Script to Install & Update Zoom
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on
09-28-2018
09:12 AM
- last edited on
03-04-2025
08:30 AM
by
kh-richa_mig
We use Zoom a lot, so I modified a script that we were using for Firefox (Thanks Joe Farage!) to install or update to the newest version of Zoom. This one enables HD video, sets SSO logins to be default, and configures the URL for SSO. Below is the script:
#!/bin/sh
#####################################################################################################
#
# ABOUT THIS PROGRAM
#
# NAME
# ZoomInstall.sh -- Installs or updates Zoom
#
# SYNOPSIS
# sudo ZoomInstall.sh
#
####################################################################################################
#
# HISTORY
#
# Version: 1.0
#
# - Shannon Johnson, 28.9.2018
# (Adapted from the FirefoxInstall.sh script by Joe Farage, 18.03.2015)
#
####################################################################################################
# Script to download and install Zoom.
# Only works on Intel systems.
#
# Set preferences - set to anything besides "true" to disable
hdvideo="true"
ssodefault="true"
ssohost="psu.zoom.us"
# choose language (en-US, fr, de)
lang=""
# CHECK TO SEE IF A VALUE WAS PASSED IN PARAMETER 1 AND, IF SO, ASSIGN TO "lang"
if [ "$4" != "" ] && [ "$lang" == "" ]; then
lang=$4
else
lang="en-US"
fi
pkgfile="ZoomInstallerIT.pkg"
plistfile="us.zoom.config.plist"
logfile="/Library/Logs/ZoomInstallScript.log"
# Are we running on Intel?
if [ '`/usr/bin/uname -p`'="i386" -o '`/usr/bin/uname -p`'="x86_64" ]; then
## Get OS version and adjust for use with the URL string
OSvers_URL=$( sw_vers -productVersion | sed 's/[.]/_/g' )
## Set the User Agent string for use with curl
userAgent="Mozilla/5.0 (Macintosh; Intel Mac OS X ${OSvers_URL}) AppleWebKit/535.6.2 (KHTML, like Gecko) Version/5.2 Safari/535.6.2"
# Get the latest version of Reader available from Zoom page.
latestver=`/usr/bin/curl -s -A "$userAgent" https://zoom.us/download | grep 'ZoomInstallerIT.pkg' | awk -F'/' '{print $3}'`
echo "Latest Version is: $latestver"
# Get the version number of the currently-installed Zoom, if any.
if [ -e "/Applications/zoom.us.app" ]; then
currentinstalledver=`/usr/bin/defaults read /Applications/zoom.us.app/Contents/Info CFBundleShortVersionString`
echo "Current installed version is: $currentinstalledver"
if [ ${latestver} = ${currentinstalledver} ]; then
echo "Zoom is current. Exiting"
exit 0
fi
else
currentinstalledver="none"
echo "Zoom is not installed"
fi
url="https://zoom.us/client/${latestver}/ZoomInstallerIT.pkg"
echo "Latest version of the URL is: $url"
echo "`date`: Download URL: $url" >> ${logfile}
# Compare the two versions, if they are different or Zoom is not present then download and install the new version.
if [ "${currentinstalledver}" != "${latestver}" ]; then
# Construct the plist file for preferences
echo "<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE plist PUBLIC "-//Apple//DTD PLIST 1.0//EN" "http://www.apple.com/DTDs/PropertyList-1.0.dtd">
<plist version="1.0">
<dict>
<key>nogoogle</key>
<string>1</string>
<key>nofacebook</key>
<string>1</string>
<key>ZDisableVideo</key>
<true/>
<key>ZAutoJoinVoip</key>
<true/>
<key>ZDualMonitorOn</key>
<true/>" >> /tmp/${plistfile}
if [ "${ssohost}" != "" ]; then
echo "
<key>ZAutoSSOLogin</key>
<true/>
<key>ZSSOHost</key>
<string>$ssohost</string>" >> /tmp/${plistfile}
fi
echo "<key>ZAutoFullScreenWhenViewShare</key>
<true/>
<key>ZAutoFitWhenViewShare</key>
<true/>" >> /tmp/${plistfile}
if [ "${hdvideo}" == "true" ]; then
echo "<key>ZUse720PByDefault</key>
<true/>" >> /tmp/${plistfile}
else
echo "<key>ZUse720PByDefault</key>
<false/>" >> /tmp/${plistfile}
fi
echo "<key>ZRemoteControlAllApp</key>
<true/>
</dict>
</plist>" >> /tmp/${plistfile}
# Download and install new version
/bin/echo "`date`: Current Zoom version: ${currentinstalledver}" >> ${logfile}
/bin/echo "`date`: Available Zoom version: ${latestver}" >> ${logfile}
/bin/echo "`date`: Downloading newer version." >> ${logfile}
/usr/bin/curl -L -o /tmp/${pkgfile} ${url}
/bin/echo "`date`: Installing PKG..." >> ${logfile}
/usr/sbin/installer -allowUntrusted -pkg /tmp/${pkgfile} -target /
/bin/sleep 10
/bin/echo "`date`: Deleting downloaded PKG." >> ${logfile}
/bin/rm /tmp/${pkgfile}
#double check to see if the new version got updated
newlyinstalledver=`/usr/bin/defaults read /Applications/zoom.us.app/Contents/Info CFBundleShortVersionString`
if [ "${latestver}" = "${newlyinstalledver}" ]; then
/bin/echo "`date`: SUCCESS: Zoom has been updated to version ${newlyinstalledver}" >> ${logfile}
# /Library/Application Support/JAMF/bin/jamfHelper.app/Contents/MacOS/jamfHelper -windowType hud -title "Zoom Installed" -description "Zoom has been updated." &
else
/bin/echo "`date`: ERROR: Zoom update unsuccessful, version remains at ${currentinstalledver}." >> ${logfile}
/bin/echo "--" >> ${logfile}
exit 1
fi
# If Zoom is up to date already, just log it and exit.
else
/bin/echo "`date`: Zoom is already up to date, running ${currentinstalledver}." >> ${logfile}
/bin/echo "--" >> ${logfile}
fi
else
/bin/echo "`date`: ERROR: This script is for Intel Macs only." >> ${logfile}
fi
exit 0
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 03-11-2020 11:49 AM
@GregE use this instead
currentinstalledver='/usr/bin/defaults read /Applications/zoom.us.app/Contents/Info CFBundleVersion'
this will give you the correct value
Current version as of 3/10/20 is 4.6.18176.0301 (what the script calls for along with the download link) - technically 4.6.7.18176.0301 is whats installed, so its missing the .7.
@omatsei im curious about one thing, maybe its just difficult for me to understand.
you have all the settings go to a temp PLIST location but i dont see what its called for or written elsewhere.
</plist>" >> /tmp/${plistfile}
how does that write to or even overwrite the current/nonexistent us.zoom.config.plist located in /Library/Preferences/us.zoom.config.plist?
currently im doing the slowpoke version of deploying via composer > JSS to ensure things go as expected.
i am updating and testing your script, but would it not be more prudent to write directly to the file location, the information?
eg
#!/bin/sh
zoomPLIST='/Library/Preferences/us.zoom.config.plist'
<key>ZAutoSSOLogin</key>
<string>https://example.zoom.us</string>
</dict>
</plist>" >${zoomPLIST}
technically, i would do a
#!/bin/sh
zoomPLIST='/Library/Preferences/us.zoom.config.plist'
/bin/cat <<'EOF' >$zoomPLIST
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE plist PUBLIC "-//Apple//DTD PLIST 1.0//EN" "http://www.apple.com/DTDs/PropertyList-1.0.dtd">
<plist version="1.0">
<dict>
<key>ZRemoteControlAllApp</key>
<true/>
<key>ConfirmWhenLeave</key>
<true/>
EOF
unless i am missing something with the where the PLIST data goes, cuz thats the intriguing part.
you can add some error checking in there, for example, if your last addition to the PLIST was
<key>ZAutoFitWhenViewShare</key>
<true/>
then you can grep that in an if/then statement to the write.
Can you clarify the plist part?
thanks!
EDIT:
we can ignore that, but still wondering if cat makes sense also
from ZOOM doc:
Once the .plist file is complete, it will need to be named us.zoom.config.plist. When deploying, as long as this file is in the same folder as the ZoomInstallerIT.pkg, the installation will automatically put the .plist file into the /Library/Preferences folder.
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 03-11-2020 04:55 PM
Thanks @GregE, I made the changes you suggested. The script still works when executed manually from a file, but fails when Jamf executes the script as a policy. I get "Policy error code: 2100" when I verbose the policy using "jamf policy -event"
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 03-17-2020 08:06 AM
@stephen.watson i think you missed my post. it gives you the answer you need :)
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 03-19-2020 12:05 AM
Apologies @beeboo, I thanked the wrong user in my last post. It's still not working after applying your change :(
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 03-20-2020 10:42 AM
@stephen.watson can you post the part where you think its broken, or even the whole part?
for reference, here are some changes i made
#!/bin/sh
installedVERS=$(/usr/bin/defaults read /Applications/zoom.us.app/Contents/Info CFBundleVersion)
is yours in quotes or a variable like that?
this should replace my previous line of current version
let me know if that works for you
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 03-23-2020 08:52 AM
I have tried the script update 9/27/2019 by omatsei, and the updates posted 10/22/2019 by kbingham, the script looks good, but I am getting syntax errors: Script result: /Library/Application Support/JAMF/tmp/Zoom Update: line 153: unexpected EOF while looking for matching ``'
/Library/Application Support/JAMF/tmp/Zoom Update: line 157: syntax error: unexpected end of file
Any thoughts on this? I combed through the script and am not finding much to shed light on this. Any help is greatly appreciated.
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 03-25-2020 08:41 PM
Post a screenshot @CaptainCarl but I'll place my bet that you have/don't have a space in the correct spot.
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 03-26-2020 05:54 AM
Sounds to me as if a closing quote sign is missing somewhere. Multi-line echo commands are not really to my liking.
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 04-01-2020 11:29 PM
Finally found my failure point. 10 seconds wasn't enough time for some Macs to install Zoom before it deleted the install package. Changing it to 20s resolved the random failures.
/usr/sbin/installer -allowUntrusted -pkg /tmp/${pkgfile} -target /
/bin/sleep 20
/bin/echo "`date`: Deleting downloaded PKG." >> ${logfile}
/bin/rm /tmp/${pkgfile}
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 04-02-2020 01:44 PM
Can anyone post a perfected version of this script and your guidelines for deploying it? We would use it, but I could not retroactively install any/all of the modifications mentioned in this thread myself ;-(
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 04-05-2020 07:07 PM
Added a few echo outs when troubleshooting the failures I was getting.
Policy 1:
Recurring check-in, Script (below), Smart Group (Application Title does not have zoom.us.app)
Policy 2:
Login, run once per week, Script (same one), Smart Group (Application Title has zoom.us.app).
* You'll need to modify: sso host
#!/bin/sh
#####################################################################################################
#
# ABOUT THIS PROGRAM
#
# NAME
# ZoomInstall.sh -- Installs or updates Zoom
#
# SYNOPSIS
# sudo ZoomInstall.sh
#
####################################################################################################
#
# HISTORY
#
# Version: 1.1
#
# 1.1 - Shannon Johnson, 27.9.2019
# Updated for new zoom numbering scheme
# Fixed the repeated plist modifications
#
# 1.0 - Shannon Johnson, 28.9.2018
# (Adapted from the FirefoxInstall.sh script by Joe Farage, 18.03.2015)
#
####################################################################################################
# Script to download and install Zoom.
# Only works on Intel systems.
#
# Set preferences
hdvideo="true"
ssodefault="true"
ssohost="xxx.zoom.us"
# choose language (en-US, fr, de)
lang=""
# CHECK TO SEE IF A VALUE WAS PASSED IN PARAMETER 1 AND, IF SO, ASSIGN TO "lang"
if [ "$4" != "" ] && [ "$lang" == "" ]; then
lang=$4
else
lang="en-US"
fi
pkgfile="ZoomInstallerIT.pkg"
plistfile="us.zoom.config.plist"
logfile="/Library/Logs/ZoomInstallScript.log"
# Are we running on Intel?
if [ '`/usr/bin/uname -p`'="i386" -o '`/usr/bin/uname -p`'="x86_64" ]; then
## Get OS version and adjust for use with the URL string
OSvers_URL=$( sw_vers -productVersion | sed 's/[.]/_/g' )
## Set the User Agent string for use with curl
userAgent="Mozilla/5.0 (Macintosh; Intel Mac OS X ${OSvers_URL}) AppleWebKit/535.6.2 (KHTML, like Gecko) Version/5.2 Safari/535.6.2"
# Get the latest version of Reader available from Zoom page.
latestver=$(/usr/bin/curl -s -A "$userAgent" https://zoom.us/download | grep 'ZoomInstallerIT.pkg' | awk -F'/' '{print $3}')
echo "Latest Version is: $latestver"
# Get the version number of the currently-installed Zoom, if any.
if [ -e "/Applications/zoom.us.app" ]; then
currentinstalledver=$(/usr/bin/defaults read /Applications/zoom.us.app/Contents/Info CFBundleVersion)
echo "Current installed version is: $currentinstalledver"
if [ ${latestver} = ${currentinstalledver} ]; then
echo "Zoom is current. Exiting"
exit 0
fi
else
currentinstalledver="none"
echo "Zoom is not installed"
fi
url="https://zoom.us/client/${latestver}/ZoomInstallerIT.pkg"
echo "Latest version of the URL is: $url"
echo "`date`: Download URL: $url" >> ${logfile}
# Compare the two versions, if they are different or Zoom is not present then download and install the new version.
if [ "${currentinstalledver}" != "${latestver}" ]; then
# Construct the plist file for preferences
echo "<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE plist PUBLIC "-//Apple//DTD PLIST 1.0//EN" "http://www.apple.com/DTDs/PropertyList-1.0.dtd">
<plist version="1.0">
<dict>
<key>nogoogle</key>
<string>1</string>
<key>nofacebook</key>
<string>1</string>
<key>ZDisableVideo</key>
<true/>
<key>ZAutoJoinVoip</key>
<true/>
<key>ZDualMonitorOn</key>
<true/>" > /tmp/${plistfile}
if [ "${ssohost}" != "" ]; then
echo "
<key>ZAutoSSOLogin</key>
<true/>
<key>ZSSOHost</key>
<string>$ssohost</string>" >> /tmp/${plistfile}
fi
echo "<key>ZAutoFullScreenWhenViewShare</key>
<true/>
<key>ZAutoFitWhenViewShare</key>
<true/>" >> /tmp/${plistfile}
if [ "${hdvideo}" == "true" ]; then
echo "<key>ZUse720PByDefault</key>
<true/>" >> /tmp/${plistfile}
else
echo "<key>ZUse720PByDefault</key>
<false/>" >> /tmp/${plistfile}
fi
echo "<key>ZRemoteControlAllApp</key>
<true/>
</dict>
</plist>" >> /tmp/${plistfile}
# Download and install new version
/bin/echo "`date`: Current Zoom version: ${currentinstalledver}" >> ${logfile}
/bin/echo "`date`: Available Zoom version: ${latestver}" >> ${logfile}
/bin/echo "`date`: Downloading newer version." >> ${logfile}
/usr/bin/curl -sLo /tmp/${pkgfile} ${url}
/bin/echo "`date`: Installing PKG..." >> ${logfile}
/usr/sbin/installer -allowUntrusted -pkg /tmp/${pkgfile} -target /
/bin/sleep 20
/bin/echo "`date`: Deleting downloaded PKG." >> ${logfile}
/bin/rm /tmp/${pkgfile}
#double check to see if the new version got updated
newlyinstalledver=$(/usr/bin/defaults read /Applications/zoom.us.app/Contents/Info CFBundleVersion)
echo "Version on Mac is ${newlyinstalledver}"
if [ "${latestver}" = "${newlyinstalledver}" ]; then
/bin/echo "`date`: SUCCESS: Zoom has been updated to version ${newlyinstalledver}" >> ${logfile}
echo "Zoom updated successfully"
# /Library/Application Support/JAMF/bin/jamfHelper.app/Contents/MacOS/jamfHelper -windowType hud -title "Zoom Installed" -description "Zoom has been updated." &
else
/bin/echo "`date`: ERROR: Zoom update unsuccessful, version remains at ${currentinstalledver}." >> ${logfile}
/bin/echo "--" >> ${logfile}
echo "Zoom not updated - Installation error"
exit 1
fi
# If Zoom is up to date already, just log it and exit.
else
/bin/echo "`date`: Zoom is already up to date, running ${currentinstalledver}." >> ${logfile}
/bin/echo "--" >> ${logfile}
fi
else
/bin/echo "`date`: ERROR: This script is for Intel Macs only." >> ${logfile}
fi
exit 0
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 04-06-2020 12:07 AM
I have copied the latest script (above) but I still get Policy Error Code 2100 when running "jamf policy -event zoom -verbose"
ok, script now running fine. I found this support thread regarding a '/' in the policy title.
https://www.jamf.com/jamf-nation/discussions/20342/script-runs-fine-on-machine-fails-as-a-policy
Thank again for your help :)
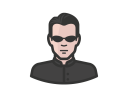
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 04-08-2020 12:37 PM
What can I do if we do not use a domain and are using the free upgrade due to COVID-19? This script forces a domain?
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 04-08-2020 05:23 PM
you can remove the line item for domain
<key>ZAutoSSOLogin</key> <true/> <key>ZSSOHost</key> <string>$ssohost</string>"
can be removed to be suited to your needs
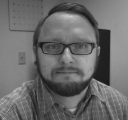
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 04-09-2020 05:38 PM
The script from 4-5-2020 runs and completes for me.
I do have one problem. the .plist file is not being updated and I'm unable to locate it.
Ideas?
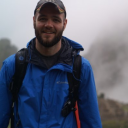
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 04-10-2020 04:41 AM
@atomczynski its located at /Library/Preferences/us.zoom.config.plist.
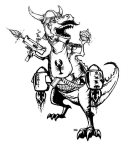
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 04-14-2020 12:58 PM
Here's what we do for ours. It's a multi pronged approach that several pieces need to be in place before updated.
We have an extension attribute that runs on the endpoint and compares what the current shipping version of Zoom vs what the user has installed. If it's out of date it gets the attribute 'Old'. If it's the current version, it is 'Latest'.
We have a smart group that is scoped to that extension attribute and only add 'Old' devices.
Once a day a policy runs against machines that are in the 'Old' smart group. The script within the policy pulls the latest IT installer version from Zoom and installs it to their computer.
This is the installer script for the policy.
#!/bin/bash
# This script runs within a policy is scoped to devices listed as 'Old' in the Extension Attribute.
OSvers_URL=$( sw_vers -productVersion | sed 's/[.]/_/g' )
userAgent="Mozilla/5.0 (Macintosh; Intel Mac OS X ${OSvers_URL}) AppleWebKit/535.6.2 (KHTML, like Gecko) Version/5.2 Safari/535.6.2"
# Get the latest version of Reader available from Zoom page.
latestver=`/usr/bin/curl -s -A "$userAgent" https://zoom.us/download | grep 'ZoomInstallerIT.pkg' | awk -F'/' '{print $3}'`
echo "Installing version ${latestver}";
url="https://zoom.us/client/${latestver}/ZoomInstallerIT.pkg"
# Construct the plist file for preferences
echo "<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE plist PUBLIC "-//Apple//DTD PLIST 1.0//EN" "http://www.apple.com/DTDs/PropertyList-1.0.dtd">
<plist version="1.0">
<dict>
<key>nogoogle</key>
<true/>
<key>nofacebook</key>
<true/>
<key>disableloginwithemail</key>
<true/>
<key>LastLoginType</key>
<true/>
<key>ZAutoUpdate</key>
<true/>
<key>ZAutoJoinVoip</key>
<true/>
<key>ZAutoSSOLogin</key>
<true/>
<key>ZSSOHost</key>
<string>pretendco.zoom.us</string>
<key>ZRemoteControlAllApp</key>
<true/>
</dict>
</plist>" >> /var/tmp/us.zoom.config.plist;
/usr/bin/curl -L -o /var/tmp/ZoomInstallerIT.pkg ${url};
/usr/sbin/installer -pkg /var/tmp/ZoomInstallerIT.pkg -target /;
This is the extension attribute to label the device as 'Old' or 'Latest'
#!/bin/bash
# This script is used as an extension attribute and compares
# the IT installer version on the Zoom site vs what the user is running
if [ ! -d "/Applications/zoom.us.app" ]; then
echo "<result>Not Installed</result>"
exit 0
else
# Determine current OS version to pass to user string
OSvers_URL=$( sw_vers -productVersion | sed 's/[.]/_/g' )
# Create useragent to pass into curl command
userAgent="Mozilla/5.0 (Macintosh; Intel Mac OS X ${OSvers_URL}) AppleWebKit/535.6.2 (KHTML, like Gecko) Version/5.2 Safari/535.6.2"
# Pull current available version of Zoom from their site
latestver=`/usr/bin/curl -s -A "$userAgent" https://zoom.us/download | grep 'ZoomInstallerIT.pkg' | awk -F'/' '{print $3}'`
# Get current version installed on computer
installedVersion=$( defaults read /Applications/zoom.us.app/Contents/Info.plist CFBundleVersion )
# Compare zoom version vs installed version, print result
if [ "$latestver" == "$installedVersion" ]; then
echo "<result>Latest</result>"
exit 0
else
echo "<result>Old</result>"
exit 0
fi
fi
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 04-14-2020 01:47 PM
This is great, thanks for sharing, @dmarcnw ! Has anyone created something similar or recommend a way to modify this EA to show the current installed version? This would be more for reporting than scoping.
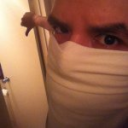
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 04-14-2020 02:06 PM
Hey @JMenacker
I modified the EA by @dmarcnw so it will display the version of Zoom installed for you:
#!/bin/bash
# This script is used as an extension attribute and displays
# the current version of Zoom installed on a Mac
if [ ! -d "/Applications/zoom.us.app" ]; then
echo "<result>Not Installed</result>"
exit 0
else
# Get current version installed on Mac
installedVersion=$( defaults read /Applications/zoom.us.app/Contents/Info.plist CFBundleVersion )
# Display current version installed on Mac
echo "Current Zoom version is: $installedVersion"
exit 0
fi

- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 04-15-2020 11:50 PM
Hi, thank you and this is brilliant...
However, i wish to simply push the us.zoom.config.plist to the /Library/Preferences/ directory, for existing Zoom installations. Doing this does NOT change the Zoom client config. Should this be possible, or does it REQUIRE a full reinstall with the plist file alongside with the pkg?

- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 04-16-2020 05:09 AM
@ajhstn, you may find this useful. It’s a manifest to help you set Zoom settings using a configuration profile.

- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 04-16-2020 05:14 PM
I want to answer my own question i posted here, it might be useful to someone.
However, i wish to simply push the us.zoom.config.plist to the /Library/Preferences/ directory, for existing Zoom installations. Doing this does NOT change the Zoom client config. Should this be possible, or does it REQUIRE a full reinstall with the plist file alongside with the pkg?
The answer is;
-- https://zoom.us/client/latest/Zoom.pkg does not recognise /Library/Managed Preferences/ or /Library/Preferences
-- https://zoom.us/client/latest/ZoomInstallerIT.pkg does recognise both paths above.
So if you want to just push a config to an existing Zoom client, you must make sure that Zoom was installed using the ZoomInstallerIT.pkg package, not Zoom.pkg.
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 04-16-2020 07:06 PM
@ajhstn, a zillion thanks for this tip. I literally spent most than half the day trying to figure out why my plists would not stick. The funny thing was that some things like auto sso login were loading and some were not and so I was convinced that I entering the plist wrong. I also found out the Zoom client was also storing some settings in ~/Library/Application Support/zoom.us/data/zoomus.db -- really fustrating!
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 04-23-2020 11:52 AM
@talkingmoose that manifest worked really well for me. thank you!

- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 04-23-2020 12:07 PM
[~mmark - childrensnational], thanks for feedback! Glad to hear it's working.
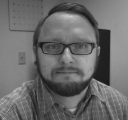
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 04-30-2020 03:04 PM
Today I see some failures of install.
Script result: Latest Version is: 5.0.23508.0430
Current installed version is: 4.6.20561.0413
Latest version of the URL is: https://zoom.us/client/5.0.23508.0430/ZoomInstallerIT.pkg
installer: Package name is Zoom
installer: Upgrading at base path /
installer: The upgrade was successful.
Version on Mac is 4.6.20561.0413
Zoom not updated - Installation error
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 04-30-2020 05:02 PM
I am also getting that but suspect it may be due to Zoom running at the time it's trying to upgrade? I have mine set to run at login so it shouldn't be that reason, but there's no consistency to the Macs that fail (OS versions).
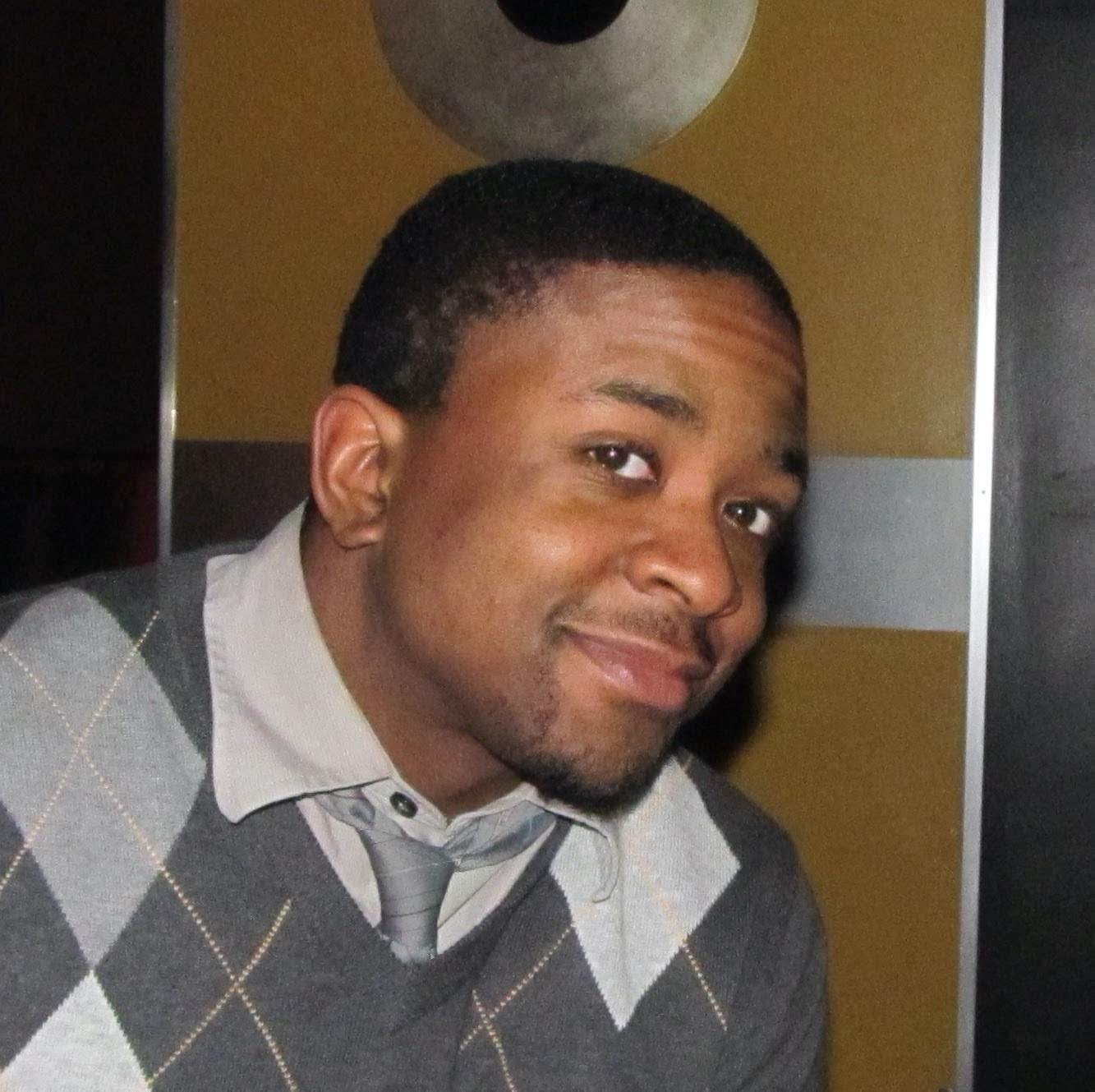
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 05-01-2020 07:47 AM
I see that error too and you are correct that this is because Zoom is running. I will try some more logic with buttons but for now I added to the jamf helper notification in the Script to notify that the end user will need to quit zoom and reopen for the update to take effect.
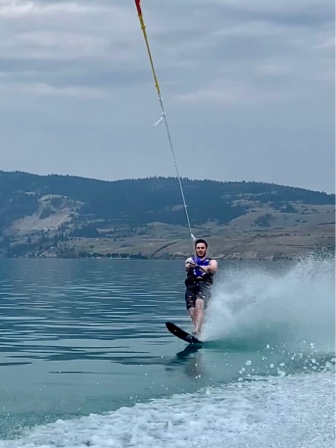
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 05-01-2020 09:56 AM
Out of curiosity, is including the .plist with the .pkg better practice compared to utilizing a configuration profile and the Custom Settings payload?
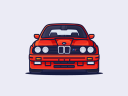
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 05-15-2020 06:37 AM
Is anyone having problems with this line? I am not returning any result for this...
EDIT: Nvm, I forgot to include the variables inside the command. Disregard.
#!/bin/sh
latestver=`/usr/bin/curl -s -A "$userAgent" https://zoom.us/download | grep 'ZoomInstallerIT.pkg' | awk -F'/' '{print $3}'`
echo "Latest Version is: $latestver"
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 05-20-2020 06:26 AM
@ajhstn What method did you use or how did you push the us.zoom.config.plist to the /Library/Preferences/ directory? I'm not having any luck with Composer?
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 05-20-2020 12:59 PM
https://www.jamf.com/jamf-nation/discussions/35399/zoom-update-is-requiring-admin-credentials-in-self-service#responseChild202203
answered you other thread, but you can just make the plist as you want then drop it into composer.
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 05-29-2020 02:45 PM
Do I actually have to have the *.pkg file in the local disk of the Computers in the Scope?
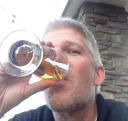
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 06-03-2020 01:21 PM
I would like to use the above script without the plist options. We don't use Zoom SSO or control how users connect. Can I just edit the plist section out of the script?
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 06-16-2020 09:32 AM
@erichughes yes ofc, just deploy the zoomIT pkg if thats all you desire.
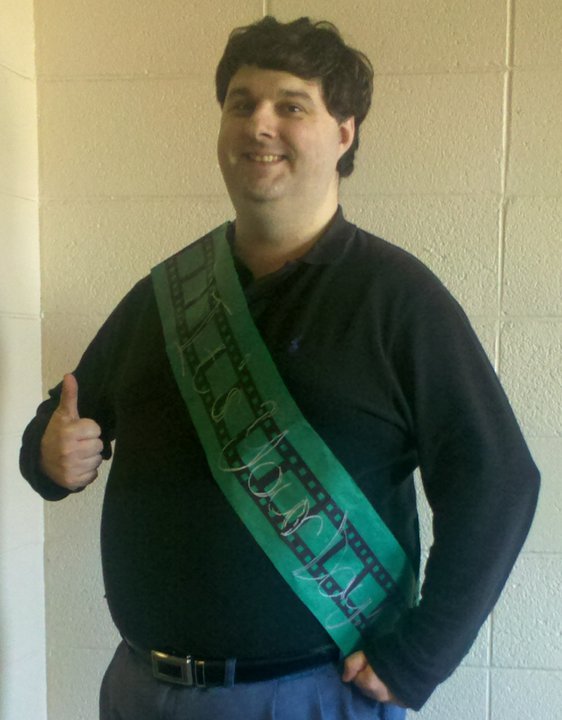
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 07-03-2020 10:31 AM
How do I modify the script to allow Google instead of an SSO?
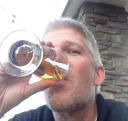
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 07-07-2020 10:48 AM
@beeboo That is what we do now. Gets old uploading a new package every week. Need to get an AutoPKG workflow set up at some point.
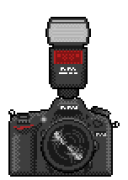
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 08-18-2020 11:26 PM
My brain is not working right tonight. We don’t use SSO on our campus for Zoom, I’m not figuring out how to make this script work to install Zoom and update Zoom without needing admin credentials. How should the script look?
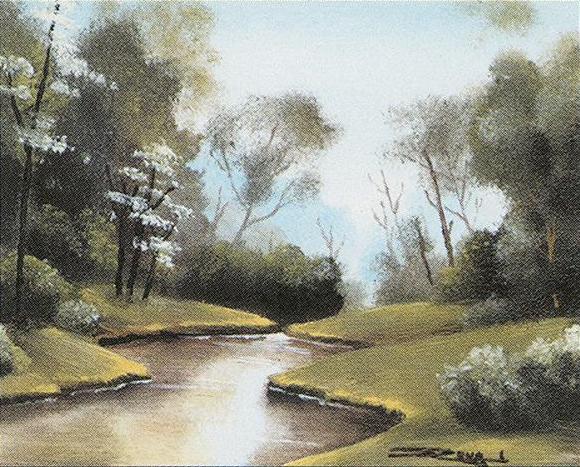
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 08-19-2020 06:01 AM
This is what I use. We don't manage zooms preferences or settings at all. Just want the people who are using it to have the newest version.
#!/bin/bash
#https://www.jamf.com/jamf-nation/third-party-products/files/1051/install-latest-zoom-client
# this is the full URL
url="https://zoom.us/client/latest/ZoomInstallerIT.pkg"
# change directory to /private/tmp to make this the working directory
cd /private/tmp/
# download the installer package and name it for the linkID
/usr/bin/curl -JL "$url" -o "ZoomInstallerIT.pkg"
# install the package
/usr/sbin/installer -pkg "ZoomInstallerIT.pkg" -target /
# remove the installer package when done
/bin/rm -f "ZoomInstallerIT.pkg"
exit 0
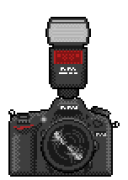
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 08-19-2020 08:48 AM
@strayer Thank you for this. This won't prompt for admin credentials?
