API Data for ios device rename.
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on
09-16-2017
08:46 AM
- last edited on
03-04-2025
05:43 AM
by
kh-richa_mig
I am trying to create a script to rename a couple thousand IOS devices using a CSV file. I am unable to use the MUT tool because of how our JSS is structured for our departments. I am struggling with finding the API data I need to rename our new ipads. Any help would be appreciated.
- Labels:
-
Configuration Profiles
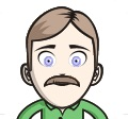
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 09-16-2017 10:19 AM
Here is one I have used in the past. I have not used it in a good while so TEST, TEST, and TEST again. Preferably not in production.
#!/bin/sh
# Name: iOSRenamer.sh
# Date: 19 February 2016
# Pieced together by: Mike Donovan (mike.donovan@killeenisd.org)
# Purpose: used to read in device names from a CSV file and update the record in the JSS
# Version: 1.0
#
# A good portion of this script is re-purposed from the scripts posted in the following JAMF Nation articles
# and Trey Howell's move records script on GitHub
#
# https://github.com/jamftrey/JSS-API-SCRIPTS/blob/master/mvrecords.sh
#
# https://jamfnation.jamfsoftware.com/discussion.html?id=13118#respond
#
# https://jamfnation.jamfsoftware.com/discussion.html?id=13663
#
# And others
#
# A CSV file with two columns for serial numbers in the first column and device name in the second column with NO HEADINGS will be needed
######## asks for JSS Address and adds variable
jssAddress="$(osascript -e 'Tell application "System Events" to display dialog "Enter JSS Address:" default answer "https://yourjssaddress.com:8443"' -e 'text returned of result' 2>/dev/null)"
if [ $? -ne 0 ]; then
# The user pressed Cancel
exit 1 # exit with an error status
elif [ -z "$jssAddress" ]; then
# The user left the project name blank
osascript -e 'Tell application "System Events" to display alert "You must enter a JSS Address; cancelling..." as warning'
exit 1 # exit with an error status
fi
############## asks for user for API and adds variable
jssAPIUsername="$(osascript -e 'Tell application "System Events" to display dialog "Enter API username: have same account on both JSS:" with hidden answer default answer ""' -e 'text returned of result' 2>/dev/null)"
if [ $? -ne 0 ]; then
# The user pressed Cancel
exit 1 # exit with an error status
elif [ -z "$jssAPIUsername" ]; then
# The user left the project name blank
osascript -e 'Tell application "System Events" to display alert "You must enter a JSS Address; cancelling..." as warning'
exit 1 # exit with an error status
fi
######### asks for password for API users and adds variable
jssAPIPassword="$(osascript -e 'Tell application "System Events" to display dialog "Enter API Password: have same account on both JSS:" default answer "" with hidden answer' -e 'text returned of result' 2>/dev/null)"
if [ $? -ne 0 ]; then
# The user pressed Cancel
exit 1 # exit with an error status
elif [ -z "$jssAPIPassword" ]; then
# The user left the project name blank
osascript -e 'Tell application "System Events" to display alert "You must enter a JSS Address; cancelling..." as warning'
exit 1 # exit with an error status
fi
######## asks for path to the CSV file
file="$(osascript -e 'Tell application "System Events" to display dialog "Enter path to the CSV file:" default answer "/Users/username/Desktop/DeviceRenamer.csv"' -e 'text returned of result' 2>/dev/null)"
if [ $? -ne 0 ]; then
# The user pressed Cancel
exit 1 # exit with an error status
elif [ -z "$file" ]; then
# The user left the project name blank
osascript -e 'Tell application "System Events" to display alert "You must enter a file path; cancelling..." as warning'
exit 1 # exit with an error status
fi
#Verify we can read the file
data=`cat $file`
if [[ "$data" == "" ]]; then
echo "Unable to read the file path specified"
echo "Ensure there are no spaces and that the path is correct"
exit 1
fi
#Find how many computers to import
computerqty=`awk -F, 'END {printf "%s
", NR}' $file`
echo "Computerqty= " $computerqty
#Set a counter for the loop
counter="0"
#Loop through the CSV and submit data to the API
while [ $counter -lt $computerqty ]
do
counter=$[$counter+1]
line=`echo "$data" | head -n $counter | tail -n 1`
serialNumber=`echo "$line" | awk -F , '{print $1}'`
newName=`echo "$line" | awk -F , '{print $2}'`
echo "Attempting to update name for $serialNumber"
## Create the xml file for later upload to the JSS
echo "<?xml version="1.0" encoding="UTF-8" standalone="no"?>
<mobile_devices>
<mobile_device>
<name>${newName}</name>
</mobile_device>
</mobile_devices>" > /tmp/DeviceRenamer.xml
## Upload xml to the JSS via API
curl -fsku "${jssAPIUsername}:${jssAPIPassword}" "${jssAddress}/JSSResource/mobile_devices/serialnumber/${serialNumber}" -T /tmp/DeviceRenamer.xml -X PUT
## Check to see if we got a 0 exit status from the PUT command
if [ $? == 0 ]; then
echo "Device "$serialNumber" name was updated to "$newName""
else
#echo "Rename failed"
echo "Device "$serialNumber" rename failed serial number may not exist in the JSS"
## Clean up the xml file
#rm -f "/tmp/DeviceRenamer.xml"
exit 1
fi
done
## Clean up the xml file
rm -f "/tmp/DeviceRenamer.xml"
exit 0
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 09-16-2017 10:37 AM
thank you my good sir
