- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on
02-13-2017
10:56 AM
- last edited on
03-04-2025
05:52 AM
by
kh-richa_mig
Here is a script that i use to rename computers remotely via Jamf. Many computers were not following our standard naming convention, such as computers named "Admin MacBook Pro", etc..
#!/bin/sh
serial=`/usr/sbin/system_profiler SPHardwareDataType | /usr/bin/awk '/Serial Number (system)/ {print $NF}'`
/usr/sbin/scutil --set ComputerName "CRP${serial}"
/usr/sbin/scutil --set LocalHostName "CRP${serial}"
/usr/sbin/scutil --set HostName "CRP${serial}"
sudo dsconfigad -force -remove -u YourMacBindingAccount -p YourBindingAccountPassword
sudo jamf policy -id 482
#### or with user interaction use this script:
serial=`/usr/sbin/system_profiler SPHardwareDataType | /usr/bin/awk '/Serial Number (system)/ {print $NF}'`
function machinename () {
osascript <<EOT
tell application "Finder"
activate
set nameentry to text returned of (display dialog "
Please name your computer.
The naming convention is the 3 letter
dept name,
followed by the serial number.
Example:
CRP${base}${serial}
The serial number for this Mac is:
${base}${serial}" default answer "" with icon 2)
end tell
EOT
}
function renameComputer(){
echo "The New Computer name is: $ComputerName"
scutil --set HostName $ComputerName
scutil --set LocalHostName $ComputerName
scutil --set ComputerName $ComputerName
echo Rename Successful
}
ComputerName=$(machinename)
renameComputer
sudo dsconfigad -force -remove -u YourMacBindingAccount -p BindingAccountPassword
sudo jamf policy -id 482
sudo jamf manage
sudo jamf recon
sudo /usr/local/jamf/bin/jamf displayMessage -message "To Join your Computer to our Corp Domain, please re-start your computer."
exit 0
Solved! Go to Solution.
- Labels:
-
Scripts
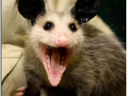
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 02-13-2017 11:44 AM
If the reason you're using system_profiler
is to avoid the double quotes returned by @nsantoro example you can remove them by piping the output to sed like this.
ioreg -l | awk '/IOPlatformSerialNumber/ { print $4;}' | sed -e 's/^"//' -e 's/"$//'
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 02-13-2017 11:21 AM
@nsantoro two quick things. First, you may want to re-post that using the "script" tags (the >_ icon above) so that the script is not messed up by formatting.
Second, instead of using system_profiler
to get the serial number of the machine, you may want to move over to using ioreg
as it is less resource heavy and quicker:
ioreg -l | awk '/IOPlatformSerialNumber/ { print $4;}'
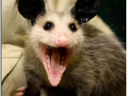
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 02-13-2017 11:44 AM
If the reason you're using system_profiler
is to avoid the double quotes returned by @nsantoro example you can remove them by piping the output to sed like this.
ioreg -l | awk '/IOPlatformSerialNumber/ { print $4;}' | sed -e 's/^"//' -e 's/"$//'
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 02-13-2017 01:39 PM
@stevewood thanks.
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 02-13-2017 04:30 PM
thank you @MadPossum & @stevewood I just made changes to my scripts and it works quicker.
#bin/sh
serial=`ioreg -l | awk '/IOPlatformSerialNumber/ { print $4;}' | sed -e 's/^"//' -e 's/"$//'`
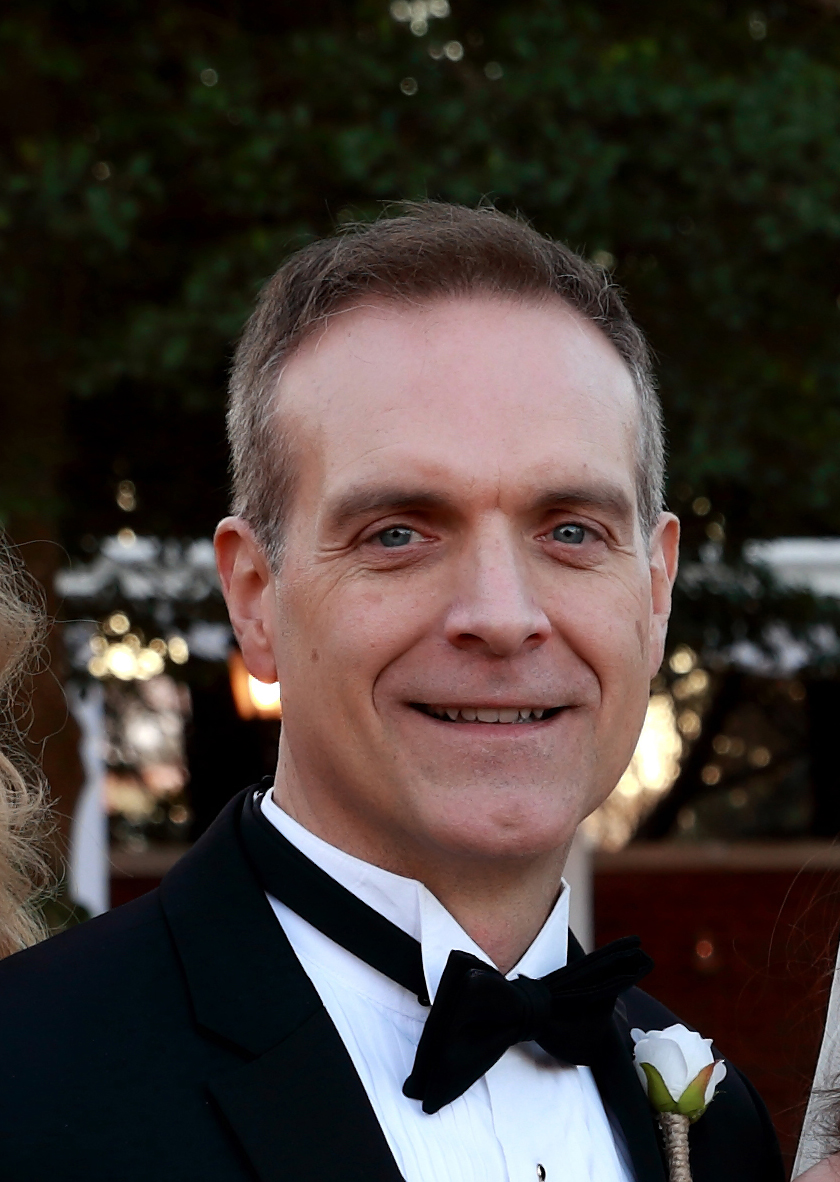
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 02-14-2017 08:21 AM
Hey @Nsantoro,
Question for you. Your script refers to this policy in your JSS:
sudo jamf policy -id 482
What is this policy being called doing? Is policy 482 an AD binding/unbinding action you've setup or something? (I'm looking to use your script here in our environment...it would help me as well..)
Thanks.
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 02-14-2017 08:28 AM
@steve.summers the policy call is pointing to another policy that i have in place which is a directory bind policy. Using Jamf Builtin function under options/ Directory Bindings.)
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 02-14-2017 10:02 AM
No User Interaction:
- Rename Computer name to proper naming convention
- Force Unbinds
- Calls to a Jamf Policy (mine is 482) - which Binds the Mac to the Domain.
#!/bin/sh
serial=`ioreg -l | awk '/IOPlatformSerialNumber/ { print $4;}' | sed -e 's/^"//' -e 's/"$//'`
/usr/sbin/scutil --set ComputerName "CRP${serial}"
/usr/sbin/scutil --set LocalHostName "CRP${serial}"
/usr/sbin/scutil --set HostName "CRP${serial}"
sudo dsconfigad -force -remove -u YourMacBindingAccount -p YourBindingAccountPassword
sudo jamf policy -id 482
This Script has user interaction.
I made this available to our onsite support techs: to bind Macs to the domain.
#!/bin/sh
serial=`ioreg -l | awk '/IOPlatformSerialNumber/ { print $4;}' | sed -e 's/^"//' -e 's/"$//'``
function machinename () {
osascript <<EOT
tell application "Finder"
activate
set nameentry to text returned of (display dialog "
Please name your computer.
The naming convention is the 3 letter
dept name,
followed by the serial number.
Example:
CRP${base}${serial}
The serial number for this Mac is:
${base}${serial}" default answer "" with icon 2)
end tell
EOT
}
function renameComputer(){
echo "The New Computer name is: $ComputerName"
scutil --set HostName $ComputerName
scutil --set LocalHostName $ComputerName
scutil --set ComputerName $ComputerName
echo Rename Successful
}
ComputerName=$(machinename)
renameComputer
sudo dsconfigad -force -remove -u YourMacBindingAccount -p BindingAccountPassword
sudo jamf policy -id 482
sudo jamf manage
sudo jamf recon
sudo /usr/local/jamf/bin/jamf displayMessage -message "To Join your Computer to our Corp Domain, please re-start your computer."
exit 0
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 07-31-2019 04:46 PM
I tried both commands to get the serial number:
ioreg -l | awk '/IOPlatformSerialNumber/ { print $4;}' | sed -e 's/^"//' -e 's/"$//'
/usr/sbin/system_profiler SPHardwareDataType | /usr/bin/awk '/Serial Number (system)/ {print $NF}'
My experience is the system_profiler method feels actually quite faster than ioreg.
