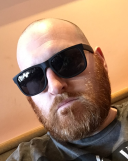
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on
04-09-2020
03:00 PM
- last edited on
03-04-2025
02:56 AM
by
kh-richa_mig
I have an an issue where I need to update some user fields in the API but I don't really know where to start.
In summary, I need to loop through all users and for every user whose 'managed_apple_id' field is blank/null I need to copy the data from the 'roster_managed_apple_id' field to the 'managed_apple_id' field.
Does that make sense? Is anyone able to help?
Solved! Go to Solution.
- Labels:
-
Scripts
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 04-14-2020 07:46 AM
Something like this may help you?
#!/bin/bash
jssURL="https://xxxxx.jamfcloud.com"
## DO NOT EDIT BELOW THIS LINE ##
echo "Please enter your JSS username:"
read jssUser
echo "Please enter your JSS password:"
read -s jssPass
usersToGetAtOneTime="10000"
# Make sure xmlstarlet is installed
if ! command -v xmlstarlet > /dev/null ; then
echo "You must install xmlstarlet before using this script."
echo "Try "brew install xmlstarlet""
exit 1
fi
# Get list of users
echo "Downloading list of user IDs..."
while read -r line; do
ids+=("$line")
done <<< "$(curl -X GET -k -s -u "$jssUser:$jssPass" "$jssURL/JSSResource/users" | xmlstarlet sel -t -v '/users/user/id')"
# Find users with roster_managed_apple_id in
echo "Gathering User Information"
count="$usersToGetAtOneTime"
for id in "${ids[@]}"; do
managedAppleID=$(curl -X GET -k -s -u "$jssUser:$jssPass" "$jssURL/JSSResource/users/id/$id" | xmlstarlet sel -t -v '/user/roster_managed_apple_id')
# Update Managed Apple ID if Roster Managed Apple ID Exists
if [ -z "$managedAppleID" ]
then
echo "No Managed Apple ID, moving on"
else
echo "$managedAppleID"
curl -X PUT -H "Accept: application/xml" -H "Content-type: application/xml" -k -u "$jssUser:$jssPass" -d "<user><managed_apple_id>$managedAppleID</managed_apple_id></user>" "$jssURL/JSSResource/users/id/$id"
((count--))
fi
done
exit 0
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 04-14-2020 07:46 AM
Something like this may help you?
#!/bin/bash
jssURL="https://xxxxx.jamfcloud.com"
## DO NOT EDIT BELOW THIS LINE ##
echo "Please enter your JSS username:"
read jssUser
echo "Please enter your JSS password:"
read -s jssPass
usersToGetAtOneTime="10000"
# Make sure xmlstarlet is installed
if ! command -v xmlstarlet > /dev/null ; then
echo "You must install xmlstarlet before using this script."
echo "Try "brew install xmlstarlet""
exit 1
fi
# Get list of users
echo "Downloading list of user IDs..."
while read -r line; do
ids+=("$line")
done <<< "$(curl -X GET -k -s -u "$jssUser:$jssPass" "$jssURL/JSSResource/users" | xmlstarlet sel -t -v '/users/user/id')"
# Find users with roster_managed_apple_id in
echo "Gathering User Information"
count="$usersToGetAtOneTime"
for id in "${ids[@]}"; do
managedAppleID=$(curl -X GET -k -s -u "$jssUser:$jssPass" "$jssURL/JSSResource/users/id/$id" | xmlstarlet sel -t -v '/user/roster_managed_apple_id')
# Update Managed Apple ID if Roster Managed Apple ID Exists
if [ -z "$managedAppleID" ]
then
echo "No Managed Apple ID, moving on"
else
echo "$managedAppleID"
curl -X PUT -H "Accept: application/xml" -H "Content-type: application/xml" -k -u "$jssUser:$jssPass" -d "<user><managed_apple_id>$managedAppleID</managed_apple_id></user>" "$jssURL/JSSResource/users/id/$id"
((count--))
fi
done
exit 0
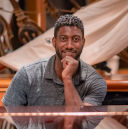
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 04-14-2020 10:15 AM
If you are move comfortable manipulating CSVs than scripting, you can check out The MUT. There is a good How To Video on their page
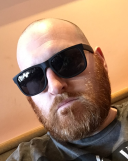
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 04-18-2020 04:14 AM
Thanks @chris.reynolds that worked a treat! Also thanks @shaquir, I will check that out!
