Verify password is correct
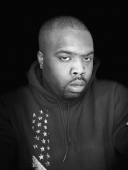
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 06-06-2020 05:39 PM
So I am trying to create an upgrade macOS Catalina policy in self service, but I want a dialog box to appear prompting the user for their password. If that password is correct, then it will run the upgrade policy. If the password is not correct, it fails.
This is what I have so far, but the policy triggers whether the password is correct or not.
#!/bin/bash
## Get the logged in user's name
userName=$(/usr/bin/stat -f%Su /dev/console)
buttonClicked=$("/Library/Application Support/JAMF/bin/jamfHelper.app/Contents/MacOS/jamfHelper" -windowType "utility" -icon "/Users/Shared/logo.png" -title "WARNING!" -alignDescription center -description "Verification is required to proceed." -button1 "OK" -button2 Cancel -defaultButton 2)
if [[ "$?" == "0" ]]; then
echo "Prompting ${userName} for their login password."
userPass=$(/usr/bin/osascript -e "
on run
display dialog "To continue with macOS Catalina update" & return & return & "Enter login password for '$userName'" default answer "" buttons {"Cancel", "Ok"} default button 2 with icon POSIX file "/Users/Shared/logo.png" with text and hidden answer
set userPass to text returned of the result
return userPass
end run")
if [ "$?" == "0" ]; then
sudo jamf policy -trigger macOSCatalina
fi
fi
- Labels:
-
Scripts
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 06-08-2020 12:41 AM
Hello Fernadez
We have similar workflow to enable a mobile account (AD) into the FileVault enable users.
I've changed our script to get close to your needs.
I would stick to one UI method either Jamf helper or osascript.
The script does prompt for the user password, check if the value is not empty or if the osascript has timeout (need to cover all possible variables).
Also as this is a self-service process I removed the Cancel button, no need to start something if it will be cancelled later, but that is the fine tuning of your process.
Note jamf is running as root, no need for sudo in sudo jamf policy -trigger macOSCatalina.
Furthermore as this is running as root we will need to change to the user environment so
sudo -u "$userName" osascript ....
Our script was using text for different languages (localization) but I've removed that so you will see some repetition.
Basically:
1. Prompt for password
2. Check if it is not empty
3. Check if it is valid
#!/bin/bash
#########################################################################################
#
# FUNCTIONS
#
#########################################################################################
wrongUserPassword() {
userResponce=$(sudo -u "$userName" osascript <<EOF
use AppleScript version "2.4" -- Yosemite (10.10) or later
use scripting additions
set theTextReturned to "nil"
set theIcon to POSIX file "/Users/Shared/logo.png" as alias
set appTitle to "macOS Catalina update"
set okTextButton to "OK"
set enterPassword to "Enter your password to allow this"
set passwordWrong to "Incorrect Password"
set errorPasswordText to passwordWrong & return & enterPassword
set theResponse to display dialog {errorPasswordText} default answer "" buttons {okTextButton} default button 1 with title {appTitle} with icon theIcon with hidden answer
set theTextReturned to the text returned of theResponse
if theTextReturned is "nil" then
return "cancelled"
else if theTextReturned is "" then
return "nopassword"
else
return theTextReturned
end if
EOF
)
validatePassword "$userResponce"
}
noUserPassword() {
userResponce=$(sudo -u "$userName" osascript <<EOF
use AppleScript version "2.4" -- Yosemite (10.10) or later
use scripting additions
set theTextReturned to "nil"
set theIcon to POSIX file "/Users/Shared/logo.png" as alias
set appTitle to "macOS Catalina update"
set okTextButton to "OK"
set enterPassword to "Enter your password to allow this"
set passwordEmpty to "The password is empty."
set errorPasswordText to passwordEmpty & return & enterPassword
set theResponse to display dialog {errorPasswordText} default answer "" buttons {okTextButton} default button 1 with title {appTitle} with icon theIcon with hidden answer
set theTextReturned to the text returned of theResponse
if theTextReturned is "nil" then
return "cancelled"
else if theTextReturned is "" then
return "nopassword"
else
return theTextReturned
end if
EOF
)
validatePassword "$userResponce"
}
promptUserPassword() {
userResponce=$(sudo -u "$userName" osascript <<EOF
use AppleScript version "2.4" -- Yosemite (10.10) or later
use scripting additions
set theTextReturned to "nil"
set theIcon to POSIX file "/Users/Shared/logo.png" as alias
set appTitle to "macOS Catalina update"
set okTextButton to "OK"
set changesText to "To continue with macOS Catalina update" & return & return & "Enter login password for $userName"
set theResponse to display dialog {changesText} default answer "" buttons {okTextButton} default button 1 with title {appTitle} with icon theIcon with hidden answer
set theTextReturned to the text returned of theResponse
if theTextReturned is "nil" then
return "cancelled"
else if theTextReturned is "" then
return "nopassword"
else
return theTextReturned
end if
EOF
)
}
verifyPassword() {
dscl . authonly "$userName" "$userResponce" &> /dev/null; resultCode=$?
if [ "$resultCode" -eq 0 ];then
echo "Password Check: PASSED"
# DO THE REST OF YOUR ACTIONS....
jamf policy -trigger macOSCatalina
else
# Prompt for User Password
echo "Password Check: WRONG PASSWORD"
wrongUserPassword
fi
}
validatePassword() {
case "$userResponce" in
"nopassword" ) echo "Password Check: NO PASSWORD" & noUserPassword ;;
"cancelled" ) echo "Password Check: Time Out" & exit 1 ;;
* ) verifyPassword ;;
esac
}
##########################################################################################
#
# SCRIPT CONTENTS
#
##########################################################################################
# Get the Username of the currently logged user
userName=$(ls -la /dev/console | cut -d " " -f 4)
# Ask the logged user for the password
promptUserPassword "$userName"
# Check is password is correct
validatePassword "$userResponce"
Hope this is helpful
Regards
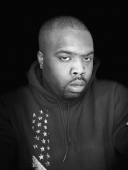
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 06-08-2020 10:07 PM
I ended up getting it to work using the following:
#!/bin/sh
################################## VARIABLES ##################################
jamfHelper="/Library/Application Support/JAMF/bin/jamfHelper.app/Contents/MacOS/jamfHelper"
PROMPT_TITLE="WARNING!"
MAIN_TITLE="macOS Catalina"
ERROR_TITLE="ERROR"
WARN_ICON="/System/Library/CoreServices/CoreTypes.bundle/Contents/Resources/AlertCautionIcon.icns"
ERROR_ICON="/System/Library/CoreServices/CoreTypes.bundle/Contents/Resources/AlertStopIcon.icns"
PROMPT_MESSAGE="Verification is required to proceed!"
FORGOT_PW_MESSAGE="Your password can not be verified.
Contact IT at help@help.com."
LOGO="/Users/Shared/logo.png"
LOGO_POSIX="$(/usr/bin/osascript -e 'tell application "System Events" to return POSIX file "'"$LOGO"'" as text')"
ERROR_POSIX="$(/usr/bin/osascript -e 'tell application "System Events" to return POSIX file "'"$ERROR_ICON"'" as text')"
CURRENT_USER=`ls -l /dev/console | awk '{ print $3 }'`
LOGGED_USER=`ls -l /dev/console | awk '{ print $3 }'`
GET_USER=$(ls -l /dev/console | awk '{ print $3 }')
FIRST_NAME=$(finger -s $GET_USER | head -2 | tail -n 1 | awk '{ print ($2)} ')
LAST_NAME=$(finger -s $GET_USER | head -2 | tail -n 1 | awk '{print ($3)}')
USER_ID=$(/usr/bin/id -u "$CURRENT_USER")
L_ID=$USER_ID
L_METHOD="asuser"
################################## MAIN PROCESS ##################################
# Display a branded prompt explaining the password prompt.
echo "Alerting user $CURRENT_USER about incoming password prompt..."
"$jamfHelper" -windowType "utility" -icon "$WARN_ICON" -title "$PROMPT_TITLE" -alignDescription center -description "$PROMPT_MESSAGE" -button1 "Next" -defaultButton 1 -startlaunchd &>/dev/null
# Get the logged in user's password via a prompt.
echo "Prompting $CURRENT_USER for their Mac password..."
USER_PASS="$(/bin/launchctl "$L_METHOD" "$L_ID" /usr/bin/osascript -e 'display dialog "To continue with macOS update
Enter login password for '$FIRST_NAME' '$LAST_NAME'" default answer "" with title "'"${MAIN_TITLE//"/\"}"'" giving up after 86400 with text buttons {"OK"} default button 1 with hidden answer with icon file "'"${LOGO_POSIX//"/\"}"'"' -e 'return text returned of result')"
# Validate the user's password
TRY=1
until /usr/bin/dscl /Search -authonly "$CURRENT_USER" "$USER_PASS" &>/dev/null; do
(( TRY++ ))
echo "Prompting $CURRENT_USER for their Mac password (attempt $TRY)..."
USER_PASS="$(/bin/launchctl "$L_METHOD" "$L_ID" /usr/bin/osascript -e 'display dialog "Sorry, that password was incorrect. Please try again." default answer "" with title "'"${ERROR_TITLE//"/\"}"'" giving up after 86400 with text buttons {"OK"} default button 1 with hidden answer with icon file "'"${ERROR_POSIX//"/\"}"'"' -e 'return text returned of result')"
if (( TRY >= 3 )); then
echo "[ERROR] Password prompt unsuccessful after 3 attempts. Displaying "forgot password" message..."
/bin/launchctl "$L_METHOD" "$L_ID" "$jamfHelper" -windowType "utility" -icon "$ERROR_ICON" -title "$ERROR_TITLE" -alignDescription center -description "$FORGOT_PW_MESSAGE" -button1 'OK' -defaultButton 1 -startlaunchd &>/dev/null &
exit 1
fi
done
echo "Successfully validated the correct password."
# Execute macOS Catalina Upgrade
sudo jamf policy -trigger macOS-Catalina
Its personalized by getting the user's first and last name, after 3 failed attempts, it cancels the process, and they have the cancel option just in case they decide to do this upgrade later. I'm actually going to incorporate this prompt to policies that are a bit more complex than flushing the dns or clearing a cache.
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 12-21-2020 07:14 AM
nice this is what im looking but
1) login user password was different, AD user password was different to sync do we have any script no application or enterprise app
2) user working from home and VPN need to connect for the validation check if not connected need to connect prompt
3) the user ID is the same in MAC login user validation required
4) no LAPS user just has a local Admin account having it
then all ok let sync the password to the AD logged account. if all success
pls help if has any script
