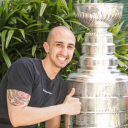
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 03-12-2012 09:52 AM
What is the best way to get the RealName of the current logged in user? I am writing an Extension attribute to call an AD group and if the AD group contains a match of the real name of the logged in user to that of the AD group then you receive a Yes or No output.
Solved! Go to Solution.
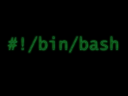
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 03-12-2012 11:18 AM
try mine; like this
#!/bin/bash
group="FNGMACAPPSTORE"
for username in $(stat /dev/console | awk '{print $5}'); do
if dscl /Search -read /Users/"${username}" memberOf | grep -q "${group}"; then
echo "<result>Yes</result>"
else
echo "<result>No</result>"
fi
done
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 03-12-2012 10:24 AM
something like the below should work:
loggedInUser=`/bin/ls -l /dev/console | /usr/bin/awk '{ print $3 }'`
userRealName=`dscl . -read /Users/$loggedInUser | grep RealName: | cut -c11-`
if [[ -z $userRealName ]]; then
userRealName=`dscl . -read /Users/$loggedInUser | awk '/^RealName:/,/^RecordName:/' | sed -n 2p | cut -c 2-`
fi
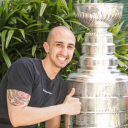
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 03-12-2012 10:28 AM
finger | egrep -m 1 -o '[ ][^ ][ ][^ ][ ]'
I got this to display what I want but is the best way?
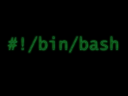
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 03-12-2012 10:54 AM
Here is the answer to the question that you asked but there is other information available to see what groups the person belongs too...
for username in $(stat /dev/console | awk '{print $5}'); do
dscl /Search -read /Users/"${username}" RealName | awk '{print $2}'
done
Put another way...
#!/bin/bash
group="Some Group"
for username in $(stat /dev/console | awk '{print $5}'); do
if dscl /Search -read /Users/"${username}" memberOf | grep -q "${group}"; then
echo "<result>Yes</result>"
else
echo "<result>No</result>"
fi
done
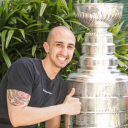
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 03-12-2012 11:00 AM
This is what I am using
#!/bin/bash
realName=$(finger | egrep -m 1 -o '[ ][^ ]+[ ][^ ]+[ ]')
dscl /Active Directory/FFE/All Domains -read /Groups/FNGMACAPPSTORE | grep "$realName"
if [ $? -eq 0 ]; then
result=Yes
else
result=No
fi
echo "<result>$result</result>"
It does not work, however if I replace $realName with "Matthew Lee" it does work. When I use the finger command it returns what I expect "Matthew Lee". I am assuming that my setting $realName with the finger command is the issue.
The above commands aren't giving me the output I need :(
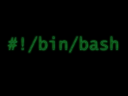
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 03-12-2012 11:18 AM
try mine; like this
#!/bin/bash
group="FNGMACAPPSTORE"
for username in $(stat /dev/console | awk '{print $5}'); do
if dscl /Search -read /Users/"${username}" memberOf | grep -q "${group}"; then
echo "<result>Yes</result>"
else
echo "<result>No</result>"
fi
done
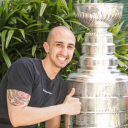
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 03-12-2012 11:23 AM
Seems to work so far! I am going to test it a bit and post the results! Thanks!
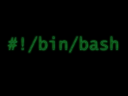
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 03-12-2012 11:25 AM
Np :)
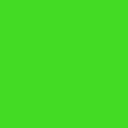
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 03-19-2012 10:36 AM
stat can just provide the username
stat -f%Su /dev/console
Be warned though. My experience is that the new Virtual Desktop ScreenSharing changes the ownership of /dev/console and so if you do this kind of screen sharing then you could end up with incorrect reports!
I have no idea if this is only the case on certain versions of 10.7, but I imagine that this wont change.
However, you will find:
who | grep console
macsupport console Mar 19 16:16
root console Mar 19 15:58
sean console Mar 19 11:58
I don't like this method. If you have a user called console (unlikely I know). You could choose to grab all of the console logins and then loop through them.
or
#ps aux | grep login
macsupport 24779 0.0 0.1 2626380 10728 ?? Ss 4:16PM 0:00.26 /System/Library/CoreServices/loginwindow.app/Contents/MacOS/loginwindow
root 24063 0.0 0.0 2440216 2880 s000 Ss 4:08PM 0:00.04 login -pf sean
root 20190 0.0 0.1 2642104 11312 ?? Ss 3:58PM 0:00.37 /System/Library/CoreServices/loginwindow.app/Contents/MacOS/loginwindow
root 316 0.0 0.0 2518496 2368 ?? Ss 11:57AM 0:00.28 /System/Library/CoreServices/logind
sean 69 0.0 0.7 2733900 55024 ?? Ss 11:57AM 0:05.57 /System/Library/CoreServices/loginwindow.app/Contents/MacOS/loginwindow console
root 28655 0.0 0.0 2434892 504 s002 S+ 4:45PM 0:00.00 grep login
You could grep for MacOS/loginwindow and again loop through them or if you only want the actual logged in user, use the ps command to grep for either 'login -pf' and take the last field or 'loginwindow console' and take first field. There are a bunch of ways to approach this and improve, but this gives some ideas.
Alternatively, learn objective C.
Either way, I imagine there are an awful lot of people that are about to be rewriting a lot of scripts; something to add to the task list!
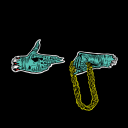
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 03-19-2012 11:23 AM
Sean,
Good catch on the virtual desktop screen sharing. I have not currently tested any of this so I cannot confirm or deny. Another method I can give for you all to test is reading the info form the loginwindow.plist file.
see example:
bash-3.2$ defaults read /Library/Preferences/com.apple.loginwindow lastUserName
admin
bash-3.2$
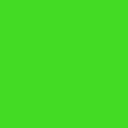
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 03-19-2012 04:18 PM
Sorry, but this plist doesn't update with fast user switching, one of the reasons for using the owner of console. I haven't tested this yet with the output of 'ps'. Mind you, I may not have tested the update of the plist with Lion!!!
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 03-10-2013 09:58 PM
tlarkin,
Mac OS update 'lastUserName' with last logged in user name. In case of multiple user log-in at the same time, last user name will be the one who logged in at last. So that terminal command 'defaults read /Library/Preferences/com.apple.loginwindow lastUserName' will return last logged in user name. That means we can not say it return current logged in user. Yes in case of single user log in it gives proper result.
For getting current user name, we can use '$(stat /dev/console | awk '{print $5}')'.
Thank you.
