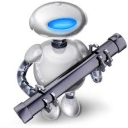
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-06-2015 08:49 AM
I want to check to see if a couple of directories exist and if not create them before running rest of the script
I’m using if-then-else statements but that is not working for me. I find I have to run the script 3 times: 1st-time it creates the first directory, 2nd – it creates the second directory and then 3rd – time it runs the rest of the script. After the directories have been created the script runs fine. I just don’t want it to have to run it three times before it works as it should.
Thanks
#!/bin/sh
if [ ! -d $ZZdesktop ]; then
mkdir -p $ZZdesktop
else
if [ ! -d $ZZdocuments ]; then
mkdir -p $ZZdocuments
else
rsync -ahv --progress --delete "/Users/$CUser/Desktop/" /Users/$CUser/Box Sync/$CUser/zz_backup/z_desktop/ 2>> /Users/Shared/${CUser}syncerror_desktop
fi
fi
Solved! Go to Solution.
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-06-2015 08:53 AM
It's because of the "else" statements. First time it runs, it does "mkdir -p $ZZdesktop" and then bails on the rest because the rest is in an "else" statement. It will follow the "else" branch the next time it runs.
I think you can just make them two separate "if" statements.
#!/bin/sh
if [ ! -d $ZZdesktop ]; then
mkdir -p $ZZdesktop
fi
if [ ! -d $ZZdocuments ]; then
mkdir -p $ZZdocuments
fi
rsync -ahv --progress --delete "/Users/$CUser/Desktop/" /Users/$CUser/Box Sync/$CUser/zz_backup/z_desktop/ 2>> /Users/Shared/${CUser}syncerror_desktop
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-06-2015 08:53 AM
It's because of the "else" statements. First time it runs, it does "mkdir -p $ZZdesktop" and then bails on the rest because the rest is in an "else" statement. It will follow the "else" branch the next time it runs.
I think you can just make them two separate "if" statements.
#!/bin/sh
if [ ! -d $ZZdesktop ]; then
mkdir -p $ZZdesktop
fi
if [ ! -d $ZZdocuments ]; then
mkdir -p $ZZdocuments
fi
rsync -ahv --progress --delete "/Users/$CUser/Desktop/" /Users/$CUser/Box Sync/$CUser/zz_backup/z_desktop/ 2>> /Users/Shared/${CUser}syncerror_desktop
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-06-2015 10:36 AM
would definitely go with what @alexjdale says. if you insist on using 'else' though here is what it would look like
#!/bin/sh
if [ ! -d $ZZdesktop ]; then
mkdir -p $ZZdesktop
mkdir -p $ZZdocuments
rsync -ahv --progress --delete "/Users/$CUser/Desktop/" /Users/$CUser/Box Sync/$CUser/zz_backup/z_desktop/ 2>> /Users/Shared/${CUser}syncerror_desktop
else
if [ ! -d $ZZdocuments ]; then
mkdir -p $ZZdocuments
rsync -ahv --progress --delete "/Users/$CUser/Desktop/" /Users/$CUser/Box Sync/$CUser/zz_backup/z_desktop/ 2>> /Users/Shared/${CUser}syncerror_desktop
else
rsync -ahv --progress --delete "/Users/$CUser/Desktop/" /Users/$CUser/Box Sync/$CUser/zz_backup/z_desktop/ 2>> /Users/Shared/${CUser}syncerror_desktop
fi
fi
Not pretty. A lot of duplicated effort.
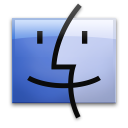
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-06-2015 10:45 AM
+1 to @alexjdale's suggestion. Check for each needed directory in its own if/then block. By the time the script reaches the rsync line, everything should be in place to run correctly.
Although its not actually clear why those folders are being created based on what you posted. I assume there is more to the script we aren't seeing.
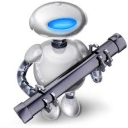
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-06-2015 11:26 AM
Thanks @alexjdale so simple, @bpave777 not sure why I was thinking it needed the else statement.
@mm2270 No I did not post the entire script, just the part that had me stumped, thanks.
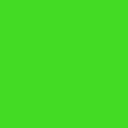
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-09-2015 05:06 AM
If you are going to run the same command multiple times, you could set an array and then iterate.
#!/bin/bash
declare -a MakeDirs=( '/Volumes/Users/user1/test'
'/Volumes/Users/user2/test' )
for each_dir in "${MakeDirs[@]}"
do
if [[ ! -d "$each_dir" ]]
then
mkdir -p "$each_dir"
fi
done
exit 0
