Citrix Receiver update script

- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 05-10-2016 06:30 AM
Script to download the latest Citrix Receiver for Mac:
#!/bin/bash
#####################################################################################################
#
# ABOUT THIS PROGRAM
#
# NAME
# CitrixReceiverUpdate.sh -- Updates Citrix Receiver
#
# SYNOPSIS
# sudo CitrixReceiverUpdate.sh
#
# LICENSE
# Distributed under the MIT License
#
# EXIT CODES
# 0 - Citrix Receiver is current
# 1 - Citrix Receiver installed successfully
# 2 - Citrix Receiver NOT installed
# 3 - Citrix Receiver update unsuccessful
# 4 - Citrix Receiver is running or was attempted to be installed manually and user deferred install
# 5 - Not an Intel-based Mac
# 6 - ERROR: Wireless connected to a known bad WiFi network that won't allow downloading of the installer
#
####################################################################################################
#
# HISTORY
#
# Version: 1.0
#
# - v.1.0 Luis Lugo, 09.05.2016 : Updates Citrix Receiver
#
####################################################################################################
# Script to download and install Citrix Receiver.
# Setting variables
receiverProcRunning=0
# Echo function
echoFunc () {
# Date and Time function for the log file
fDateTime () { echo $(date +"%a %b %d %T"); }
# Title for beginning of line in log file
Title="InstallLatestCitrixReceiver:"
# Header string function
fHeader () { echo $(fDateTime) $(hostname) $Title; }
# Check for the log file
if [ -e "/Library/Logs/CitrixReceiverUpdateScript.log" ]; then
echo $(fHeader) "$1" >> "/Library/Logs/CitrixReceiverUpdateScript.log"
else
cat > "/Library/Logs/CitrixReceiverUpdateScript.log"
if [ -e "/Library/Logs/CitrixReceiverUpdateScript.log" ]; then
echo $(fHeader) "$1" >> "/Library/Logs/CitrixReceiverUpdateScript.log"
else
echo "Failed to create log file, writing to JAMF log"
echo $(fHeader) "$1" >> "/var/log/jamf.log"
fi
fi
# Echo out
echo $(fDateTime) ": $1"
}
# Exit function
exitFunc () {
case $1 in
0) exitCode="0 - Citrix Receiver is current! Version: $2";;
1) exitCode="1 - SUCCESS: Citrix Receiver has been updated to version $2";;
2) exitCode="2 - ERROR: Citrix Receiver NOT installed!";;
3) exitCode="3 - ERROR: Citrix Receiver update unsuccessful, version remains at $2!";;
4) exitCode="4 - ERROR: Citrix Receiver is running or was attempted to be installed manually and user deferred install.";;
5) exitCode="5 - ERROR: Not an Intel-based Mac.";;
6) exitCode="6 - ERROR: Wireless connected to a known bad WiFi network that won't allow downloading of the installer! SSID: $2";;
*) exitCode="$1";;
esac
echoFunc "Exit code: $exitCode"
echoFunc "======================== Script Complete ========================"
exit $1
}
# Check to see if Citrix Receiver is running
receiverRunningCheck () {
processNum=$(ps aux | grep "Citrix Receiver" | wc -l)
if [ $processNum -gt 1 ]
then
# Receiver is running, prompt the user to close it or defer the upgrade
receiverRunning
fi
}
# If Citrix Receiver is running, prompt the user to close it
receiverRunning () {
echoFunc "Citrix Receiver appears to be running!"
hudTitle="Citrix Receiver Update"
hudDescription="Citrix Receiver needs to be updated. Please save your work and close the application to proceed. You can defer if needed.
If you have any questions, please call the help desk."
jamfHelperPrompt=`/Library/Application Support/JAMF/bin/jamfHelper.app/Contents/MacOS/jamfHelper -windowType hud -lockHUD -title "$hudTitle" -description "$hudDescription" -button1 "Proceed" -button2 "Defer" -defaultButton 1`
case $jamfHelperPrompt in
0)
echoFunc "Proceed selected"
receiverProcRunning=1
receiverRunningCheck
;;
2)
echoFunc "Deferment Selected"
exitFunc 4
;;
*)
echoFunc "Selection: $?"
#receiverProcRunning=1
#receiverRunningCheck
exitFunc 3 "Unknown"
;;
esac
}
# If Citrix Receiver is running, prompt the user to close it
receiverUpdateMan () {
echoFunc "Citrix Receiver appears to be running!"
hudTitle="Citrix Receiver Update"
hudDescription="Citrix Receiver needs to be updated. You will see a program downloading the installer. You can defer if needed.
If you have any questions, please call the help desk."
jamfHelperPrompt=`/Library/Application Support/JAMF/bin/jamfHelper.app/Contents/MacOS/jamfHelper -windowType hud -lockHUD -title "$hudTitle" -description "$hudDescription" -button1 "Defer" -button2 "Proceed" -defaultButton 1 -timeout 60 -countdown`
case $jamfHelperPrompt in
0)
echoFunc "Deferment Selected"
exitFunc 4
;;
2)
echoFunc "Proceed selected"
receiverRunningCheck
;;
*)
echoFunc "Selection: $?"
exitFunc 3 "Unknown"
;;
esac
}
echoFunc ""
echoFunc "======================== Starting Script ========================"
# Are we on a bad wireless network?
if [[ "$4" != "" ]]
then
wifiSSID=`/System/Library/PrivateFrameworks/Apple80211.framework/Versions/Current/Resources/airport -I | awk '/ SSID/ {print substr($0, index($0, $2))}'`
echoFunc "Current Wireless SSID: $wifiSSID"
badSSIDs=( $4 )
for (( i = 0; i < "${#badSSIDs[@]}"; i++ ))
do
if [[ "$wifiSSID" == "${badSSIDs[i]}" ]]
then
echoFunc "Connected to a WiFi network that blocks downloads!"
exitFunc 6 "${badSSIDs[i]}"
fi
done
fi
# Are we running on Intel?
if [ '`/usr/bin/uname -p`'="i386" -o '`/usr/bin/uname -p`'="x86_64" ]; then
## Get OS version and adjust for use with the URL string
OSvers_URL=$( sw_vers -productVersion | sed 's/[.]/_/g' )
## Set the User Agent string for use with curl
userAgent="Mozilla/5.0 (Macintosh; Intel Mac OS X ${OSvers_URL}) AppleWebKit/535.6.2 (KHTML, like Gecko) Version/5.2 Safari/535.6.2"
# Get the latest version of Receiver available from Citrix's Receiver page.
latestver=``
while [ -z "$latestver" ]
do
latestver=`curl -s -L https://www.citrix.com/downloads/citrix-receiver/mac/receiver-for-mac-latest.html | grep "<h1>Receiver " | awk '{print $2}'`
done
echoFunc "Latest Citrix Receiver Version is: $latestver"
latestvernorm=`echo ${latestver}`
# Get the version number of the currently-installed Citrix Receiver, if any.
if [ -e "/Applications/Citrix Receiver.app" ]; then
currentinstalledapp="Citrix Receiver"
currentinstalledver=`/usr/bin/defaults read /Applications/Citrix Receiver.app/Contents/Info CFBundleShortVersionString`
echoFunc "Current Receiver installed version is: $currentinstalledver"
if [ ${latestvernorm} = ${currentinstalledver} ]; then
exitFunc 0 "${currentinstalledapp} ${currentinstalledver}"
else
# Not running the latest version, check if Receiver is running
receiverRunningCheck
fi
else
currentinstalledapp="None"
currentinstalledver="N/A"
exitFunc 2
fi
# Build URL and dmg file name
CRCurrVersNormalized=$( echo $latestver | sed -e 's/[.]//g' )
echoFunc "CRCurrVersNormalized: $CRCurrVersNormalized"
url1="https:"
url2=`curl -s -L https://www.citrix.com/downloads/citrix-receiver/mac/receiver-for-mac-latest.html | grep "ctx-dl-link ie10-download-hide promptDw"" | awk '{print $8}' | cut -c 6-112`
url=`echo "${url1}${url2}"`
echoFunc "Latest version of the URL is: $url"
dmgfile="Citrix_Rec_${CRCurrVersNormalized}.dmg"
# Compare the two versions, if they are different or Citrix Receiver is not present then download and install the new version.
if [ "${currentinstalledver}" != "${latestvernorm}" ]; then
echoFunc "Current Receiver version: ${currentinstalledapp} ${currentinstalledver}"
echoFunc "Available Receiver version: ${latestver} => ${CRCurrVersNormalized}"
echoFunc "Downloading newer version."
curl -s -o /tmp/${dmgfile} ${url}
case $? in
0)
echoFunc "Checking if the file exists after downloading."
if [ -e "/tmp/${dmgfile}" ]; then
receiverFileSize=$(du -k "/tmp/${dmgfile}" | cut -f 1)
echoFunc "Downloaded File Size: $receiverFileSize kb"
else
echoFunc "File NOT downloaded!"
exitFunc 3 "${currentinstalledapp} ${currentinstalledver}"
fi
echoFunc "Checking if Receiver is running one last time before we install"
receiverRunningCheck
echoFunc "Mounting installer disk image."
hdiutil attach /tmp/${dmgfile} -nobrowse -quiet
echoFunc "Installing..."
installer -pkg /Volumes/Citrix Receiver/Install Citrix Receiver.pkg -target / > /dev/null
sleep 10
echoFunc "Unmounting installer disk image."
umount "/Volumes/Citrix Receiver"
sleep 10
echoFunc "Deleting disk image."
rm /tmp/${dmgfile}
#double check to see if the new version got update
if [ -e "/Applications/Citrix Receiver.app" ]; then
newlyinstalledver=`/usr/bin/defaults read /Applications/Citrix Receiver.app/Contents/Info CFBundleShortVersionString`
if [ "${latestvernorm}" = "${newlyinstalledver}" ]; then
echoFunc "SUCCESS: Citrix Receiver has been updated to version ${newlyinstalledver}, issuing JAMF recon command"
jamf recon
if [ $receiverProcRunning -eq 1 ];
then
/Library/Application Support/JAMF/bin/jamfHelper.app/Contents/MacOS/jamfHelper -windowType hud -lockHUD -title "Citrix Receiver Updated" -description "Citrix Receiver has been updated to version ${newlyinstalledver}." -button1 "OK" -defaultButton 1
fi
exitFunc 1 "${newlyinstalledver}"
else
exitFunc 3 "${currentinstalledapp} ${currentinstalledver}"
fi
else
exitFunc 3 "${currentinstalledapp} ${currentinstalledver}"
fi
;;
*)
echoFunc "Curl function failed on download! Error: $?. Review error codes here: https://curl.haxx.se/libcurl/c/libcurl-errors.html"
;;
esac
else
# If Citrix Receiver is up to date already, just log it and exit.
exitFunc 0 "${currentinstalledapp} ${currentinstalledver}"
fi
else
# This script is for Intel Macs only.
exitFunc 5
fi
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 05-10-2016 07:47 AM
Oddly enough I was thinking about this earlier. Thank you.
I'll try it out.

- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 10-18-2016 10:15 AM
Version 12.3.0 broke some things with the script. Mainly, it sees the version as "12.3" instead of "12.3.0", so I added a check around line 184 to check for this specific version, and add ".0" after. I also tidied up the download url to look for the string between the double quotes, instead of counting characters (line 211).
#!/bin/bash
#####################################################################################################
#
# ABOUT THIS PROGRAM
#
# NAME
# CitrixReceiverUpdate.sh -- Installs or updates Citrix Receiver
#
# SYNOPSIS
# sudo CitrixReceiverUpdate.sh
#
# LICENSE
# Distributed under the MIT License
#
# EXIT CODES
# 0 - Citrix Receiver is current
# 1 - Citrix Receiver installed successfully
# 2 - Citrix Receiver NOT installed
# 3 - Citrix Receiver update unsuccessful
# 4 - Citrix Receiver is running or was attempted to be installed manually and user deferred install
# 5 - Not an Intel-based Mac
#
####################################################################################################
#
# HISTORY
#
# Version: 1.0
#
# - v.1.0 Luie Lugo, 09.05.2016 : Updates Citrix Receiver
# - v.1.1 Luie Lugo, 10.18.2016 : Updated for v12.3, also cleaned up download URL handling
#
####################################################################################################
# Script to download and install Citrix Receiver.
# Setting variables
receiverProcRunning=0
# Echo function
echoFunc () {
# Date and Time function for the log file
fDateTime () { echo $(date +"%a %b %d %T"); }
# Title for beginning of line in log file
Title="InstallLatestCitrixReceiver:"
# Header string function
fHeader () { echo $(fDateTime) $(hostname) $Title; }
# Check for the log file
if [ -e "/Library/Logs/CitrixReceiverUpdateScript.log" ]; then
echo $(fHeader) "$1" >> "/Library/Logs/CitrixReceiverUpdateScript.log"
else
cat "" > "/Library/Logs/CitrixReceiverUpdateScript.log"
if [ -e "/Library/Logs/CitrixReceiverUpdateScript.log" ]; then
echo $(fHeader) "$1" >> "/Library/Logs/CitrixReceiverUpdateScript.log"
else
echo "Failed to create log file, writing to JAMF log"
echo $(fHeader) "$1" >> "/var/log/jamf.log"
fi
fi
# Echo out
echo $(fDateTime) ": $1"
}
# Exit function
exitFunc () {
case $1 in
0) exitCode="0 - Citrix Receiver is current! Version: $2";;
1) exitCode="1 - SUCCESS: Citrix Receiver has been updated to version $2";;
2) exitCode="2 - ERROR: Citrix Receiver NOT installed!";;
3) exitCode="3 - ERROR: Citrix Receiver update unsuccessful, version remains at $2!";;
4) exitCode="4 - ERROR: Citrix Receiver is running or was attempted to be installed manually and user deferred install.";;
5) exitCode="5 - ERROR: Not an Intel-based Mac.";;
6) exitCode="6 - ERROR: Wireless connected to a known bad WiFi network that won't allow downloading of the installer! SSID: $2";;
*) exitCode="$1";;
esac
echoFunc "Exit code: $exitCode"
echoFunc "======================== Script Complete ========================"
exit $1
}
# Check to see if Citrix Receiver is running
receiverRunningCheck () {
processNum=$(ps aux | grep "Citrix Receiver" | wc -l)
if [ $processNum -gt 1 ]
then
# Receiver is running, prompt the user to close it or defer the upgrade
receiverRunning
fi
}
# If Citrix Receiver is running, prompt the user to close it
receiverRunning () {
echoFunc "Citrix Receiver appears to be running!"
hudTitle="Citrix Receiver Update"
hudDescription="Citrix Receiver needs to be updated. Please save your work and close the application to proceed. You can defer if needed.
If you have any questions, please call SPOC at 1-888-905-9500."
#sudo -u $(ls -l /dev/console | awk '{print $3}') utility
jamfHelperPrompt=`/Library/Application Support/JAMF/bin/jamfHelper.app/Contents/MacOS/jamfHelper -windowType hud -lockHUD -title "$hudTitle" -description "$hudDescription" -button1 "Proceed" -button2 "Defer" -defaultButton 1`
case $jamfHelperPrompt in
0)
echoFunc "Proceed selected"
receiverProcRunning=1
receiverRunningCheck
;;
2)
echoFunc "Deferment Selected"
exitFunc 4
;;
*)
echoFunc "Selection: $?"
#receiverProcRunning=1
#receiverRunningCheck
exitFunc 3 "Unknown"
;;
esac
}
# If Citrix Receiver is running, prompt the user to close it
receiverUpdateMan () {
echoFunc "Citrix Receiver appears to be running!"
hudTitle="Citrix Receiver Update"
hudDescription="Citrix Receiver needs to be updated. You will see a program downloading the installer. You can defer if needed.
If you have any questions, please call SPOC at 1-888-905-9500."
jamfHelperPrompt=`/Library/Application Support/JAMF/bin/jamfHelper.app/Contents/MacOS/jamfHelper -windowType hud -lockHUD -title "$hudTitle" -description "$hudDescription" -button1 "Defer" -button2 "Proceed" -defaultButton 1 -timeout 60 -countdown`
case $jamfHelperPrompt in
0)
echoFunc "Deferment Selected"
exitFunc 4
;;
2)
echoFunc "Proceed selected"
receiverRunningCheck
;;
*)
echoFunc "Selection: $?"
exitFunc 3 "Unknown"
;;
esac
}
echoFunc ""
echoFunc "======================== Starting Script ========================"
# Are we on a bad wireless network?
if [[ "$4" != "" ]]
then
wifiSSID=`/System/Library/PrivateFrameworks/Apple80211.framework/Versions/Current/Resources/airport -I | awk '/ SSID/ {print substr($0, index($0, $2))}'`
echoFunc "Current Wireless SSID: $wifiSSID"
badSSIDs=( $4 )
for (( i = 0; i < "${#badSSIDs[@]}"; i++ ))
do
if [[ "$wifiSSID" == "${badSSIDs[i]}" ]]
then
echoFunc "Connected to a WiFi network that blocks downloads!"
exitFunc 6 "${badSSIDs[i]}"
fi
done
fi
# Are we running on Intel?
if [ '`/usr/bin/uname -p`'="i386" -o '`/usr/bin/uname -p`'="x86_64" ]; then
## Get OS version and adjust for use with the URL string
OSvers_URL=$( sw_vers -productVersion | sed 's/[.]/_/g' )
## Set the User Agent string for use with curl
userAgent="Mozilla/5.0 (Macintosh; Intel Mac OS X ${OSvers_URL}) AppleWebKit/535.6.2 (KHTML, like Gecko) Version/5.2 Safari/535.6.2"
# Get the latest version of Receiver available from Citrix's Receiver page.
latestver=``
while [ -z "$latestver" ]
do
latestver=`curl -s -L https://www.citrix.com/downloads/citrix-receiver/mac/receiver-for-mac-latest.html | grep "<h1>Receiver " | awk '{print $2}'`
done
if [ ${latestver} = "12.3" ]; then
latestver="12.3.0"
fi
echoFunc "Latest Citrix Receiver Version is: $latestver"
latestvernorm=`echo ${latestver}`
# Get the version number of the currently-installed Citrix Receiver, if any.
if [ -e "/Applications/Citrix Receiver.app" ]; then
currentinstalledapp="Citrix Receiver"
currentinstalledver=`/usr/bin/defaults read /Applications/Citrix Receiver.app/Contents/Info CFBundleShortVersionString`
echoFunc "Current Receiver installed version is: $currentinstalledver"
if [ ${latestvernorm} = ${currentinstalledver} ]; then
exitFunc 0 "${currentinstalledapp} ${currentinstalledver}"
else
# Not running the latest version, check if Receiver is running
receiverRunningCheck
fi
else
currentinstalledapp="None"
currentinstalledver="N/A"
exitFunc 2
fi
# Build URL and dmg file name
CRCurrVersNormalized=$( echo $latestver | sed -e 's/[.]//g' )
echoFunc "CRCurrVersNormalized: $CRCurrVersNormalized"
url1="https:"
url2=`curl -s -L https://www.citrix.com/downloads/citrix-receiver/mac/receiver-for-mac-latest.html | grep "ctx-dl-link ie10-download-hide promptDw"" | awk '{print $8}' | awk -F" '{ print $2 }'`
url=`echo "${url1}${url2}"`
echoFunc "Latest version of the URL is: $url"
dmgfile="Citrix_Rec_${CRCurrVersNormalized}.dmg"
# Compare the two versions, if they are different or Citrix Receiver is not present then download and install the new version.
if [ "${currentinstalledver}" != "${latestvernorm}" ]; then
echoFunc "Current Receiver version: ${currentinstalledapp} ${currentinstalledver}"
echoFunc "Available Receiver version: ${latestver} => ${CRCurrVersNormalized}"
echoFunc "Downloading newer version."
curl -s -o /tmp/${dmgfile} ${url}
case $? in
0)
echoFunc "Checking if the file exists after downloading."
if [ -e "/tmp/${dmgfile}" ]; then
receiverFileSize=$(du -k "/tmp/${dmgfile}" | cut -f 1)
echoFunc "Downloaded File Size: $receiverFileSize kb"
else
echoFunc "File NOT downloaded!"
exitFunc 3 "${currentinstalledapp} ${currentinstalledver}"
fi
echoFunc "Checking if Receiver is running one last time before we install"
receiverRunningCheck
echoFunc "Mounting installer disk image."
hdiutil attach /tmp/${dmgfile} -nobrowse -quiet
echoFunc "Installing..."
echo "$(date +"%a %b %d %T") Installing Citrix Receiver v$latestver" >> /var/log/CitrixReceiverInstall.log
installer -pkg "/Volumes/Citrix Receiver/Install Citrix Receiver.pkg" -target / >> /var/log/CitrixReceiverInstall.log # > /dev/null
sleep 10
echoFunc "Unmounting installer disk image."
umount "/Volumes/Citrix Receiver"
sleep 10
echoFunc "Deleting disk image."
rm /tmp/${dmgfile}
#double check to see if the new version got update
if [ -e "/Applications/Citrix Receiver.app" ]; then
newlyinstalledver=`/usr/bin/defaults read /Applications/Citrix Receiver.app/Contents/Info CFBundleShortVersionString`
if [ "${latestvernorm}" = "${newlyinstalledver}" ]; then
echoFunc "SUCCESS: Citrix Receiver has been updated to version ${newlyinstalledver}, issuing JAMF recon command"
jamf recon
if [ $receiverProcRunning -eq 1 ];
then
/Library/Application Support/JAMF/bin/jamfHelper.app/Contents/MacOS/jamfHelper -windowType hud -lockHUD -title "Citrix Receiver Updated" -description "Citrix Receiver has been updated to version ${newlyinstalledver}." -button1 "OK" -defaultButton 1
fi
exitFunc 1 "${newlyinstalledver}"
else
exitFunc 3 "${currentinstalledapp} ${currentinstalledver}"
fi
else
exitFunc 3 "${currentinstalledapp} ${currentinstalledver}"
fi
;;
*)
echoFunc "Curl function failed on download! Error: $?. Review error codes here: https://curl.haxx.se/libcurl/c/libcurl-errors.html"
;;
esac
else
# If Citrix Receiver is up to date already, just log it and exit.
exitFunc 0 "${currentinstalledapp} ${currentinstalledver}"
fi
else
# This script is for Intel Macs only.
exitFunc 5
fi
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 02-07-2017 12:10 PM
I can't get this script to work for me. It always thinks Citrix is in use and won't proceed. Even when the app isn't in use there is always something active from Citrix in the Activity Manager. I have played with the script and even tried removing the in use checks but it still won't install. Is anyone else using this or have something better?
I'm currently running Citrix Receiver 12.1.100 and need to update to 12.4. I would rather have the script kill Citrix on it's own instead of requesting the user to do something.
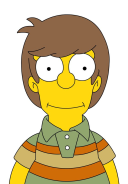
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 03-20-2017 06:22 PM
I tried to get this script going but isn't working for me either. I'm trying to update from 12.4.0 to 12.5.0 but i get this error
Executing Policy Auto-Update Citrix Receiver
Running script autoUpdateCitrixReciever.sh...
Script exit code: 0
Script result: cat: : No such file or directory
Tue Mar 21 12:20:37 :
Tue Mar 21 12:20:37 : ======================== Starting Script ========================
Tue Mar 21 12:20:38 : Latest Citrix Receiver Version is: 12.5.0
Tue Mar 21 12:20:38 : Current Receiver installed version is: 12.4.0
Tue Mar 21 12:20:38 : CRCurrVersNormalized: 1250
Tue Mar 21 12:20:39 : Latest version of the URL is: https:
Tue Mar 21 12:20:39 : Current Receiver version: Citrix Receiver 12.4.0
Tue Mar 21 12:20:39 : Available Receiver version: 12.5.0 => 1250
Tue Mar 21 12:20:39 : Downloading newer version.
Tue Mar 21 12:20:39 : Curl function failed on download! Error: 6. Review error codes here: https://curl.haxx.se/libcurl/c/libcurl-errors.html

- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 04-11-2017 01:37 PM
I'm getting the same results as @khuong_lai : Curl function failed on download! Error: 6. Review error codes here: https://curl.haxx.se/libcurl/c/libcurl-errors.html

- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 04-12-2017 10:28 AM
Hmmm... similar issue here. I have 12.5 installed, so I thought I'd test how it handles that.
bash-3.2# sudo ./CitrixReceiverUpdate.sh
cat: : No such file or directory
Wed Apr 12 13:26:13 :
Wed Apr 12 13:26:13 : ======================== Starting Script ========================
Wed Apr 12 13:26:13 : Latest Citrix Receiver Version is: 12.5
Wed Apr 12 13:26:13 : Current Receiver installed version is: 12.5.0
Wed Apr 12 13:26:13 : CRCurrVersNormalized: 125
Wed Apr 12 13:26:14 : Latest version of the URL is: https:
Wed Apr 12 13:26:14 : Current Receiver version: Citrix Receiver 12.5.0
Wed Apr 12 13:26:14 : Available Receiver version: 12.5 => 125
Wed Apr 12 13:26:14 : Downloading newer version.
Wed Apr 12 13:26:14 : Curl function failed on download! Error: 6. Review error codes here: https://curl.haxx.se/libcurl/c/libcurl-errors.html
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 05-19-2017 10:09 AM
I am getting the same output as cwaldrip.
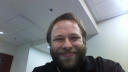
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 05-24-2017 09:51 AM
Is the reported download link valid? It should be in the log.
echoFunc "Latest version of the URL is: $url"
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 05-24-2017 09:57 AM
looks like no? Latest version of the URL is: https:
Wed May 24 09:54:38 : ======================== Starting Script ========================
Wed May 24 09:54:39 : Latest Citrix Receiver Version is: 12.5
Wed May 24 09:54:39 : Current Receiver installed version is: 12.5.0
Wed May 24 09:54:39 : CRCurrVersNormalized: 125
Wed May 24 09:54:40 : Latest version of the URL is: https:
Wed May 24 09:54:40 : Current Receiver version: Citrix Receiver 12.5.0
Wed May 24 09:54:40 : Available Receiver version: 12.5 => 125
Wed May 24 09:54:40 : Downloading newer version.
Wed May 24 09:54:40 : Curl function failed on download! Error: 6. Review error codes here: https://curl.haxx.se/libcurl/c/libcurl-errors.html
m
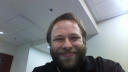
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 05-31-2017 01:00 PM
They changed the website... You need to replace the line that sets url2. This will work as a work around:
url2=`curl -s -L https://www.citrix.com/downloads/citrix-receiver/mac/receiver-for-mac-latest.html#ctx-dl-eula-external | grep dmg? | sed 's/.*rel=.(.*)..id=.*/1/'`
The original author also hard coded a minor version add with 12.3 (so it would become 12.3.0) so I added some regex for that check so it'll match the installed version for everything else (12.5 becomes 12.5.0) Below is the relevant section that needs to be changed:
# Get the latest version of Receiver available from Citrix's Receiver page.
latestver=``
while [ -z "$latestver" ]
do
latestver=`curl -s -L https://www.citrix.com/downloads/citrix-receiver/mac/receiver-for-mac-latest.html | grep "<h1>Receiver " | awk '{print $2}'`
done
if [[ ${latestver} =~ ^[0-9]+.[0-9]+$ ]; then
# Missing minor version to match the installed version, so we’ll add it here.
latestver=${latestver}.0
fi
echoFunc "Latest Citrix Receiver Version is: $latestver"
latestvernorm=`echo ${latestver}`
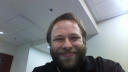
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 05-31-2017 01:41 PM
Here's an updated version that lets you edit OP's contact info and place your ORGs info instead. Check the variable section.
#!/bin/bash
#####################################################################################################
#
# ABOUT THIS PROGRAM
#
# NAME
# CitrixReceiverUpdate.sh -- Installs or updates Citrix Receiver
#
# SYNOPSIS
# sudo CitrixReceiverUpdate.sh
#
# LICENSE
# Distributed under the MIT License
#
# EXIT CODES
# 0 - Citrix Receiver is current
# 1 - Citrix Receiver installed successfully
# 2 - Citrix Receiver NOT installed
# 3 - Citrix Receiver update unsuccessful
# 4 - Citrix Receiver is running or was attempted to be installed manually and user deferred install
# 5 - Not an Intel-based Mac
#
####################################################################################################
#
# HISTORY
#
# Version: 1.2
#
# - v.1.2 Brian Monroe, 05.31.2016 : Fixed downloads and added regex for version matching
# - v.1.1 Luie Lugo, 10.18.2016 : Updated for v12.3, also cleaned up download URL handling
# - v.1.0 Luie Lugo, 09.05.2016 : Updates Citrix Receiver
#
####################################################################################################
# Script to download and install Citrix Receiver.
# Setting variables
receiverProcRunning=0
contactinfo="<YourOrgSupportNameAndInfoHere>"
# Echo function
echoFunc () {
# Date and Time function for the log file
fDateTime () { echo $(date +"%a %b %d %T"); }
# Title for beginning of line in log file
Title="InstallLatestCitrixReceiver:"
# Header string function
fHeader () { echo $(fDateTime) $(hostname) $Title; }
# Check for the log file
if [ -e "/Library/Logs/CitrixReceiverUpdateScript.log" ]; then
echo $(fHeader) "$1" >> "/Library/Logs/CitrixReceiverUpdateScript.log"
else
cat "" > "/Library/Logs/CitrixReceiverUpdateScript.log"
if [ -e "/Library/Logs/CitrixReceiverUpdateScript.log" ]; then
echo $(fHeader) "$1" >> "/Library/Logs/CitrixReceiverUpdateScript.log"
else
echo "Failed to create log file, writing to JAMF log"
echo $(fHeader) "$1" >> "/var/log/jamf.log"
fi
fi
# Echo out
echo $(fDateTime) ": $1"
}
# Exit function
exitFunc () {
case $1 in
0) exitCode="0 - Citrix Receiver is current! Version: $2";;
1) exitCode="1 - SUCCESS: Citrix Receiver has been updated to version $2";;
2) exitCode="2 - ERROR: Citrix Receiver NOT installed!";;
3) exitCode="3 - ERROR: Citrix Receiver update unsuccessful, version remains at $2!";;
4) exitCode="4 - ERROR: Citrix Receiver is running or was attempted to be installed manually and user deferred install.";;
5) exitCode="5 - ERROR: Not an Intel-based Mac.";;
6) exitCode="6 - ERROR: Wireless connected to a known bad WiFi network that won't allow downloading of the installer! SSID: $2";;
*) exitCode="$1";;
esac
echoFunc "Exit code: $exitCode"
echoFunc "======================== Script Complete ========================"
exit $1
}
# Check to see if Citrix Receiver is running
receiverRunningCheck () {
processNum=$(ps aux | grep "Citrix Receiver" | wc -l)
if [ $processNum -gt 1 ]
then
# Receiver is running, prompt the user to close it or defer the upgrade
receiverRunning
fi
}
# If Citrix Receiver is running, prompt the user to close it
receiverRunning () {
echoFunc "Citrix Receiver appears to be running!"
hudTitle="Citrix Receiver Update"
hudDescription="Citrix Receiver needs to be updated. Please save your work and close the application to proceed. You can defer if needed.
If you have any questions, please contact: ${contactinfo}."
#sudo -u $(ls -l /dev/console | awk '{print $3}') utility
jamfHelperPrompt=`/Library/Application Support/JAMF/bin/jamfHelper.app/Contents/MacOS/jamfHelper -windowType hud -lockHUD -title "$hudTitle" -description "$hudDescription" -button1 "Proceed" -button2 "Defer" -defaultButton 1`
case $jamfHelperPrompt in
0)
echoFunc "Proceed selected"
receiverProcRunning=1
receiverRunningCheck
;;
2)
echoFunc "Deferment Selected"
exitFunc 4
;;
*)
echoFunc "Selection: $?"
#receiverProcRunning=1
#receiverRunningCheck
exitFunc 3 "Unknown"
;;
esac
}
# If Citrix Receiver is running, prompt the user to close it
receiverUpdateMan () {
echoFunc "Citrix Receiver appears to be running!"
hudTitle="Citrix Receiver Update"
hudDescription="Citrix Receiver needs to be updated. You will see a program downloading the installer. You can defer if needed.
If you have any questions, please contact: ${contactinfo}."
jamfHelperPrompt=`/Library/Application Support/JAMF/bin/jamfHelper.app/Contents/MacOS/jamfHelper -windowType hud -lockHUD -title "$hudTitle" -description "$hudDescription" -button1 "Defer" -button2 "Proceed" -defaultButton 1 -timeout 60 -countdown`
case $jamfHelperPrompt in
0)
echoFunc "Deferment Selected"
exitFunc 4
;;
2)
echoFunc "Proceed selected"
receiverRunningCheck
;;
*)
echoFunc "Selection: $?"
exitFunc 3 "Unknown"
;;
esac
}
echoFunc ""
echoFunc "======================== Starting Script ========================"
# Are we on a bad wireless network?
if [[ "$4" != "" ]]
then
wifiSSID=`/System/Library/PrivateFrameworks/Apple80211.framework/Versions/Current/Resources/airport -I | awk '/ SSID/ {print substr($0, index($0, $2))}'`
echoFunc "Current Wireless SSID: $wifiSSID"
badSSIDs=( $4 )
for (( i = 0; i < "${#badSSIDs[@]}"; i++ ))
do
if [[ "$wifiSSID" == "${badSSIDs[i]}" ]]
then
echoFunc "Connected to a WiFi network that blocks downloads!"
exitFunc 6 "${badSSIDs[i]}"
fi
done
fi
# Are we running on Intel?
if [ '`/usr/bin/uname -p`'="i386" -o '`/usr/bin/uname -p`'="x86_64" ]; then
## Get OS version and adjust for use with the URL string
OSvers_URL=$( sw_vers -productVersion | sed 's/[.]/_/g' )
## Set the User Agent string for use with curl
userAgent="Mozilla/5.0 (Macintosh; Intel Mac OS X ${OSvers_URL}) AppleWebKit/535.6.2 (KHTML, like Gecko) Version/5.2 Safari/535.6.2"
# Get the latest version of Receiver available from Citrix's Receiver page.
latestver=``
while [ -z "$latestver" ]
do
latestver=`curl -s -L https://www.citrix.com/downloads/citrix-receiver/mac/receiver-for-mac-latest.html | grep "<h1>Receiver " | awk '{print $2}'`
done
if [[ ${latestver} =~ ^[0-9]+.[0-9]+$ ]]; then
# Missing minor version to match the installed version, so we’ll add it here.
latestver=${latestver}.0
fi
echoFunc "Latest Citrix Receiver Version is: $latestver"
latestvernorm=`echo ${latestver}`
# Get the version number of the currently-installed Citrix Receiver, if any.
if [ -e "/Applications/Citrix Receiver.app" ]; then
currentinstalledapp="Citrix Receiver"
currentinstalledver=`/usr/bin/defaults read /Applications/Citrix Receiver.app/Contents/Info CFBundleShortVersionString`
echoFunc "Current Receiver installed version is: $currentinstalledver"
if [ ${latestvernorm} = ${currentinstalledver} ]; then
exitFunc 0 "${currentinstalledapp} ${currentinstalledver}"
else
# Not running the latest version, check if Receiver is running
receiverRunningCheck
fi
else
currentinstalledapp="None"
currentinstalledver="N/A"
exitFunc 2
fi
# Build URL and dmg file name
CRCurrVersNormalized=$( echo $latestver | sed -e 's/[.]//g' )
echoFunc "CRCurrVersNormalized: $CRCurrVersNormalized"
url1="https:"
url2=`curl -s -L https://www.citrix.com/downloads/citrix-receiver/mac/receiver-for-mac-latest.html#ctx-dl-eula-external | grep dmg? | sed 's/.*rel=.(.*)..id=.*/1/'`
url=`echo "${url1}${url2}"`
echoFunc "Latest version of the URL is: $url"
dmgfile="Citrix_Rec_${CRCurrVersNormalized}.dmg"
# Compare the two versions, if they are different or Citrix Receiver is not present then download and install the new version.
if [ "${currentinstalledver}" != "${latestvernorm}" ]; then
echoFunc "Current Receiver version: ${currentinstalledapp} ${currentinstalledver}"
echoFunc "Available Receiver version: ${latestver} => ${CRCurrVersNormalized}"
echoFunc "Downloading newer version."
curl -s -o /tmp/${dmgfile} ${url}
case $? in
0)
echoFunc "Checking if the file exists after downloading."
if [ -e "/tmp/${dmgfile}" ]; then
receiverFileSize=$(du -k "/tmp/${dmgfile}" | cut -f 1)
echoFunc "Downloaded File Size: $receiverFileSize kb"
else
echoFunc "File NOT downloaded!"
exitFunc 3 "${currentinstalledapp} ${currentinstalledver}"
fi
echoFunc "Checking if Receiver is running one last time before we install"
receiverRunningCheck
echoFunc "Mounting installer disk image."
hdiutil attach /tmp/${dmgfile} -nobrowse -quiet
echoFunc "Installing..."
echo "$(date +"%a %b %d %T") Installing Citrix Receiver v$latestver" >> /var/log/CitrixReceiverInstall.log
installer -pkg "/Volumes/Citrix Receiver/Install Citrix Receiver.pkg" -target / >> /var/log/CitrixReceiverInstall.log # > /dev/null
sleep 10
echoFunc "Unmounting installer disk image."
umount "/Volumes/Citrix Receiver"
sleep 10
echoFunc "Deleting disk image."
rm /tmp/${dmgfile}
#double check to see if the new version got update
if [ -e "/Applications/Citrix Receiver.app" ]; then
newlyinstalledver=`/usr/bin/defaults read /Applications/Citrix Receiver.app/Contents/Info CFBundleShortVersionString`
if [ "${latestvernorm}" = "${newlyinstalledver}" ]; then
echoFunc "SUCCESS: Citrix Receiver has been updated to version ${newlyinstalledver}, issuing JAMF recon command"
jamf recon
if [ $receiverProcRunning -eq 1 ];
then
/Library/Application Support/JAMF/bin/jamfHelper.app/Contents/MacOS/jamfHelper -windowType hud -lockHUD -title "Citrix Receiver Updated" -description "Citrix Receiver has been updated to version ${newlyinstalledver}." -button1 "OK" -defaultButton 1
fi
exitFunc 1 "${newlyinstalledver}"
else
exitFunc 3 "${currentinstalledapp} ${currentinstalledver}"
fi
else
exitFunc 3 "${currentinstalledapp} ${currentinstalledver}"
fi
;;
*)
echoFunc "Curl function failed on download! Error: $?. Review error codes here: https://curl.haxx.se/libcurl/c/libcurl-errors.html"
;;
esac
else
# If Citrix Receiver is up to date already, just log it and exit.
exitFunc 0 "${currentinstalledapp} ${currentinstalledver}"
fi
else
# This script is for Intel Macs only.
exitFunc 5
fi
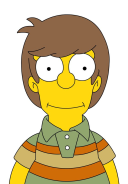
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 06-23-2017 11:17 PM
Script works great again, thanks! I'm noticing that it does the install but JSS still says it fails. Any ideas?
An error occurred while running the policy "Citrix Receiver Update 12.6.0" on the computer "air15-20".
Actions from policy log:
Executing Policy Citrix Receiver Update 12.6.0
Running script autoUpdateCitrixReciever.sh...
Script exit code: 1
Script result: cat: : No such file or directory
Sat Jun 24 13:53:22 :
Sat Jun 24 13:53:22 : ======================== Starting Script ========================
Sat Jun 24 13:53:24 : Latest Citrix Receiver Version is: 12.6.0
Sat Jun 24 13:53:25 : Current Receiver installed version is: 12.4.0
Sat Jun 24 13:53:25 : CRCurrVersNormalized: 1260
Sat Jun 24 13:53:26 : Latest version of the URL is: https://downloads.citrix.com/13154/CitrixReceiver.dmg?gda=1498280000_22209b8e32a3b0a0313d90b8105690c1
Sat Jun 24 13:53:26 : Current Receiver version: Citrix Receiver 12.4.0
Sat Jun 24 13:53:26 : Available Receiver version: 12.6.0 => 1260
Sat Jun 24 13:53:26 : Downloading newer version.
Sat Jun 24 13:54:26 : Checking if the file exists after downloading.
Sat Jun 24 13:54:26 : Downloaded File Size: 55472 kb
Sat Jun 24 13:54:26 : Checking if Receiver is running one last time before we install
Sat Jun 24 13:54:26 : Mounting installer disk image.
Sat Jun 24 13:54:28 : Installing...
Sat Jun 24 13:54:44 : Unmounting installer disk image.
Sat Jun 24 13:54:54 : Deleting disk image.
Sat Jun 24 13:54:54 : SUCCESS: Citrix Receiver has been updated to version 12.6.0, issuing JAMF recon command
Retrieving inventory preferences from https://jss.trinity.edu.au:8443/...
Finding extension attributes...
Locating package receipts...
Locating accounts...
Locating software updates...
Locating applications...
Locating hard drive information...
Searching path: /Applications
Locating printers...
Locating hardware information (Mac OS X 10.11.6)...
Gathering application usage information...
Submitting data to https://jss.trinity.edu.au:8443/...
<computer_id>764</computer_id>
Sat Jun 24 13:55:38 : Exit code: 1 - SUCCESS: Citrix Receiver has been updated to version 12.6.0
Sat Jun 24 13:55:38 : ======================== Script Complete ========================
Error running script: return code was 1.
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 06-24-2017 08:01 AM
@khuong_lai because the script uses Bash exit codes to tell what it has done, and the author chose to use exit code 1 to show a successful install, the JSS will always see it as a failed script. Any exit code other than 0 will be seen as a failed attempt by the JSS, as the JSS expects exit code 0 for success.
Rather than using exit codes, the script should probably be modified to write to an EA via the API, or write to a PLIST file that is then read by an EA, or simply echo some text with what was found.
At any rate, something other than exit codes should be used, or users of the script just need to know a failure isn't necessarily a failure.
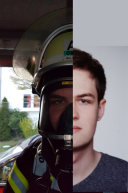
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 06-29-2017 02:53 AM
I had an issue with "Citrix appears to be running" - it always showed up even if the receiver was not running. Then I realised, that ps aux also found different processes. So I changed this module of the script as follows:
# Check to see if Citrix Receiver is running
receiverRunningCheck () {
processNum=$(ps aux | grep "Citrix Receiver.app" | wc -l)
if [ $processNum -gt 0 ]
then
# Receiver is running, prompt the user to close it or defer the upgrade
receiverRunning
fi
}
With this changed module the workflow works for me :-) btw. thank you for the script
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 10-04-2017 01:11 PM
Has anyone had any luck using this script to perform a clean install of Citrix Receiver 12.7?
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 09-18-2018 12:02 PM
Citrix Receiver has become Citrix Workspace.
Any suggestions on how to modify the script to include this new version?
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 09-19-2018 04:20 AM
I have made some quick modifications to the script to support Citrix Workspace . Seems to install ok, but It throws an error 3 at my end.
#!/bin/bash
#####################################################################################################
#
# ABOUT THIS PROGRAM
#
# NAME
# CitrixWorkspaceUpdate.sh -- Installs or updates Citrix Workspace
#
# SYNOPSIS
# sudo CitrixWorkspaceUpdate.sh
#
# LICENSE
# Distributed under the MIT License
#
# EXIT CODES
# 0 - Citrix Workspace is current
# 1 - Citrix Workspace installed successfully
# 2 - Citrix Workspace NOT installed
# 3 - Citrix Workspace update unsuccessful
# 4 - Citrix Workspace is running or was attempted to be installed manually and user deferred install
# 5 - Not an Intel-based Mac
#
####################################################################################################
#
# HISTORY
#
# Version: 1.3
# - v.1.3 Peter Wreiber 09.19.2018 : Added support for Citrix Workspace
# - v.1.2 Brian Monroe, 05.31.2016 : Fixed downloads and added regex for version matching
# - v.1.1 Luie Lugo, 10.18.2016 : Updated for v12.3, also cleaned up download URL handling
# - v.1.0 Luie Lugo, 09.05.2016 : Updates Citrix Receiver
#
####################################################################################################
# Script to download and install Citrix Workspace.
# Setting variables
WorkspaceProcRunning=0
contactinfo="<YourOrgSupportNameAndInfoHere>"
# Echo function
echoFunc () {
# Date and Time function for the log file
fDateTime () { echo $(date +"%a %b %d %T"); }
# Title for beginning of line in log file
Title="InstallLatestCitrixWorkspace:"
# Header string function
fHeader () { echo $(fDateTime) $(hostname) $Title; }
# Check for the log file
if [ -e "/Library/Logs/CitrixWorkspaceUpdateScript.log" ]; then
echo $(fHeader) "$1" >> "/Library/Logs/CitrixWorkspaceUpdateScript.log"
else
cat "" > "/Library/Logs/CitrixWorkspaceUpdateScript.log"
if [ -e "/Library/Logs/CitrixWorkspaceUpdateScript.log" ]; then
echo $(fHeader) "$1" >> "/Library/Logs/CitrixWorkspaceUpdateScript.log"
else
echo "Failed to create log file, writing to JAMF log"
echo $(fHeader) "$1" >> "/var/log/jamf.log"
fi
fi
# Echo out
echo $(fDateTime) ": $1"
}
# Exit function
exitFunc () {
case $1 in
0) exitCode="0 - Citrix Workspace is current! Version: $2";;
1) exitCode="1 - SUCCESS: Citrix Workspace has been updated to version $2";;
2) exitCode="2 - ERROR: Citrix Workspace NOT installed!";;
3) exitCode="3 - ERROR: Citrix Workspace update unsuccessful, version remains at $2!";;
4) exitCode="4 - ERROR: Citrix Workspace is running or was attempted to be installed manually and user deferred install.";;
5) exitCode="5 - ERROR: Not an Intel-based Mac.";;
6) exitCode="6 - ERROR: Wireless connected to a known bad WiFi network that won't allow downloading of the installer! SSID: $2";;
*) exitCode="$1";;
esac
echoFunc "Exit code: $exitCode"
echoFunc "======================== Script Complete ========================"
exit $1
}
# Check to see if Citrix Workspace is running
WorkspaceRunningCheck () {
processNum=$(ps aux | grep "Citrix Workspace" | wc -l)
if [ $processNum -gt 1 ]
then
# Workspace is running, prompt the user to close it or defer the upgrade
WorkspaceRunning
fi
}
# If Citrix Workspace is running, prompt the user to close it
WorkspaceRunning () {
echoFunc "Citrix Workspace appears to be running!"
hudTitle="Citrix Workspace Update"
hudDescription="Citrix Workspace needs to be updated. Please save your work and close the application to proceed. You can defer if needed.
If you have any questions, please contact: ${contactinfo}."
#sudo -u $(ls -l /dev/console | awk '{print $3}') utility
#jamfHelperPrompt=`/Library/Application Support/JAMF/bin/jamfHelper.app/Contents/MacOS/jamfHelper -windowType hud -lockHUD -title "$hudTitle" -description "$hudDescription" -button1 "Proceed" -button2 "Defer" -defaultButton 1`
case $jamfHelperPrompt in
0)
echoFunc "Proceed selected"
WorkspaceProcRunning=1
WorkspaceRunningCheck
;;
2)
echoFunc "Deferment Selected"
exitFunc 4
;;
*)
echoFunc "Selection: $?"
#WorkspaceProcRunning=1
#WorkspaceRunningCheck
exitFunc 3 "Unknown"
;;
esac
}
# If Citrix Workspace is running, prompt the user to close it
WorkspaceUpdateMan () {
echoFunc "Citrix Workspace appears to be running!"
hudTitle="Citrix Workspace Update"
hudDescription="Citrix Workspace needs to be updated. You will see a program downloading the installer. You can defer if needed.
If you have any questions, please contact: ${contactinfo}."
#jamfHelperPrompt=`/Library/Application Support/JAMF/bin/jamfHelper.app/Contents/MacOS/jamfHelper -windowType hud -lockHUD -title "$hudTitle" -description "$hudDescription" -button1 "Defer" -button2 "Proceed" -defaultButton 1 -timeout 60 -countdown`
case $jamfHelperPrompt in
0)
echoFunc "Deferment Selected"
exitFunc 4
;;
2)
echoFunc "Proceed selected"
WorkspaceRunningCheck
;;
*)
echoFunc "Selection: $?"
exitFunc 3 "Unknown"
;;
esac
}
echoFunc ""
echoFunc "======================== Starting Script ========================"
# Are we on a bad wireless network?
if [[ "$4" != "" ]]
then
wifiSSID=`/System/Library/PrivateFrameworks/Apple80211.framework/Versions/Current/Resources/airport -I | awk '/ SSID/ {print substr($0, index($0, $2))}'`
echoFunc "Current Wireless SSID: $wifiSSID"
badSSIDs=( $4 )
for (( i = 0; i < "${#badSSIDs[@]}"; i++ ))
do
if [[ "$wifiSSID" == "${badSSIDs[i]}" ]]
then
echoFunc "Connected to a WiFi network that blocks downloads!"
exitFunc 6 "${badSSIDs[i]}"
fi
done
fi
# Are we running on Intel?
if [ '`/usr/bin/uname -p`'="i386" -o '`/usr/bin/uname -p`'="x86_64" ]; then
## Get OS version and adjust for use with the URL string
OSvers_URL=$( sw_vers -productVersion | sed 's/[.]/_/g' )
## Set the User Agent string for use with curl
userAgent="Mozilla/5.0 (Macintosh; Intel Mac OS X ${OSvers_URL}) AppleWebKit/535.6.2 (KHTML, like Gecko) Version/5.2 Safari/535.6.2"
# Get the latest version of Workspace available from Citrix's Workspace page.
latestver=``
while [ -z "$latestver" ]
do
latestver=`curl -s -L https://www.citrix.com/downloads/workspace-app/mac/workspace-app-for-mac-latest.html#ctx-dl-eula-external | grep "<h1>Citrix " | awk '{print $2}'`
done
if [[ ${latestver} =~ ^[0-9]+.[0-9]+$ ]]; then
# Missing minor version to match the installed version, so we’ll add it here.
latestver=${latestver}.0
fi
echoFunc "Latest Citrix Workspace Version is: $latestver"
latestvernorm=`echo ${latestver}`
# Get the version number of the currently-installed Citrix Workspace, if any.
if [ -e "/Applications/Citrix Workspace.app" ]; then
currentinstalledapp="Citrix Workspace"
currentinstalledver=`/usr/bin/defaults read /Applications/Citrix Workspace.app/Contents/Info CFBundleShortVersionString`
echoFunc "Current Workspace installed version is: $currentinstalledver"
if [ ${latestvernorm} = ${currentinstalledver} ]; then
exitFunc 0 "${currentinstalledapp} ${currentinstalledver}"
fi
fi
# Build URL and dmg file name
CRCurrVersNormalized=$( echo $latestver | sed -e 's/[.]//g' )
echoFunc "CRCurrVersNormalized: $CRCurrVersNormalized"
url1="https:"
url2=`curl -s -L https://www.citrix.com/downloads/workspace-app/mac/workspace-app-for-mac-latest.html#ctx-dl-eula-external | grep dmg? | sed 's/.*rel=.(.*)..id=.*/1/'`
url=`echo "${url1}${url2}"`
echoFunc "Latest version of the URL is: $url"
dmgfile="Citrix_work_${CRCurrVersNormalized}.dmg"
# Compare the two versions, if they are different or Citrix Workspace is not present then download and install the new version.
if [ "${currentinstalledver}" != "${latestvernorm}" ]; then
echoFunc "Current Workspace version: ${currentinstalledapp} ${currentinstalledver}"
echoFunc "Available Workspace version: ${latestver} => ${CRCurrVersNormalized}"
echoFunc "Downloading newer version."
curl -s -o /tmp/${dmgfile} ${url}
case $? in
0)
echoFunc "Checking if the file exists after downloading."
if [ -e "/tmp/${dmgfile}" ]; then
WorkspaceFileSize=$(du -k "/tmp/${dmgfile}" | cut -f 1)
echoFunc "Downloaded File Size: $WorkspaceFileSize kb"
fi
echoFunc "Checking if Workspace is running one last time before we install"
#WorkspaceRunningCheck
echoFunc "Mounting installer disk image."
hdiutil attach /tmp/${dmgfile} -nobrowse -quiet
echoFunc "Installing..."
echo "$(date +"%a %b %d %T") Installing Citrix Workspace v$latestver" >> /var/log/CitrixWorkspaceInstall.log
/usr/sbin/installer -pkg "/Volumes/Citrix Workspace/Install Citrix Workspace.pkg" -target / >> /var/log/CitrixWorkspaceInstall.log # > /dev/null
sleep 10
echoFunc "Unmounting installer disk image."
/sbin/umount /Volumes/Cit*
sleep 10
echoFunc "Deleting disk image."
rm /tmp/${dmgfile}
#double check to see if the new version got update
if [ -e "/Applications/Citrix Workspace.app" ]; then
newlyinstalledver=`/usr/bin/defaults read /Applications/Citrix Workspace.app/Contents/Info CFBundleShortVersionString`
if [ "${latestvernorm}" = "${newlyinstalledver}" ]; then
echoFunc "SUCCESS: Citrix Workspace has been updated to version ${newlyinstalledver}, issuing JAMF recon command"
jamf recon
if [ $WorkspaceProcRunning -eq 1 ];
then
/Library/Application Support/JAMF/bin/jamfHelper.app/Contents/MacOS/jamfHelper -windowType hud -lockHUD -title "Citrix Workspace Updated" -description "Citrix Workspace has been updated to version ${newlyinstalledver}." -button1 "OK" -defaultButton 1
fi
exitFunc 1 "${newlyinstalledver}"
else
exitFunc 3 "${currentinstalledapp} ${currentinstalledver}"
fi
else
exitFunc 3 "${currentinstalledapp} ${currentinstalledver}"
fi
;;
*)
echoFunc "Curl function failed on download! Error: $?. Review error codes here: https://curl.haxx.se/libcurl/c/libcurl-errors.html"
;;
esac
else
# If Citrix Workspace is up to date already, just log it and exit.
exitFunc 0 "${currentinstalledapp} ${currentinstalledver}"
fi
else
# This script is for Intel Macs only.
exitFunc 5
fi
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 09-20-2018 03:09 AM
Tried it at my end and getting same error, appears to download the file but fails when trying to execute the installation bit of the script.
Thu Sep 20 11:06:01 : ======================== Starting Script ========================
Thu Sep 20 11:06:02 : Latest Citrix Workspace Version is: Workspace
Thu Sep 20 11:06:02 : CRCurrVersNormalized: Workspace
Thu Sep 20 11:06:03 : Latest version of the URL is: https://downloads.citrix.com/14987/CitrixWorkspaceApp.dmg?gda=1537441505_5e9cc241de7a57197961ea6b218f4e14
Thu Sep 20 11:06:03 : Current Workspace version:
Thu Sep 20 11:06:03 : Available Workspace version: Workspace => Workspace
Thu Sep 20 11:06:03 : Downloading newer version.
Thu Sep 20 11:06:39 : Checking if the file exists after downloading.
Thu Sep 20 11:06:39 : Downloaded File Size: 114752 kb
Thu Sep 20 11:06:39 : Checking if Workspace is running one last time before we install
Thu Sep 20 11:06:39 : Mounting installer disk image.
Thu Sep 20 11:06:41 : Installing...
Thu Sep 20 11:06:51 : Unmounting installer disk image.
Thu Sep 20 11:07:02 : Deleting disk image.
Thu Sep 20 11:07:02 : Exit code: 3 - ERROR: Citrix Workspace update unsuccessful, version remains at !
Thu Sep 20 11:07:02 : ======================== Script Complete ========================
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-14-2018 07:40 AM
Fixed the exit code 3 problem and also some version parsing and normalisation issues:
!/bin/bash
#########################################################################################
# ABOUT THIS PROGRAM
NAME
CitrixWorkspaceUpdate.sh -- Installs or updates Citrix Workspace
SYNOPSIS
sudo CitrixWorkspaceUpdate.sh
LICENSE
Distributed under the MIT License
EXIT CODES
0 - Citrix Workspace is current
1 - Citrix Workspace installed successfully
2 - Citrix Workspace NOT installed
3 - Citrix Workspace update unsuccessful
4 - Citrix Workspace is running or was attempted to be installed manually and user deferred install
5 - Not an Intel-based Mac
########################################################################################
# HISTORY
Version: 1.4
- v.1.4 Alex Waddell 11.12.2018 : Fixed various version parsing and normalisation issues, resolved exit code 3 problem
- v.1.3 Peter Wreiber 09.19.2018 : Added support for Citrix Workspace
- v.1.2 Brian Monroe, 05.31.2016 : Fixed downloads and added regex for version matching
- v.1.1 Luie Lugo, 10.18.2016 : Updated for v12.3, also cleaned up download URL handling
- v.1.0 Luie Lugo, 09.05.2016 : Updates Citrix Receiver
########################################################################################
Script to download and install Citrix Workspace.
Setting variables
WorkspaceProcRunning=0
contactinfo="NatWest Markets CurrencyPay Support"
Echo function
echoFunc () { # Date and Time function for the log file fDateTime () { echo $(date +"%a %b %d %T"); }
# Title for beginning of line in log file Title="InstallLatestCitrixWorkspace:"
# Header string function fHeader () { echo $(fDateTime) $(hostname) $Title; }
# Check for the log file if [ -e "/Library/Logs/CitrixWorkspaceUpdateScript.log" ]; then echo $(fHeader) "$1" >> "/Library/Logs/CitrixWorkspaceUpdateScript.log" else cat "" > "/Library/Logs/CitrixWorkspaceUpdateScript.log" if [ -e "/Library/Logs/CitrixWorkspaceUpdateScript.log" ]; then echo $(fHeader) "$1" >> "/Library/Logs/CitrixWorkspaceUpdateScript.log" else echo "Failed to create log file, writing to JAMF log" echo $(fHeader) "$1" >> "/var/log/jamf.log" fi fi
# Echo out
echo $(fDateTime) ": $1"
}
Exit function
exitFunc () {
case $1 in
0) exitCode="0 - Citrix Workspace is current! Version: $2";;
1) exitCode="1 - SUCCESS: Citrix Workspace has been updated to version $2";;
2) exitCode="2 - ERROR: Citrix Workspace NOT installed!";;
3) exitCode="3 - ERROR: Citrix Workspace update unsuccessful, version remains at $2!";;
4) exitCode="4 - ERROR: Citrix Workspace is running or was attempted to be installed manually and user deferred install.";;
5) exitCode="5 - ERROR: Not an Intel-based Mac.";;
6) exitCode="6 - ERROR: Wireless connected to a known bad WiFi network that won't allow downloading of the installer! SSID: $2";;
*) exitCode="$1";;
esac
echoFunc "Exit code: $exitCode"
echoFunc "======================== Script Complete ========================"
exit $1
}
Check to see if Citrix Workspace is running
WorkspaceRunningCheck () {
processNum=$(ps aux | grep "Citrix Workspace" | wc -l)
if [ $processNum -gt 1 ]
then
# Workspace is running, prompt the user to close it or defer the upgrade
WorkspaceRunning
fi
}
If Citrix Workspace is running, prompt the user to close it
WorkspaceRunning () { echoFunc "Citrix Workspace appears to be running!" hudTitle="Citrix Workspace Update" hudDescription="Citrix Workspace needs to be updated. Please save your work and close the application to proceed. You can defer if needed.
If you have any questions, please contact: ${contactinfo}."
#sudo -u $(ls -l /dev/console | awk '{print $3}') utility
#jamfHelperPrompt=/Library/Application Support/JAMF/bin/jamfHelper.app/Contents/MacOS/jamfHelper -windowType hud -lockHUD -title "$hudTitle" -description "$hudDescription" -button1 "Proceed" -button2 "Defer" -defaultButton 1
case $jamfHelperPrompt in
0)
echoFunc "Proceed selected"
WorkspaceProcRunning=1
WorkspaceRunningCheck
;;
2)
echoFunc "Deferment Selected"
exitFunc 4
;;
*)
echoFunc "Selection: $?"
#WorkspaceProcRunning=1
#WorkspaceRunningCheck
exitFunc 3 "Unknown"
;;
esac
}
If Citrix Workspace is running, prompt the user to close it
WorkspaceUpdateMan () { echoFunc "Citrix Workspace appears to be running!" hudTitle="Citrix Workspace Update" hudDescription="Citrix Workspace needs to be updated. You will see a program downloading the installer. You can defer if needed.
If you have any questions, please contact: ${contactinfo}."
#jamfHelperPrompt=/Library/Application Support/JAMF/bin/jamfHelper.app/Contents/MacOS/jamfHelper -windowType hud -lockHUD -title "$hudTitle" -description "$hudDescription" -button1 "Defer" -button2 "Proceed" -defaultButton 1 -timeout 60 -countdown
case $jamfHelperPrompt in
0)
echoFunc "Deferment Selected"
exitFunc 4
;;
2)
echoFunc "Proceed selected"
WorkspaceRunningCheck
;;
*)
echoFunc "Selection: $?"
exitFunc 3 "Unknown"
;;
esac
}
echoFunc ""
echoFunc "======================== Starting Script ========================"
Are we on a bad wireless network?
if [[ "$4" != "" ]]
then
wifiSSID=/System/Library/PrivateFrameworks/Apple80211.framework/Versions/Current/Resources/airport -I | awk '/ SSID/ {print substr($0, index($0, $2))}'
echoFunc "Current Wireless SSID: $wifiSSID"
badSSIDs=( $4 )
for (( i = 0; i < "${#badSSIDs[@]}"; i++ ))
do
if [[ "$wifiSSID" == "${badSSIDs[i]}" ]]
then
echoFunc "Connected to a WiFi network that blocks downloads!"
exitFunc 6 "${badSSIDs[i]}"
fi
done
fi
Are we running on Intel?
if [ '/usr/bin/uname -p
'="i386" -o '/usr/bin/uname -p
'="x86_64" ]; then
## Get OS version and adjust for use with the URL string
OSvers_URL=$( sw_vers -productVersion | sed 's/[.]/_/g' )
## Set the User Agent string for use with curl userAgent="Mozilla/5.0 (Macintosh; Intel Mac OS X ${OSvers_URL}) AppleWebKit/535.6.2 (KHTML, like Gecko) Version/5.2 Safari/535.6.2"
# Get the latest version of Workspace available from Citrix's Workspace page.
latestver=``
while [ -z "$latestver" ]
do
latestver=curl -s -L https://www.citrix.com/downloads/workspace-app/mac/workspace-app-for-mac-latest.html#ctx-dl-eula-external | grep "<h1>Citrix " | awk '{print $4}'
done
if [[ ${latestver} =~ ^[0-9].[0-9]$ ]]; then
# Missing minor version to match the installed version, so we’ll add it here.
latestver=${latestver}.0
fi
echoFunc "Latest Available Citrix Workspace Version for install is: $latestver"
latestvernorm=echo ${latestver}
# Get the version number of the currently-installed Citrix Workspace, if any.
if [ -e "/Applications/Citrix Workspace.app" ]; then
currentinstalledapp="Citrix Workspace"
currentinstalledver=/usr/bin/defaults read /Applications/Citrix Workspace.app/Contents/Info CFBundleShortVersionString
currentinstalledvernorm=$( echo $currentinstalledver | sed -e 's/[.]/0/g' )
currentinstalledvernorm=${currentinstalledvernorm:0:4}
echoFunc "Current Workspace installed version is: $currentinstalledvernorm"
if [ ${latestvernorm} = ${currentinstalledvernorm} ]; then
exitFunc 0 "No update needed as ${currentinstalledapp} ${currentinstalledvernorm} is current version"
fi
fi
# Build URL and dmg file name
CRCurrVersNormalized=$( echo $latestver | sed -e 's/[.]//g' )
echoFunc "CRCurrVersNormalized: $CRCurrVersNormalized"
url1="https:"
url2=curl -s -L https://www.citrix.com/downloads/workspace-app/mac/workspace-app-for-mac-latest.html#ctx-dl-eula-external | grep dmg? | sed 's/.rel=.(.)..id=./1/'
url=echo "${url1}${url2}"
echoFunc "Latest version of the URL is: $url"
dmgfile="Citrix_work${CRCurrVersNormalized}.dmg"
# Compare the two versions, if they are different or Citrix Workspace is not present then download and install the new version. if [ "${currentinstalledvernorm}" != "${latestvernorm}" ]; then echoFunc "Current Installed Workspace version: ${currentinstalledapp} ${currentinstalledvernorm}" echoFunc "Available Workspace version : ${latestver} => ${CRCurrVersNormalized}" echoFunc "Downloading newer version." curl -s -o /tmp/${dmgfile} ${url} case $? in 0) echoFunc "Checking if the file exists after downloading." if [ -e "/tmp/${dmgfile}" ]; then WorkspaceFileSize=$(du -k "/tmp/${dmgfile}" | cut -f 1) echoFunc "Downloaded File Size: $WorkspaceFileSize kb" fi echoFunc "Checking if Workspace is running one last time before we install" #WorkspaceRunningCheck echoFunc "Mounting installer disk image." hdiutil attach /tmp/${dmgfile} -nobrowse -quiet echoFunc "Installing..." echo "$(date +"%a %b %d %T") Installing Citrix Workspace v$latestver" >> /var/log/CitrixWorkspaceInstall.log /usr/sbin/installer -pkg "/Volumes/Citrix Workspace/Install Citrix Workspace.pkg" -target / >> /var/log/CitrixWorkspaceInstall.log # > /dev/null
sleep 10 echoFunc "Unmounting installer disk image." /sbin/umount /Volumes/Cit* sleep 10 echoFunc "Deleting disk image." rm /tmp/${dmgfile}
#double check to see if the new version got update
if [ -e "/Applications/Citrix Workspace.app" ]; then
newlyinstalledver=/usr/bin/defaults read /Applications/Citrix Workspace.app/Contents/Info CFBundleShortVersionString
if [ "${latestvernorm}" = "${newlyinstalledver}" ]; then
echoFunc "SUCCESS: Citrix Workspace has been updated to version ${newlyinstalledver}, issuing JAMF recon command"
jamf recon
if [ $WorkspaceProcRunning -eq 1 ];
then
/Library/Application Support/JAMF/bin/jamfHelper.app/Contents/MacOS/jamfHelper -windowType hud -lockHUD -title "Citrix Workspace Updated" -description "Citrix Workspace has been updated to version ${newlyinstalledver}." -button1 "OK" -defaultButton 1
fi
exitFunc 1 "${newlyinstalledver}"
else
exitFunc 3 "${currentinstalledapp} ${currentinstalledver}"
fi
else
exitFunc 3 "${currentinstalledapp} ${currentinstalledver}"
fi
;;
*)
echoFunc "Curl function failed on download! Error: $?. Review error codes here: https://curl.haxx.se/libcurl/c/libcurl-errors.html"
;;
esac
else
# If Citrix Workspace is up to date already, just log it and exit.
exitFunc 0 "No update needed as ${currentinstalledapp} ${currentinstalledvernorm} is current version"
fi
else
# This script is for Intel Macs only.
exitFunc 5
fi
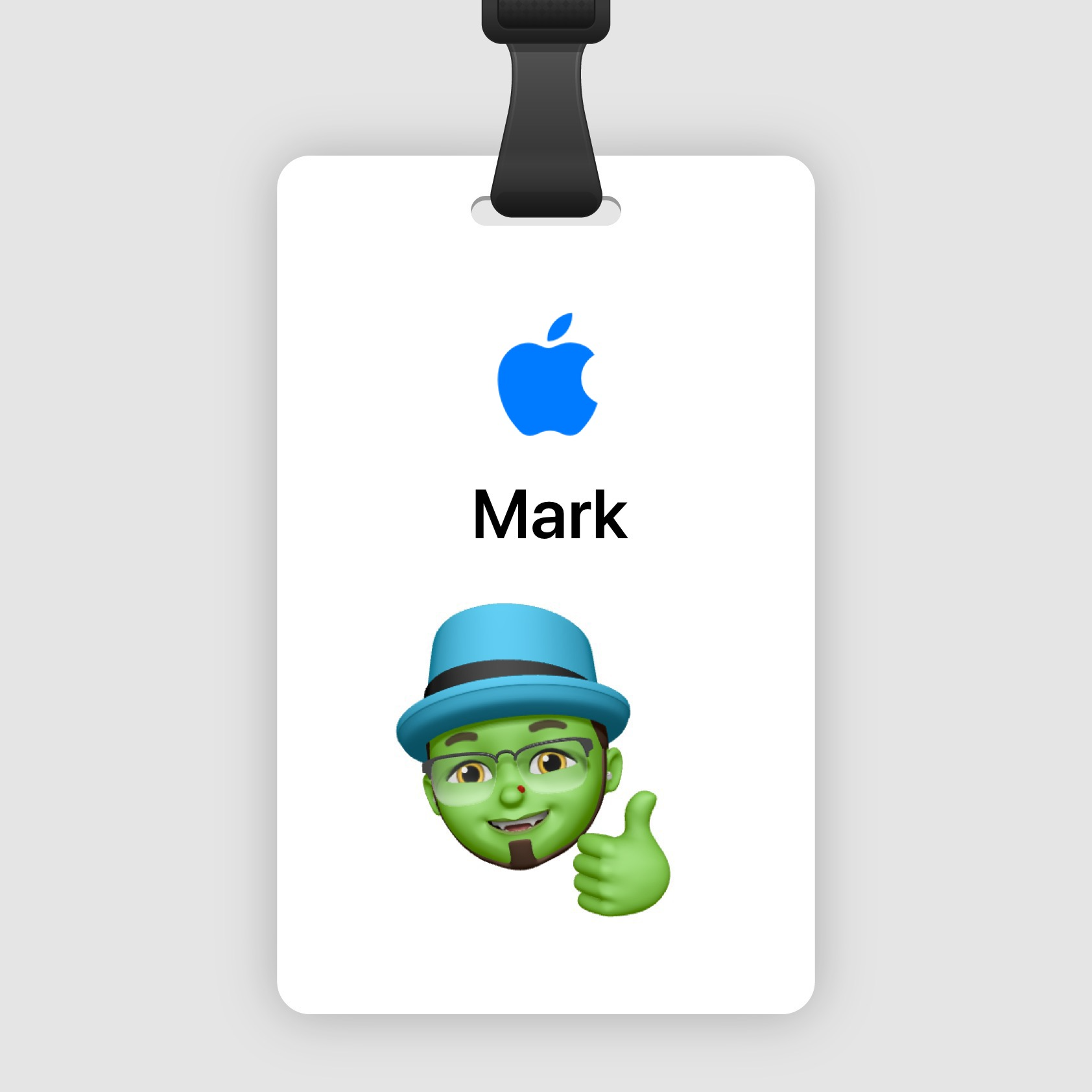
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 11-26-2018 06:00 AM
@peter.wreiber I think Citrix have changed things again.
curl -s -L https://www.citrix.com/downloads/workspace-app/mac/workspace-app-for-mac-latest.html#ctx-dl-eula-external | grep "<h1>Citrix " | awk '{print $2}'
returns Workspace as the full line returned is
<h1>Citrix Workspace app 1809 for Mac</h1>
'{print $4}' gives the version now.
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 12-05-2018 05:12 PM
If suppression is a requirement, good to see these settings are now where hey belong, at the computer level:
#!/bin/sh
/usr/bin/defaults write /Library/Preferences/com.citrix.receiver.nomas AutoUpdateState -string Disabled
/usr/bin/defaults write /Library/Preferences/com.citrix.receiver.nomas HockeySDKAutomaticallySendCrashReports -bool false
/usr/bin/defaults write /Library/Preferences/com.citrix.receiver.nomas HockeySDKCrashReportActivated -bool false
/usr/bin/defaults write /Library/Preferences/com.citrix.receiver.nomas TraceOn -bool false
/usr/bin/defaults write /Library/Preferences/com.citrix.receiver.nomas DisplaySplashScreen -bool false
/usr/bin/defaults write /Library/Preferences/com.citrix.receiver.nomas BITApplicationWasLaunched -bool true
/usr/bin/defaults write /Library/Preferences/com.citrix.receiver.nomas CEIPEnabled -bool false
exit 0
...and yes, can use a Configuration Profile...pick yer poison. :)
https://donmontalvo.com
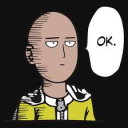
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 01-22-2019 03:27 AM
Hi all,
Is it possible for someone to post the latest complete script version? without the Error 3 code.
I'am fairly new with JAMF and I will follow a course this february to get the hang of it.
I already know how to edit and make profiles and policies with adding scripts etc.
But my scripting skills are what you can call a complete beginner.
Luckily we have you guys :)
Thanks for the help.
Cheers,
Dennis
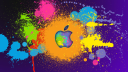
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 01-22-2019 06:49 AM
Hi all,
I am trying to get the Citrix Workspace up and running..
The thing is..... When I install the package via JAMF the Workspace app is not detected.. When I manually install the package all is working fine..
I am going nuts here..... Anyone any idea?
I have tried using this script
I have tried using the original installer
I have tried package made by Autopkr.
All with the same results.. Install is OK (no errors) but Workspace is not detected when starting the saas app. When I install the package interactively all is OK..
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 03-19-2019 11:14 AM
Hi all,
After quite some wrangling I believe I have resolved the script errors - the joy of version numbers changing & needing to be padded and normalised...
#!/bin/bash
#####################################################################################################
#
# ABOUT THIS PROGRAM
#
# NAME
# CitrixWorkspaceUpdate.sh -- Installs or updates Citrix Workspace
#
# SYNOPSIS
# sudo CitrixWorkspaceUpdate.sh
#
# LICENSE
# Distributed under the MIT License
#
# EXIT CODES
# 0 - Citrix Workspace is current
# 1 - Citrix Workspace installed successfully
# 2 - Citrix Workspace NOT installed
# 3 - Citrix Workspace update unsuccessful
# 4 - Citrix Workspace is running or was attempted to be installed manually and user deferred install
# 5 - Not an Intel-based Mac
#
####################################################################################################
#
# HISTORY
#
# Version: 1.5
# - v.1.5 Alex Waddell 22.02.2019 : Fixed (for real this time) various version parsing and normalisation issues, resolved exit code problems
# - v.1.4 Alex Waddell 11.12.2018 : Fixed various version parsing and normalisation issues, resolved exit code 3 problem
# - v.1.3 Peter Wreiber 09.19.2018 : Added support for Citrix Workspace
# - v.1.2 Brian Monroe, 05.31.2016 : Fixed downloads and added regex for version matching
# - v.1.1 Luie Lugo, 10.18.2016 : Updated for v12.3, also cleaned up download URL handling
# - v.1.0 Luie Lugo, 09.05.2016 : Updates Citrix Receiver
#
####################################################################################################
# Script to download and install Citrix Workspace.
# Setting variables
WorkspaceProcRunning=0
contactinfo="NatWest Markets CurrencyPay Support"
# Echo function
echoFunc () {
# Date and Time function for the log file
fDateTime () { echo $(date +"%a %b %d %T"); }
# Title for beginning of line in log file
Title="InstallLatestCitrixWorkspace:"
# Header string function
fHeader () { echo $(fDateTime) $(hostname) $Title; }
# Check for the log file
if [ -e "/Library/Logs/CitrixWorkspaceUpdateScript.log" ]; then
echo $(fHeader) "$1" >> "/Library/Logs/CitrixWorkspaceUpdateScript.log"
else
cat "" > "/Library/Logs/CitrixWorkspaceUpdateScript.log"
if [ -e "/Library/Logs/CitrixWorkspaceUpdateScript.log" ]; then
echo $(fHeader) "$1" >> "/Library/Logs/CitrixWorkspaceUpdateScript.log"
else
echo "Failed to create log file, writing to JAMF log"
echo $(fHeader) "$1" >> "/var/log/jamf.log"
fi
fi
# Echo out
echo $(fDateTime) ": $1"
}
# Exit function
exitFunc () {
case $1 in
0) exitCode="0 - Citrix Workspace is current! Version: $2";;
1) exitCode="1 - SUCCESS: Citrix Workspace has been updated to version $2";;
2) exitCode="2 - ERROR: Citrix Workspace NOT installed!";;
3) exitCode="3 - ERROR: Citrix Workspace update unsuccessful, version remains at $2!";;
4) exitCode="4 - ERROR: Citrix Workspace is running or was attempted to be installed manually and user deferred install.";;
5) exitCode="5 - ERROR: Not an Intel-based Mac.";;
6) exitCode="6 - ERROR: Wireless connected to a known bad WiFi network that won't allow downloading of the installer! SSID: $2";;
*) exitCode="$1";;
esac
echoFunc "Exit code: $exitCode"
echoFunc "======================== Script Complete ========================"
exit $1
}
# Check to see if Citrix Workspace is running
WorkspaceRunningCheck () {
processNum=$(ps aux | grep "Citrix Workspace" | wc -l)
if [ $processNum -gt 1 ]
then
# Workspace is running, prompt the user to close it or defer the upgrade
WorkspaceRunning
fi
}
# If Citrix Workspace is running, prompt the user to close it
WorkspaceRunning () {
echoFunc "Citrix Workspace appears to be running!"
hudTitle="Citrix Workspace Update"
hudDescription="Citrix Workspace needs to be updated. Please save your work and close the application to proceed. You can defer if needed.
If you have any questions, please contact: ${contactinfo}."
#sudo -u $(ls -l /dev/console | awk '{print $3}') utility
#jamfHelperPrompt=`/Library/Application Support/JAMF/bin/jamfHelper.app/Contents/MacOS/jamfHelper -windowType hud -lockHUD -title "$hudTitle" -description "$hudDescription" -button1 "Proceed" -button2 "Defer" -defaultButton 1`
case $jamfHelperPrompt in
0)
echoFunc "Proceed selected"
WorkspaceProcRunning=1
WorkspaceRunningCheck
;;
2)
echoFunc "Deferment Selected"
exitFunc 4
;;
*)
echoFunc "Selection: $?"
#WorkspaceProcRunning=1
#WorkspaceRunningCheck
exitFunc 3 "Unknown"
;;
esac
}
# If Citrix Workspace is running, prompt the user to close it
WorkspaceUpdateMan () {
echoFunc "Citrix Workspace appears to be running!"
hudTitle="Citrix Workspace Update"
hudDescription="Citrix Workspace needs to be updated. You will see a program downloading the installer. You can defer if needed.
If you have any questions, please contact: ${contactinfo}."
#jamfHelperPrompt=`/Library/Application Support/JAMF/bin/jamfHelper.app/Contents/MacOS/jamfHelper -windowType hud -lockHUD -title "$hudTitle" -description "$hudDescription" -button1 "Defer" -button2 "Proceed" -defaultButton 1 -timeout 60 -countdown`
case $jamfHelperPrompt in
0)
echoFunc "Deferment Selected"
exitFunc 4
;;
2)
echoFunc "Proceed selected"
WorkspaceRunningCheck
;;
*)
echoFunc "Selection: $?"
exitFunc 3 "Unknown"
;;
esac
}
echoFunc ""
echoFunc "======================== Starting Script ========================"
# Are we on a bad wireless network?
if [[ "$4" != "" ]]
then
wifiSSID=`/System/Library/PrivateFrameworks/Apple80211.framework/Versions/Current/Resources/airport -I | awk '/ SSID/ {print substr($0, index($0, $2))}'`
echoFunc "Current Wireless SSID: $wifiSSID"
badSSIDs=( $4 )
for (( i = 0; i < "${#badSSIDs[@]}"; i++ ))
do
if [[ "$wifiSSID" == "${badSSIDs[i]}" ]]
then
echoFunc "Connected to a WiFi network that blocks downloads!"
exitFunc 6 "${badSSIDs[i]}"
fi
done
fi
# Are we running on Intel?
if [ '`/usr/bin/uname -p`'="i386" -o '`/usr/bin/uname -p`'="x86_64" ]; then
## Get OS version and adjust for use with the URL string
OSvers_URL=$( sw_vers -productVersion | sed 's/[.]/_/g' )
## Set the User Agent string for use with curl
userAgent="Mozilla/5.0 (Macintosh; Intel Mac OS X ${OSvers_URL}) AppleWebKit/535.6.2 (KHTML, like Gecko) Version/5.2 Safari/535.6.2"
# Get the latest version of Workspace available from Citrix's Workspace page.
latestver=``
while [ -z "$latestver" ]
do
latestver=`curl -s -L https://www.citrix.com/downloads/workspace-app/mac/workspace-app-for-mac-latest.html#ctx-dl-eula-external | grep "<h1>Citrix " | awk '{print $4}'`
done
if [[ ${latestver} =~ ^[0-9]+.[0-9]+$ ]]; then
# Missing minor version to match the installed version, so we’ll add it here.
latestver=${latestver}.0
fi
latestvernorm=`echo ${latestver}`
echoFunc "Latest Available Citrix Workspace Version for install is: $latestvernorm"
currentinstalledvernorm="0000"
# Get the version number of the currently-installed Citrix Workspace, if any.
if [ -e "/Applications/Citrix Workspace.app" ]
then
currentinstalledapp="Citrix Workspace"
currentinstalledver=`/usr/bin/defaults read /Applications/Citrix Workspace.app/Contents/Info CFBundleShortVersionString`
echoFunc "Current installed version is: $currentinstalledver"
if [[ ${currentinstalledver} =~ ^[0-9]{2}.[0-9]{2}.[0-9]{0,2}$ ]];
then
currentinstalledvernorm=$( echo $currentinstalledver | sed -e 's/[.]//g' )
currentinstalledvernorm=${currentinstalledvernorm:0:4}
else
# Missing minor version so we’ll add it here.
currentinstalledvernorm=${currentinstalledver:0:4}
currentinstalledvernorm=$( echo $currentinstalledvernorm | sed -e 's/[.]/0/g' )
fi
echoFunc "Current installed version normalised is: $currentinstalledvernorm"
if [ ${latestvernorm} = ${currentinstalledvernorm} ]; then
exitFunc 0 "No update needed as ${currentinstalledapp} ${currentinstalledvernorm} is current version"
fi
fi
# Build URL and dmg file name
CRCurrVersNormalized=$( echo $latestver | sed -e 's/[.]//g' )
echoFunc "CRCurrVersNormalized: $CRCurrVersNormalized"
url1="https:"
url2=`curl -s -L https://www.citrix.com/downloads/workspace-app/mac/workspace-app-for-mac-latest.html#ctx-dl-eula-external | grep dmg? | sed 's/.*rel=.(.*)..id=.*/1/'`
url=`echo "${url1}${url2}"`
echoFunc "Latest version of the URL is: $url"
dmgfile="Citrix_work_${CRCurrVersNormalized}.dmg"
# Compare the two versions, if they are different or Citrix Workspace is not present then download and install the new version.
if [ "${currentinstalledvernorm}" != "${latestvernorm}" ]
then
echoFunc "Current Installed Workspace version: ${currentinstalledapp} ${currentinstalledvernorm}"
echoFunc "Available Workspace version : ${latestver} => ${currentinstalledvernorm}"
echoFunc "Downloading newer version."
curl -s -o /tmp/${dmgfile} ${url}
case $? in
0)
echoFunc "Checking if the file exists after downloading."
if [ -e "/tmp/${dmgfile}" ]; then
WorkspaceFileSize=$(du -k "/tmp/${dmgfile}" | cut -f 1)
echoFunc "Downloaded File Size: $WorkspaceFileSize kb"
fi
echoFunc "Checking if Workspace is running one last time before we install"
#WorkspaceRunningCheck
echoFunc "Mounting installer disk image."
hdiutil attach /tmp/${dmgfile} -nobrowse -quiet
echoFunc "Installing..."
echo "$(date +"%a %b %d %T") Installing Citrix Workspace v$latestver" >> /var/log/CitrixWorkspaceInstall.log
/usr/sbin/installer -pkg "/Volumes/Citrix Workspace/Install Citrix Workspace.pkg" -target / >> /var/log/CitrixWorkspaceInstall.log # > /dev/null
sleep 10
echoFunc "Unmounting installer disk image."
/sbin/umount /Volumes/Cit*
sleep 10
echoFunc "Deleting disk image."
rm /tmp/${dmgfile}
sleep 10
#double check to see if the new version got update
if [ -e "/Applications/Citrix Workspace.app" ]
then
newlyinstalledver=`/usr/bin/defaults read /Applications/Citrix Workspace.app/Contents/Info CFBundleShortVersionString`
echoFunc "Newly installed version is: $newlyinstalledver"
if [[ ${newlyinstalledver} =~ ^[0-9]{2}.[0-9]{2}.[0-9]{0,2}$ ]];
then
newlyinstalledvernorm=$( echo $newlyinstalledver | sed -e 's/[.]//g' )
newlyinstalledvernorm=${newlyinstalledvernorm:0:4}
else
# Missing minor version so we’ll add it here.
newlyinstalledvernorm=${newlyinstalledver:0:4}
newlyinstalledvernorm=$( echo $newlyinstalledvernorm | sed -e 's/[.]/0/g' )
fi
if [ "${latestvernorm}" = "${newlyinstalledvernorm}" ]
then
echoFunc "SUCCESS: Citrix Workspace has been updated to version ${newlyinstalledvernorm}, issuing JAMF recon command"
jamf recon
if [ $WorkspaceProcRunning -eq 1 ]
then
/Library/Application Support/JAMF/bin/jamfHelper.app/Contents/MacOS/jamfHelper -windowType hud -lockHUD -title "Citrix Workspace Updated" -description "Citrix Workspace has been updated to version ${newlyinstalledvernorm}." -button1 "OK" -defaultButton 1
fi
exitFunc 0 "${newlyinstalledvernorm}"
else
exitFunc 3 "${currentinstalledapp} ${currentinstalledvernorm}"
fi
else
exitFunc 3 "${currentinstalledapp} ${currentinstalledvernorm}"
fi
;;
*)
echoFunc "Curl function failed on download! Error: $?. Review error codes here: https://curl.haxx.se/libcurl/c/libcurl-errors.html"
;;
esac
else
# If Citrix Workspace is up to date already, just log it and exit.
exitFunc 0 "No update needed as ${currentinstalledapp} ${currentinstalledvernorm} is current version"
fi
else
# This script is for Intel Macs only.
exitFunc 5
fi
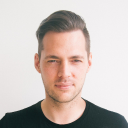
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 03-19-2019 08:19 PM
Just saw someone else beat me to it
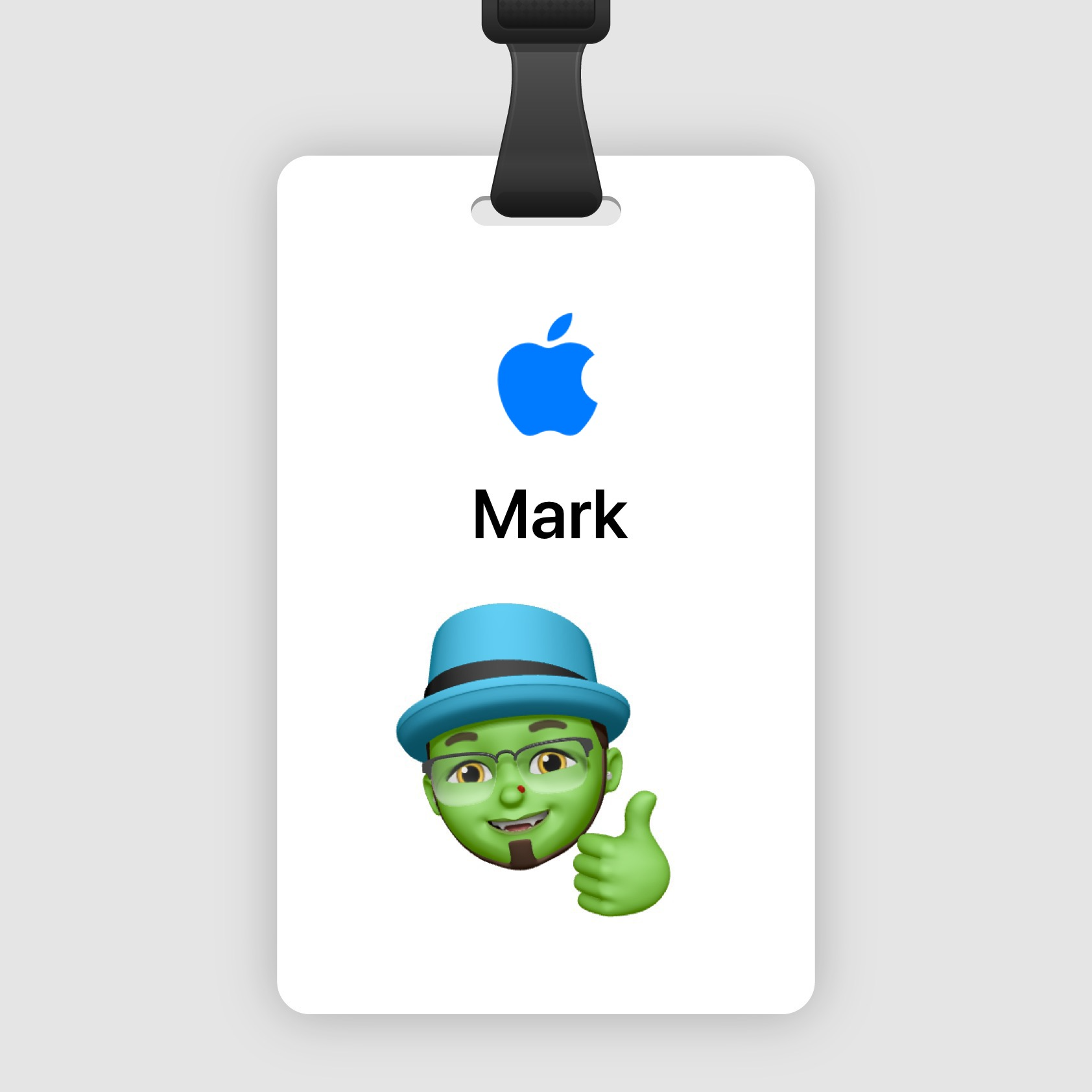
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 05-15-2019 04:46 AM
@alexwaddell I'm going to delve into the script but I just tested it to do an install, which it performed ok but reported a fail
Wed May 15 04:42:47 : Newly installed version is: 19.3.1 Wed May 15 04:42:47 : Exit code: 3 - ERROR: Citrix Workspace update unsuccessful, version remains at 0000!
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 05-15-2019 05:13 AM
looks awesome! Thank you for everybody's hard work!
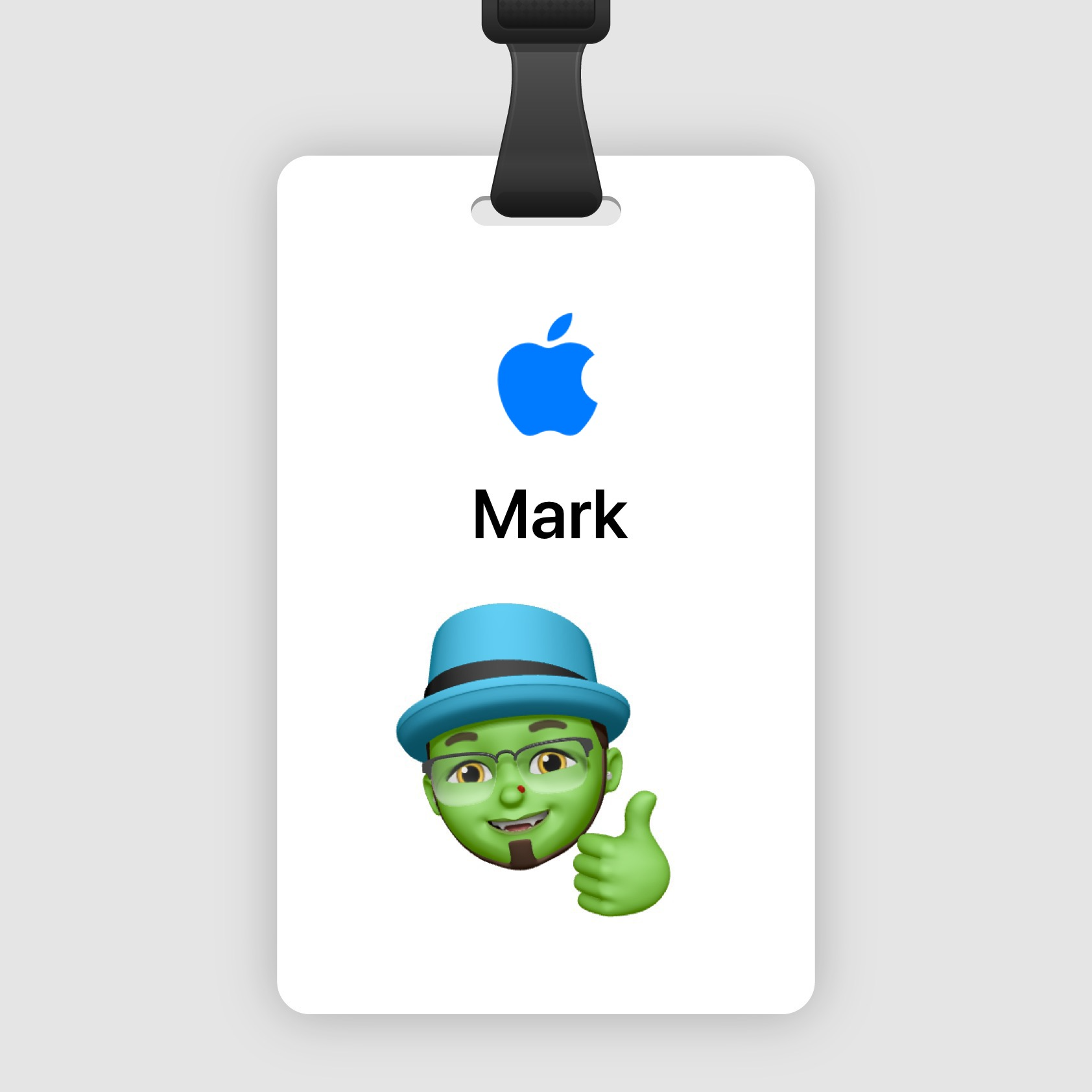
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 05-15-2019 05:45 AM
@dsmetham how about posting your script as well?
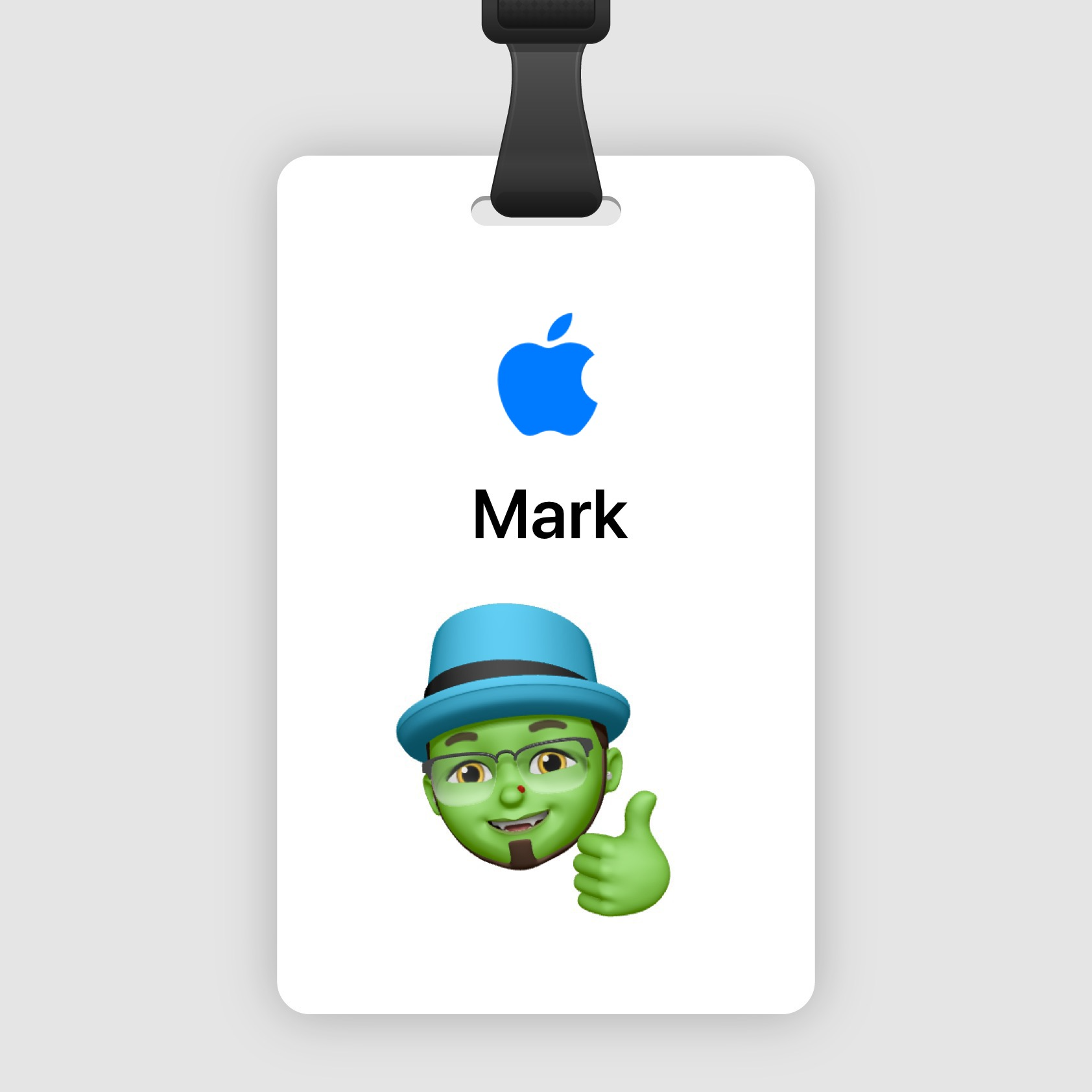
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 05-15-2019 01:26 PM
So I got bitten by the bug on this one as I actually need it for a job and ended up with this version. It doesn't go the complexity of checking the version to 3 sub versions, as from what I can see the important bit is the first two. If Workplace is running then it simply tidies up and exits to await another try.
The exit codes are tweaked to only be 0 or 1 so jamf reports pass or fail correctly. expected results; no update required, update worked and workplace running all are pass for me. all others are fail.
here it is
#!/bin/bash
#####################################################################################################
#
# ABOUT THIS PROGRAM
#
# NAME
# CitrixWorkspaceUpdate.sh -- Installs or updates Citrix Workspace
#
# SYNOPSIS
# sudo CitrixWorkspaceUpdate.sh
#
# LICENSE
# Distributed under the MIT License
#
# EXIT CODES
# 0 - Citrix Workspace is current
# 1 - Citrix Workspace installed successfully
# 2 - Citrix Workspace NOT installed
# 3 - Citrix Workspace update unsuccessful
# 4 - Citrix Workspace is running
# 5 - Not an Intel-based Mac
#
# these are logged but not passed to jamf as anything other than 0 is a failed script
#
####################################################################################################
#
# HISTORY
#
#
# - v.1.7 Mark Lamont 15.05.2019 : made a whole bunch of tidy ups of bits I added for debug and changed logic round app running check
# - v.1.6 Mark Lamont 15.05.2019 : made some changes to how the version check works, only uses xx.xx and made it so only two exit codes: 0 and 1 so jaf doesn't flag false fails for a success.
# - v.1.5 Alex Waddell 22.02.2019 : Fixed (for real this time) various version parsing and normalisation issues, resolved exit code problems
# - v.1.4 Alex Waddell 11.12.2018 : Fixed various version parsing and normalisation issues, resolved exit code 3 problem
# - v.1.3 Peter Wreiber 09.19.2018 : Added support for Citrix Workspace
# - v.1.2 Brian Monroe, 05.31.2016 : Fixed downloads and added regex for version matching
# - v.1.1 Luie Lugo, 10.18.2016 : Updated for v12.3, also cleaned up download URL handling
# - v.1.0 Luie Lugo, 09.05.2016 : Updates Citrix Receiver
#
####################################################################################################
# Script to download and install Citrix Workspace.
# Setting variables
WorkspaceProcRunning=0
contactinfo="IT Support"
# Echo function
echoFunc () {
# Date and Time function for the log file
fDateTime () { echo $(date +"%a %b %d %T"); }
# Title for beginning of line in log file
Title="InstallLatestCitrixWorkspace:"
# Header string function
fHeader () { echo $(fDateTime) $(hostname) $Title; }
# Check for the log file
if [ -e "/Library/Logs/CitrixWorkspaceUpdateScript.log" ]; then
echo $(fHeader) "$1" >> "/Library/Logs/CitrixWorkspaceUpdateScript.log"
else
cat "" > "/Library/Logs/CitrixWorkspaceUpdateScript.log"
if [ -e "/Library/Logs/CitrixWorkspaceUpdateScript.log" ]; then
echo $(fHeader) "$1" >> "/Library/Logs/CitrixWorkspaceUpdateScript.log"
else
echo "Failed to create log file, writing to JAMF log"
echo $(fHeader) "$1" >> "/var/log/jamf.log"
fi
fi
# Echo out
echo $(fDateTime) ": $1"
}
# Exit function
exitFunc () {
case $1 in
0) exitCode="0 - Citrix Workspace is current! Version: $2";;
1) exitCode="1 - SUCCESS: Citrix Workspace has been updated to version $2";;
2) exitCode="2 - ERROR: Citrix Workspace NOT installed!";;
3) exitCode="3 - ERROR: Citrix Workspace update unsuccessful, version remains at $2!";;
4) exitCode="4 - ERROR: Citrix Workspace is running.";;
5) exitCode="5 - ERROR: Not an Intel-based Mac.";;
6) exitCode="6 - ERROR: Wireless connected to a known bad WiFi network that won't allow downloading of the installer! SSID: $2";;
*) exitCode="$1";;
esac
echoFunc "Exit code: $exitCode"
echoFunc "======================== Script Complete ========================"
# if exit code is expected the exit 0 else exit 1 so it shows correctly in jamf
if [ "$1" = "0" ] || [ "$1" = "1" ] || [ "$1" = "4" ]; then
echo "exit 0"
exit 0
else
echo "exit 1"
exit 1
fi
}
# Check to see if Citrix Workspace is running
WorkspaceRunningCheck () {
isRunning=$(ps ax | grep "Citrix Workspace" | grep -v grep | wc -l | sed 's/ //g')
echoFunc "isRunning is $isRunning"
if [[ $isRunning == 0 ]]
then
echoFunc "Workspace is NOT running, continuing"
else
# Workspace is running,
echoFunc "Workspace is running, get out of here"
WorkspaceRunning
fi
}
WorkspaceRunning () {
# workspace running. let's not interupt and we'll wait 'till next run'
# tidy up
echoFunc "tidying up downloaded dmg"
rm /tmp/${dmgfile}
exitFunc 4
}
echoFunc ""
echoFunc "======================== Starting Script ========================"
# Are we running on Intel?
if [ '`/usr/bin/uname -p`'="i386" -o '`/usr/bin/uname -p`'="x86_64" ]; then
## Get OS version and adjust for use with the URL string
OSvers_URL=$( sw_vers -productVersion | sed 's/[.]/_/g' )
## Set the User Agent string for use with curl
userAgent="Mozilla/5.0 (Macintosh; Intel Mac OS X ${OSvers_URL}) AppleWebKit/535.6.2 (KHTML, like Gecko) Version/5.2 Safari/535.6.2"
# Get the latest version of Workspace available from Citrix's Workspace page.
latestver=``
while [ -z "$latestver" ]
do
latestver=`curl -s -L https://www.citrix.com/downloads/workspace-app/mac/workspace-app-for-mac-latest.html#ctx-dl-eula-external | grep "<h1>Citrix " | awk '{print $4}'`
done
if [[ ${latestver} =~ ^[0-9]+.[0-9]+$ ]]; then
latestvershort=${latestver:0:4}
fi
latestvernorm=`echo ${latestver}`
echoFunc "Latest Available Citrix Workspace Version for install is: $latestvernorm"
echoFunc "latest version to compare is $latestvershort"
# default value, stays at this if not installed
currentinstalledvernorm="0000"
# Get the version number of the currently-installed Citrix Workspace, if any.
if [ -e "/Applications/Citrix Workspace.app" ]
then
currentinstalledapp="Citrix Workspace"
currentinstalledver=`/usr/bin/defaults read /Applications/Citrix Workspace.app/Contents/Info CFBundleShortVersionString`
echoFunc "Current installed version in full: $currentinstalledver"
if [[ ${currentinstalledver} =~ ^[0-9]{2}.[0-9]{2}.[0-9]{0,2}$ ]];
then
currentinstalledvernorm=$( echo $currentinstalledver | sed -e 's/[.]//g' )
currentinstalledvernorm=${currentinstalledvernorm:0:4}
#echoFunc "Installed version $currentinstalledvernorm"
else
currentinstalledvernorm=${currentinstalledver:0:4}
currentinstalledvernorm=$( echo $currentinstalledvernorm | sed -e 's/[.]/0/g' )
#echoFunc "Installed version $currentinstalledvernorm"
fi
echoFunc "Current installed version to compare is: $currentinstalledvernorm"
if [ ${latestvershort} = ${currentinstalledvernorm} ]; then
exitFunc 0 "No update needed as ${currentinstalledapp} ${currentinstalledvernorm} is current version"
fi
fi
# Build URL and dmg file name
CRCurrVersNormalized=$( echo $latestver | sed -e 's/[.]//g' )
echoFunc "CRCurrVersNormalized: $CRCurrVersNormalized"
url1="https:"
url2=`curl -s -L https://www.citrix.com/downloads/workspace-app/mac/workspace-app-for-mac-latest.html#ctx-dl-eula-external | grep dmg? | sed 's/.*rel=.(.*)..id=.*/1/'`
url=`echo "${url1}${url2}"`
echoFunc "Latest version of the URL is: $url"
dmgfile="Citrix_work_${CRCurrVersNormalized}.dmg"
# Compare the two versions, if they are different or Citrix Workspace is not present then download and install the new version.
if [ "${currentinstalledvernorm}" != "${latestvershort}" ]
then
echoFunc "Current Installed Workspace version: ${currentinstalledapp} ${currentinstalledvernorm}"
echoFunc "Available Workspace version : ${latestvershort} => ${currentinstalledvernorm}"
echoFunc "Downloading newer version."
curl -s -o /tmp/${dmgfile} ${url}
case $? in
0)
echoFunc "Checking if the file exists after downloading."
if [ -e "/tmp/${dmgfile}" ]; then
WorkspaceFileSize=$(du -k "/tmp/${dmgfile}" | cut -f 1)
echoFunc "Downloaded File Size: $WorkspaceFileSize kb"
fi
echoFunc "Checking if Workspace is running before we install"
WorkspaceRunningCheck
echoFunc "Mounting installer disk image."
hdiutil attach /tmp/${dmgfile} -nobrowse -quiet
echoFunc "Installing Citrix Workspace v$latestver"
echo "$(date +"%a %b %d %T") Installing Citrix Workspace v$latestver" >> /var/log/CitrixWorkspaceInstall.log
/usr/sbin/installer -pkg "/Volumes/Citrix Workspace/Install Citrix Workspace.pkg" -target / >> /var/log/CitrixWorkspaceInstall.log # > /dev/null
sleep 10
echoFunc "Unmounting installer disk image."
/sbin/umount /Volumes/Cit*
sleep 10
echoFunc "Deleting disk image."
rm /tmp/${dmgfile}
sleep 10
#double check to see if the new version got update
if [ -e "/Applications/Citrix Workspace.app" ]
then
newlyinstalledver=`/usr/bin/defaults read /Applications/Citrix Workspace.app/Contents/Info CFBundleShortVersionString`
echoFunc "Newly installed version is: $newlyinstalledver"
if [[ ${newlyinstalledver} =~ ^[0-9]{2}.[0-9]{2}.[0-9]{0,2}$ ]];
then
newlyinstalledvernorm=$( echo $newlyinstalledver | sed -e 's/[.]//g' )
newlyinstalledvernorm=${newlyinstalledvernorm:0:4}
echoFunc "Installed version now $newlyinstalledvernorm"
else
newlyinstalledvernorm=${newlyinstalledver:0:4}
newlyinstalledvernorm=$( echo $newlyinstalledvernorm | sed -e 's/[.]/0/' )
echoFunc "Installed version now $newlyinstalledvernorm"
if [[ ${newlyinstalledvernorm} =~ ^[0-9]+.[0-9]+$ ]]; then
echoFunc "installed version tweaked $newlyinstalledvernorm"
fi
fi
if [ "${latestvershort}" = "${newlyinstalledvernorm}" ]
then
echoFunc "SUCCESS: Citrix Workspace has been updated to version ${newlyinstalledvernorm}, issuing JAMF recon command"
jamf recon
if [ $WorkspaceProcRunning -eq 1 ]
then
/Library/Application Support/JAMF/bin/jamfHelper.app/Contents/MacOS/jamfHelper -windowType hud -lockHUD -title "Citrix Workspace Updated" -description "Citrix Workspace has been updated to version ${newlyinstalledvernorm}." -button1 "OK" -defaultButton 1
fi
exitFunc 0 "${newlyinstalledvernorm}"
else
exitFunc 3 "${currentinstalledapp} ${currentinstalledvernorm}"
fi
else
exitFunc 3 "${currentinstalledapp} ${currentinstalledvernorm}"
fi
;;
*)
echoFunc "Curl function failed on download! Error: $?. Review error codes here: https://curl.haxx.se/libcurl/c/libcurl-errors.html"
;;
esac
else
# If Citrix Workspace is up to date already, just log it and exit.
exitFunc 0 "No update needed as ${currentinstalledapp} ${currentinstalledvernorm} is current version"
fi
else
# This script is for Intel Macs only.
exitFunc 5
fi
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 08-16-2019 09:39 AM
Love this, and thank you.
I do see the following however:
Fri Aug 16 11:13:19 : Exit code: 3 - ERROR: Citrix Workspace update unsuccessful, version remains at Citrix Workspace 1903!
it seems to show fail no matter what.
Did something change not the URLS?
Thanks
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 03-04-2020 10:01 AM
follow
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 03-11-2020 09:22 AM
@marklamont I ran your script on a fresh installed of OS X and it's telling me that Citrix is running but Citrix is not installed yet. Any idea?
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 08-02-2023 06:03 AM
Hi all,
two months ago I needed to do a little adjustment to this script at my company.
The script wasn't downloading and installing Citrix.
I found out that the website for the download changed. It now offers two dmg files (An Intel only and an Universal App version).
As a result the script uses an invalid URL (containing both links) for downloading.
I fixed this by adding
tail -n1
in the following line:
url2=`curl -s -L https://www.citrix.com/downloads/workspace-app/mac/workspace-app-for-mac-latest.html#ctx-dl-eula-external | grep dmg? | tail -n1 | sed 's/.*rel=.\(.*\)..id=.*/\1/'`
This way the script grabs only the last link (the Universal App).
I thought this might be helpful for some of you.
