- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 03-25-2015 01:49 PM
I have been working on an extension attribute to display the size of the Outlook identity on client macs. I have taken some cues from the posting here , and although that script works for me it generates a list of all users and the directory names. I would like to simply get the size of the folder for the currently logged in user, as I am not interested in the Outlook folder sizes for our local admin or shared accounts. Here's what I have so far:
#!/bin/bash
echo "<result>"
if test -e /Users/$USER/Documents/Microsoft User Data/Office 2011 Identities ; then
du -sh /Users/$USER/Documents/Microsoft User Data/Office 2011 Identities | cut -f1
else echo "Not Found"
fi
echo "</result>"
exit 0
When i run this script locally, it returns
591M
Which is what i want. However, in the JSS, it always returns "Not Found". Any ideas on what is wrong here? It is probably something small and obvious, as I am fairly new to scripting
Solved! Go to Solution.
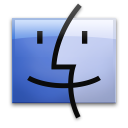
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 03-25-2015 02:11 PM
Its most likely the use of $USER in your script that its getting tripped up on. EA scripts run as root, not as the logged in or current user, so when you use $USER its looking to get the size of a folder in the root account's home directory, which doesn't exist of course.
Try something like this-
#!/bin/sh
loggedInUser=$(ls -l /dev/console | awk '{print $3}')
if [[ "$loggedInUser" != "root" ]] && [[ -e "/Users/${loggedInUser}/Documents/Microsoft User Data/Office 2011 Identities" ]]; then
result=$(du -sh "/Users/${loggedInUser}/Documents/Microsoft User Data/Office 2011 Identities" | awk '{print $1}')
else
result="N/A"
fi
echo "<result>$result</result>"
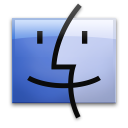
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 03-25-2015 02:11 PM
Its most likely the use of $USER in your script that its getting tripped up on. EA scripts run as root, not as the logged in or current user, so when you use $USER its looking to get the size of a folder in the root account's home directory, which doesn't exist of course.
Try something like this-
#!/bin/sh
loggedInUser=$(ls -l /dev/console | awk '{print $3}')
if [[ "$loggedInUser" != "root" ]] && [[ -e "/Users/${loggedInUser}/Documents/Microsoft User Data/Office 2011 Identities" ]]; then
result=$(du -sh "/Users/${loggedInUser}/Documents/Microsoft User Data/Office 2011 Identities" | awk '{print $1}')
else
result="N/A"
fi
echo "<result>$result</result>"
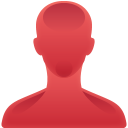
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 03-25-2015 02:15 PM
$USER would be root, assuming it is anything at all, when running an extension attribute. You'll need to iterate through user accounts to get results, and be prepared for multiple ones.
Completely untested, never run, freehand code:
#!/bin/sh
echo "<result>";
for i in `ls -d /Users/*`;
do
if test -e $i/Documents/Microsoft User Data/Office 2011 Identities ; then
echo -n "${i:7} - ";
du -sh $i/Documents/Microsoft User Data/Office 2011 Identities | cut -f1
fi;
done
echo "</result>"
A note to others who may stumble upon this: Don't assume everybody has a home folder in /Users/. Query dscl to find homes.
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 03-25-2015 02:16 PM
Interesting, as $USER worked for a different script I made...
Anyway, that looks like it works for me. Thanks!
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 02-26-2016 08:00 AM
@mm2270 How would one modify the script to return the size of just the most recently modified identity inside of "/Users/${loggedInUser}/Documents/Microsoft User Data/Office 2011 Identities" ?
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 02-26-2016 09:16 AM
you could possibly make use of:
ls -lt
which would sort a directory by last date modified. might need to use grep or awk to determine what line that is.
