.StatusCode == HttpStatusCode.Created)
{
computerCreateSuccess = true;
}
else if (response.StatusCode == HttpStatusCode.Unauthorized)
{
MessageBox.Show("Your account lacks sufficient privleges: " + response.StatusCode);
computerCreateSuccess = false;
}
else
{
MessageBox.Show("Add computer failed: " + response.StatusCode + " response message " + response.ErrorMessage + "response content" + response.Content);
computerCreateSuccess = false;
}
}
public void Message()
{
confirmMessage = "Casper: ";
if (computerExist && computerDeleteSuccess)
{
confirmMessage += GlobalVar.computerName + " has been removed from Casper " + Environment.NewLine;
}
else if (computerExist && !computerDeleteSuccess)
{
confirmMessage += GlobalVar.computerName + " could not be removed from Casper " + Environment.NewLine;
}
else
{
confirmMessage += GlobalVar.computerName + " was not in Casper" + Environment.NewLine;
}
}
}
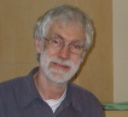
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-26-2018 01:19 PM
Thanks again.
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 08-09-2023 07:22 AM
Here is the code I'm using to retrieve data from jamf in c#
public async void button1_Click(object sender, EventArgs e)
{
var JamfDataRetriever = new JamfDataRetriever();
List<DevFromJamF> managedJamfInventoryDevices = await JamfDataRetriever.GetJamfDevices();
}
public class JamfDataRetriever
{
static HttpClient client = new HttpClient();
private static HttpClient sharedClient = new()
{
BaseAddress = new Uri("https://myServer.jamfcloud.com"),
};
public async Task<List<DevFromJamF>> GetJamfDevices()
{
string urlT = "https://myServer.jamfcloud.com/api/v1/auth/token";
string url = "https://myServer.jamfcloud.com";
string authEndpoint = "/uapi/auth/tokens";
string uName = "apiaccessAccount"; //
string pWord = "ApiAccessPassword"; //
string r = string.Empty;
//------------------------------------------------------------------------------------------------------------
var content = new StringContent("", System.Text.Encoding.UTF8, "application/json");
client.DefaultRequestHeaders.Authorization = new System.Net.Http.Headers.AuthenticationHeaderValue(
"Basic", Convert.ToBase64String(System.Text.Encoding.ASCII.GetBytes($"{uName}:{pWord}")));
// Send the POST request
HttpResponseMessage response = await client.PostAsync($"{urlT}", content);
// Check the response status
string token = "";
string expires = "";
if (response.IsSuccessStatusCode)
{
// Request was successful
string responseBody = await response.Content.ReadAsStringAsync();
// Parse the JSON response
JsonDocument jsonDocument = JsonDocument.Parse(responseBody);
JsonElement root = jsonDocument.RootElement;
// Extract the token value and expiration date
token = root.GetProperty("token").GetString();
expires = root.GetProperty("expires").GetString();
Console.WriteLine("Token: " + token);
Console.WriteLine("Expires: " + expires);
}
else
{
// Request failed
Console.WriteLine("Request failed with status code: " + response.StatusCode);
}
//------------------------------------------------------------------------------------------------------------
if (token == "")
{
token = "7u_Rt_Sg986ScVGYRdU"; // for testing
}
string? bearerToken = token;
string sections = "GENERAL,HARDWARE,USER_AND_LOCATION, OPERATING_SYSTEM";
//sections= ###Supported Values are: GENERAL, DISK_ENCRYPTION, PURCHASING, APPLICATIONS, STORAGE, USER_AND_LOCATION, CONFIGURATION_PROFILES, PRINTERS, SERVICES, HARDWARE, LOCAL_USER_ACCOUNTS, CERTIFICATES, ATTACHMENTS, PLUGINS, PACKAGE_RECEIPTS, FONTS, SECURITY, OPERATING_SYSTEM, LICENSED_SOFTWARE, IBEACONS, SOFTWARE_UPDATES, EXTENSION_ATTRIBUTES, CONTENT_CACHING, GROUP_MEMBERSHIPS
HttpClient inventoryRequest = new HttpClient();
inventoryRequest.DefaultRequestHeaders.Add("Authorization", "Bearer " + bearerToken);
inventoryRequest.DefaultRequestHeaders.Add("Accept", "application/json");
HttpResponseMessage responseInv = await inventoryRequest.GetAsync($"{url}/api/v1/computers-inventory?section={sections}&page=0&page-size=1000");
// Check the response status
if (responseInv.IsSuccessStatusCode)
{
string responseBody = await responseInv.Content.ReadAsStringAsync();
// Parse the JSON response
JsonDocument jsonDocument = JsonDocument.Parse(responseBody);
JsonElement root = jsonDocument.RootElement;
// Get the computers array
JsonElement computers = root.GetProperty("results");
TibsDbContext curContext = new TibsDbContext();
curContext.Database.ExecuteSqlRaw("TRUNCATE TABLE DevFromJamF");
int i = 1;
using (var _db = new TibsDbContext())
{
foreach (JsonElement computer in computers.EnumerateArray())
{
JsonElement general = computer.GetProperty("general");
JsonElement remoteManagement = general.GetProperty("remoteManagement");
JsonElement hardware = computer.GetProperty("hardware");
JsonElement userAndLocation = computer.GetProperty("userAndLocation");
JsonElement operatingSystem = computer.GetProperty("operatingSystem");
string computerName = general.GetProperty("name").GetString();
string userName = userAndLocation.GetProperty("username").GetString();
string versionOS = operatingSystem.GetProperty("version").GetString();
string serialNumber = hardware.GetProperty("serialNumber").GetString();
string lastContactTime = (general.GetProperty("lastContactTime").GetString());
DevFromJamF newDevFromJamF = new DevFromJamF();
newDevFromJamF.Host = computerName;
newDevFromJamF.Username = userName;
newDevFromJamF.Osfull = versionOS;
newDevFromJamF.SerialNo = serialNumber;
if (lastContactTime != "" && lastContactTime != null)
{ newDevFromJamF.LastCheckIn = DateTime.Parse(lastContactTime); }
_db.DevFromJamF.Add(newDevFromJamF);
_db.SaveChanges();
}
}
}
else
{
Console.WriteLine("Error: " + (int)response.StatusCode + " " + response.ReasonPhrase);
}
return new List<DevFromJamF>();
}
