Nice little scripts to scope to stolen computers
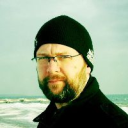
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 10-23-2014 11:23 AM
So, from time to time we get computers stolen, and more often than one might think, they don't get erased but rather just gets a new admin account (using the .applesetupdone trick).
Our users aren't too happy about us collecting geo-location on a daily basis so we crafted a nifty little script that gives us the adress of the stolen computer. The script gets scoped to a static group containing all computers that are stolen (or lost) and set to run ongoing on all triggers (to get as much data as possible). As soon as the computer reports back in to the JSS, these scripts starts collecting data.
Along with the adress-script we have another script that prints the safari surf history of the current logged in user to stdin so it gets into the policy log to be viewed and finally one script that prints out the external IP and DNS name of the computer.
The adress scripts works by listing all the wifi networks seen by the computer and crafts a URL that requests data from google. The result is the approximate adress of the computers present location and the coordinates and finally the accuracy of the positioning.
We have recovered several computers using these scripts as all we have to do is provide law enforcement with location, IP/ISP and some "proof" of who we think is using the computer (you can often tell by the surf log since people tend to log into sites like Facebook and email which nicely prints out the name in the page title and therefore gets logged using the surf history part).
The record shows that the script is amazingly correct, or rather, Google is extremely good at provide location just using wifi networks. Most of the time, Google reports an accuracy of "150" which I guess would refer to 150 meters but from experience, that could easily be 15 meters, it's that accurate!
These scripts work for us, your mileage may vary, computers may catch fire, the scripts may contains bugs and so on...
Nevertheless, here are the scripts:
To get external IP:
#!/bin/sh
IP=$(dig +short myip.opendns.com @resolver1.opendns.com)
DNS=$(host $IP | awk '{print $5}' | sed "s/.$//g")
echo "$IP - $DNS"
To log the surf history to the JSS (nb does not work in 10.10 as Safari now saves the history in a sqlite 3-file):
#!/bin/sh
unset LANG
#echo "START"
PREFIX="/tmp/safari-log"
USER=$(w | grep console | awk '{print $1}')
eval cp ~$USER/Library/Safari/History.plist $PREFIX-history-bin.plist
plutil -convert xml1 -o $PREFIX-history-xml.plist $PREFIX-history-bin.plist
split -p "WebHistoryDomains.v2" $PREFIX-history-xml.plist $PREFIX-
tail -n +5 $PREFIX-aa | egrep -o "(<string>.*</string>)|(<dict>)" | sed -E "s/</?string>//g" | sed "s/<dict>//g" | grep -v "^http://www.google.*,d.Yms$" > $PREFIX-history.txt
OLD_IFS=$IFS
IFS=""
exec 5<$PREFIX-history.txt
while read -u 5 LINE
do
echo $LINE | egrep -s "^[0-9.]+$" > /dev/null
if [ $? -ne 0 ] ; then
echo $LINE
else
TIME=$(expr $(echo $LINE | egrep -o "^[0-9]*") + 978307200)
date -r $TIME
fi
done
IFS=$OLD_IFS
rm $PREFIX-*
#echo "END"
And finally the one that prints out the address (location):
#!/bin/sh
INTERFACE=$(networksetup -listallhardwareports | grep -A1 Wi-Fi | tail -1 | awk '{print $2}')
STATUS=$(networksetup -getairportpower $INTERFACE | awk '{print $4}')
if [ $STATUS = "Off" ] ; then
sleep 5
networksetup -setairportpower $INTERFACE on
fi
/System/Library/PrivateFrameworks/Apple80211.framework/Versions/A/Resources/airport -s | tail -n +2 | awk '{print substr($0, 34, 17)"$"substr($0, 52, 4)"$"substr($0, 1, 32)}' | sort -t $ -k2,2rn | head -12 > /tmp/gl_ssids.txt
if [ $STATUS = "Off" ] ; then
networksetup -setairportpower $INTERFACE off
fi
OLD_IFS=$IFS
IFS="$"
URL="https://maps.googleapis.com/maps/api/browserlocation/json?browser=firefox&sensor=false"
exec 5</tmp/gl_ssids.txt
while read -u 5 MAC SS SSID
do
SSID=`echo $SSID | sed "s/^ *//g" | sed "s/ *$//g" | sed "s/ /%20/g"`
MAC=`echo $MAC | sed "s/^ *//g" | sed "s/ *$//g"`
SS=`echo $SS | sed "s/^ *//g" | sed "s/ *$//g"`
URL+="&wifi=mac:$MAC&ssid:$SSID&ss:$SS"
done
IFS=$OLD_IFS
#echo $URL
curl -s -A "Mozilla" "$URL" > /tmp/gl_coordinates.txt
LAT=`cat /tmp/gl_coordinates.txt | grep "lat" | awk '{print $3}' | tr -d ","`
LONG=`cat /tmp/gl_coordinates.txt | grep "lng" | awk '{print $3}' | tr -d ","`
ACC=`cat /tmp/gl_coordinates.txt | grep "accuracy" | awk '{print $3}' | tr -d ","`
#echo "LAT: $LAT"
#echo "LONG: $LONG"
#echo "ACC: $ACC"
curl -s -A "Mozilla" "http://maps.googleapis.com/maps/api/geocode/json?latlng=$LAT,$LONG&sensor=false" > /tmp/gl_address.txt
ADDRESS=`cat /tmp/gl_address.txt | grep "formatted_address" | head -1 | awk '{$1=$2=""; print $0}' | sed "s/,$//g" | tr -d " | sed "s/^ *//g"`
if [ $EA -ne 0 ] ; then
echo "<result>$ADDRESS (lat=$LAT, long=$LONG, acc=$ACC)</result>"
else
echo "$ADDRESS (lat=$LAT, long=$LONG, acc=$ACC)"
fi
rm /tmp/gl_ssids.txt /tmp/gl_coordinates.txt /tmp/gl_address.txt
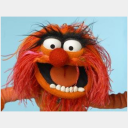
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 09-03-2017 06:10 PM
@guidotti nope. entered my API key and it still fails
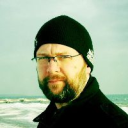
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 10-26-2017 08:53 AM
I haven't gotten around to fix this, but I really need to. It's been a while, but I think I somehow "reverse-engineered" how an android phone finds out it's location with no GPS. I'd have to go back and see if I saved the info on how I got the info, heh.
I'll get back to this thread.
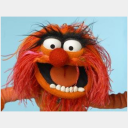
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 01-03-2018 05:34 AM
@bollman Any luck on fixing this?
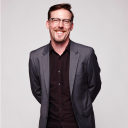
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 01-31-2018 09:05 AM
Considering we're now trying to track down a lost system, sure am wishing this script was working!!!! Agh!!!
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 01-31-2018 02:45 PM
@ChrisJScott-work You could download and install prey if it's still checking in. https://www.preyproject.com/
There is an api key that you can use to link it to your account when it's installed. We just recently tracked down a stolen laptop with it.
#!/bin/sh
#download prey
curl -o /private/tmp/prey-mac-1.7.3-x64.pkg "https://downloads.preyproject.com/prey-client-releases/node-client/1.7.3/prey-mac-1.7.3-x64.pkg"
#Run Prey Installer
API_KEY=yourkey sudo -E installer -pkg /private/tmp/prey-mac-1.7.3-x64.pkg -target /
#Remove Temp File
rm /private/tmp/prey-mac-1.7.3-x64.pkg
exit 0

- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 01-31-2018 03:31 PM
Nice and thanks for sharing.
We just firmware lock the machines, and put up a desktop wallpaper that says "We're not sure how you got this machine, but it was stolen" and then do a bunch of things:
- Disable terminal.app, iTunes, updates, printers
- Set accounts to log out after 3 minutes and set a screen saver to 1 minute
- Require a new password of ridiculous requirements be set every day
- Continuously remove any admin accounts
- Limit surfing to just Bing, Google, and Yahoo
- Make everything in the dock as big as possible
- Make everything on the desktop as big as possible
- Limit all external media to read-only
- Restrict all preferences, sharing services, functionality (camera, iCloud, etc),
- Hide most of the button under the Apple icon
- Enable Parental Controls and limit the computers to 90 minutes a day
@Nick_Gooch - Be aware that scripts are stored on the local machine prior to execution, and it is possible to scrape API keys for installers and JAMF API calls from any scripts deployed to a client. I'd be careful of putting such items on a stolen machine.
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 01-31-2018 04:13 PM
@Seven What is the danger of them getting the api key to prey? There is nothing in the prey account aside from the stolen computer?

- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 01-31-2018 04:38 PM
@Nick_Gooch - Well, it looks like prey can be a paid for service, so you'd be exposing your API creds to being stolen, which could lead to you paying for someone else to use the product.
Overall, I was just cautioning that users can see the raw content of scripts, so just a reminder to people in the thread to be careful about putting sensitive creds for licenses, accounts, or APIs in the scripts.
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 02-01-2018 07:41 AM
Thanks, I see what you are saying. I'm not to concerned in this case but could see how that could be a problem. Would putting it in as a script variable be any better?
We used the free version with the one stolen laptop we had so far. After prey was installed the police recovered it in less then an hour.
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 03-17-2018 09:42 AM
just looking at this but can see it still needs fixing

- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 03-19-2018 03:16 AM
Install Prey, it is really worth it !
A former employee left with his work laptop and I needed a way to make sure he had the computer.
This script was not working anymore so I created a free account with prey.
The API number is at the bottom of the settings page.
Create a script in Jamf with the following content :
#!/bin/sh
#download prey
curl -o /private/tmp/prey-mac-1.7.3-x64.pkg "https://downloads.preyproject.com/prey-client-releases/node-client/1.7.3/prey-mac-1.7.3-x64.pkg"
#Run Prey Installer
API_KEY=CHANGETHIS sudo -E installer -pkg /private/tmp/prey-mac-1.7.3-x64.pkg -target /
#Remove Temp File
rm /private/tmp/prey-mac-1.7.3-x64.pkg
exit 0
Scope it to the Mac you are looking for.
You can "monitor" 3 computers at once with the free version of prey.
as soon as prey is installed, you will receive a mail alert, put the computer in lost mode in prey and wait for the location and screenshots form the isight camera + desktop, to populate reports.
Enjoy !
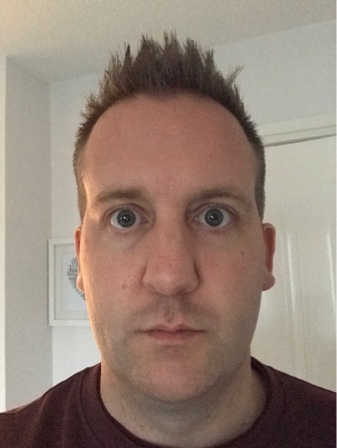
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 07-05-2018 01:25 AM
Thanks @Xaviermlp your script has worked a charm and Prey has installed on the stolen laptop. Now to pass the info over to the Police an hopefully we will get the MacBook back.
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 07-12-2018 02:51 PM
Prey is awesome. The very first screenshot we got was the user creating a new Apple ID with their full name and address. Less then an hour later the police had our laptop. It still took a few months before we were able to take possession of it.

- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 02-08-2019 04:41 AM
Thank you @Xaviermlp !
It works great on Mojave too, but..... the Privacy alert for the camera pops up.
Is there any way to force-allow that? The PPPC only gives the option to deny access.

- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 02-08-2019 08:05 AM
Ok, that's new, thanks for sharing.
I would start here : https://derflounder.wordpress.com/2018/08/31/creating-privacy-preferences-policy-control-profiles-for-macos/ .

- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 02-08-2019 08:06 AM
Oh man, just found this : https://blog.fleetsmith.com/tcc-a-quick-primer/ and it states that :
Camera and Microphone access can only be denied via the Payload Profile. They cannot be allowed/whitelisted.

- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 02-11-2019 02:53 AM
@Xaviermlp Thanks. I already found that it can only be set to deny ;-)
Checked the prey-forum: https://community.preyproject.com/t/mojave-pppc-with-camera-captures-in-lost-mode/1644
but no answers there (yet).
Really hope for a workaround there.
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 04-15-2019 09:53 AM
Hi there,
I am really interested in the Prey app. I have a stolen laptop and did successfully install using the scripts. But I can't seem to add the stolen computer to my Prey account. How is that done? Thanks....
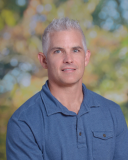
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 04-16-2019 05:43 AM
@aks if you set your API_KEY in the script the device will automatically be added to your prey account when the install completes.
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 04-23-2019 09:29 AM
Thanks! I pushed the script out, then nothing happened for a few days. Then all of a sudden, it was there. I was tracking it and had my local PD call the PD where the laptop was (1/2 way across the continent) and they picked it up at a McDonalds. Ends up the thief had 2 felony warrants out for his arrest. No telling when I will actually get the laptop back now that the PD has it, but that's ok. Neat app! Thanks for recommending it!
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 04-25-2019 02:22 PM
Thanks for the help @Xaviermlp ! Would you mind sharing the details around the policy that you use to scope this script to the target computers, such as Triggers, execution frequency, etc?
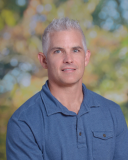
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 04-26-2019 08:46 AM
@jzarate Here's a shot of mine and I just add any serial numbers reported as missing to the scope.
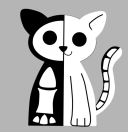
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 12-24-2019 05:21 AM
Looks like if you want to silently deploy Prey on a stolen device, you're out of luck on newer machines due to PPPC limitations. Official response from Prey: "This can’t be done, I’m sorry. This Apple policy can’t be bypassed via MDM.".
I wish Apple would come up with a "Lost Mode" for macOS devices (similar to what already exists for supervised iOS devices). That way, users would be assured that IT could only retrieve geolocation data in the event of the device being lost, with full transparency and an audit trail.
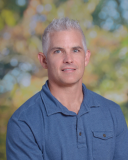
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 12-24-2019 06:57 AM
I officially deactivated our Prey account because of this @sim.brar
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 01-29-2020 10:55 AM
@TomDay, will the script no longer install Prey at all, or would it just no longer install secretly? Have you found any other solutions?
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 04-21-2021 06:50 AM
@bollman Did you ever figure out a way to fix this script? Or any way to track down a lost mac? I know a lot of people were using prey, but since it cant be installed silently on Catalina its not an option for us.
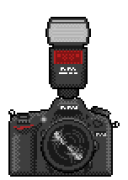
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 02-03-2022 07:41 PM
Old post, but we just had a new MBA swiped. Would love a way to track it down if possible. Anyone have a way that works with Mojave and later?
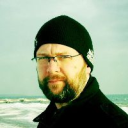
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 02-11-2022 05:40 AM
Sadly no, I haven't had the time to investigate this further. I was hoping that some open source alternative to google's wifi location service would pop up, but the last time I checked I couldn't find anything.
Nowadays, I usually trust Geoip, but for pinpointing exactly where the computer is, well, that's not good enough.
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 07-30-2024 12:22 AM
I've tried to update the script but critical part of it will not work on Sonoma+ systems due to deprecation of airport cli utility... I've tried to use some 3d party solutions but all of them needs to be whitelisted with Location Services permissions. Google Location API works fine with new token system and JSON body requests.
