- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 06-07-2016 12:11 PM
Hey everyone! I've been looking to see if there is a way I can add multiple categories at once, maybe upload a CSV file or something. I want to create multiple categories that represents each one of our school locations, which is about 45, and some additional categories. Can this be done in JSS, Casper Admin, or something else?
Solved! Go to Solution.
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 06-07-2016 04:18 PM
@tech1man re-purposing a script I used for updating lease data, you could use the API to create these. Place the categories into a CSV file that is saved with UNIX LF, then pass the API user/pass and file name to the script.
#!/bin/bash
# Name: createCategories.sh
# Date: 7 June 2016
# Author: Steve Wood (swood@integer.com)
# Purpose: used to read in a list of categories from a CSV file and create them in the JSS
#
# **** The CSV file needs to be saved as a UNIX file with LF, not CR ****
# Version: 1.0
#
# A good portion of this script is re-purposed from the script posted in the following JAMF Nation article:
#
# https://jamfnation.jamfsoftware.com/discussion.html?id=13118#respond
#
# Usage: createCategories.sh apiuser apipass path-to-csv-file
# Variables
args=("$@")
jssAPIUsername="${args[0]}"
jssAPIPassword="${args[1]}"
jssAddress="https://your.jss.com:8443" # <-- make sure to put your JSS address here
file="${args[2]}"
#Verify we can read the file
data=`cat $file`
if [[ "$data" == "" ]]; then
echo "Unable to read the file path specified"
echo "Ensure there are no spaces and that the path is correct"
exit 1
fi
#Find how many categories to import
catqty=`awk -F, 'END {printf "%s
", NR}' $file`
echo "Category Qty = " $catqty
#Set a counter for the loop
counter="0"
duplicates=[]
#Loop through the CSV and submit data to the API
while [ $counter -lt $catqty ]
do
counter=$[$counter+1]
line=`echo "$data" | head -n $counter | tail -n 1`
category=`echo "$line" | awk -F , '{print $1}'`
echo "Attempting to add category named $category"
apiData="<category><name>$category</name><priority>5</priority></category>" # <-- you can edit priority to what you want
output=`curl -sS -k -i -u ${jssAPIUsername}:${jssAPIPassword} -X POST -H "Content-Type: text/xml" -d "<?xml version="1.0" encoding="ISO-8859-1"?>$apiData" ${jssAddress}/JSSResource/categories/id/0`
echo $output
#Error Checking
error=""
error=`echo $output | grep "Conflict"`
if [[ $error != "" ]]; then
duplicates+=($category)
fi
done
echo "The following categories could not be created:"
printf -- '%s
' "${duplicates[@]}"
exit 0
I tested really quickly on my JSS and it worked to create 6 categories in seconds. You can find this on my GitHub account here:
As always, test this in dev before implementing in production.
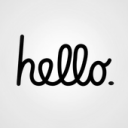
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 06-07-2016 03:26 PM
You could probably do this by writing directly to the database using an SQL statement, but for around 45 categories it's probably easier to just add them one by one either via the Casper Admin app or web interface.
Less clicking using the Casper Admin app!
Casper Admin app:
Web Interface:
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 06-07-2016 04:18 PM
@tech1man re-purposing a script I used for updating lease data, you could use the API to create these. Place the categories into a CSV file that is saved with UNIX LF, then pass the API user/pass and file name to the script.
#!/bin/bash
# Name: createCategories.sh
# Date: 7 June 2016
# Author: Steve Wood (swood@integer.com)
# Purpose: used to read in a list of categories from a CSV file and create them in the JSS
#
# **** The CSV file needs to be saved as a UNIX file with LF, not CR ****
# Version: 1.0
#
# A good portion of this script is re-purposed from the script posted in the following JAMF Nation article:
#
# https://jamfnation.jamfsoftware.com/discussion.html?id=13118#respond
#
# Usage: createCategories.sh apiuser apipass path-to-csv-file
# Variables
args=("$@")
jssAPIUsername="${args[0]}"
jssAPIPassword="${args[1]}"
jssAddress="https://your.jss.com:8443" # <-- make sure to put your JSS address here
file="${args[2]}"
#Verify we can read the file
data=`cat $file`
if [[ "$data" == "" ]]; then
echo "Unable to read the file path specified"
echo "Ensure there are no spaces and that the path is correct"
exit 1
fi
#Find how many categories to import
catqty=`awk -F, 'END {printf "%s
", NR}' $file`
echo "Category Qty = " $catqty
#Set a counter for the loop
counter="0"
duplicates=[]
#Loop through the CSV and submit data to the API
while [ $counter -lt $catqty ]
do
counter=$[$counter+1]
line=`echo "$data" | head -n $counter | tail -n 1`
category=`echo "$line" | awk -F , '{print $1}'`
echo "Attempting to add category named $category"
apiData="<category><name>$category</name><priority>5</priority></category>" # <-- you can edit priority to what you want
output=`curl -sS -k -i -u ${jssAPIUsername}:${jssAPIPassword} -X POST -H "Content-Type: text/xml" -d "<?xml version="1.0" encoding="ISO-8859-1"?>$apiData" ${jssAddress}/JSSResource/categories/id/0`
echo $output
#Error Checking
error=""
error=`echo $output | grep "Conflict"`
if [[ $error != "" ]]; then
duplicates+=($category)
fi
done
echo "The following categories could not be created:"
printf -- '%s
' "${duplicates[@]}"
exit 0
I tested really quickly on my JSS and it worked to create 6 categories in seconds. You can find this on my GitHub account here:
As always, test this in dev before implementing in production.
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 06-07-2016 04:20 PM
Just wanted to clarify that by using an ID of 0 in a POST command to the API, you can create a record. So you could create a computer, a category, a department, etc.
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 06-08-2016 05:47 AM
Thanks so much for your responses!
