script help
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 08-23-2019 11:09 AM
I have a script that I'm using for computer renaming...but one part of it where it looks at whether the model name is MacBook (and then assigning ML) or not (assigning MD) - the results are saying everything is not a MacBook (assigning MD).
#!/bin/bash
ModelType=$(system_profiler SPHardwareDataType | grep 'Model Name:' | awk '{ print $3 }')
if [ "$ModelType" = "MacBook" ]; then
TT=ML
else
TT=MD
fi
As a side note, further down in the script, it pops up a prompt for user input via osascript - which is all working fine, but if the user isn't fast enough typing in it goes to the next prompt .... can someone advise how to have it wait for the input before skipping to the next portion?
BB=$(/usr/bin/osascript -e 'tell application "System Events" to set BRAND to text returned of (display dialog "Please input the brand - AF , AI, AU" default answer "" with icon 2)')
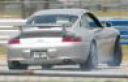
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 08-23-2019 12:12 PM
@jwojda How about this for determining Mac type:
model=$(ioreg -l | grep "product-name" | cut -d "=" -f 2 | sed -e s/[^[:alnum:]]//g | sed s/[0-9]//g)
# Default to a Desktop
TT="MD"
isMacbook=$(echo "$model" | grep "Book")
if [[ $isMacbook != "" ]]; then
TT="ML"
fi
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 08-23-2019 12:33 PM
@sdagley same result. I did have to put $isMacBook into "$isMacbook" to make it not error out, but after doing that it still went to MD
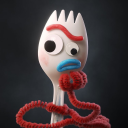
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 08-23-2019 12:52 PM
I gave the code from your original post a run and it's properly returning ML for me. Might I suggest echoing out $ModelType before the IF statement and see what value it's entering that statement with? Maybe awk is cutting things off that we're not expecting?
Also, just a side note for defining variables, TT would be a string, so I'd recommend redoing those assignments to:
TT="MD"
Not the most essential thing in the world, and certainly isn't the issue here, but it's a decent practice to make sure your variable types are always correctly defined :)
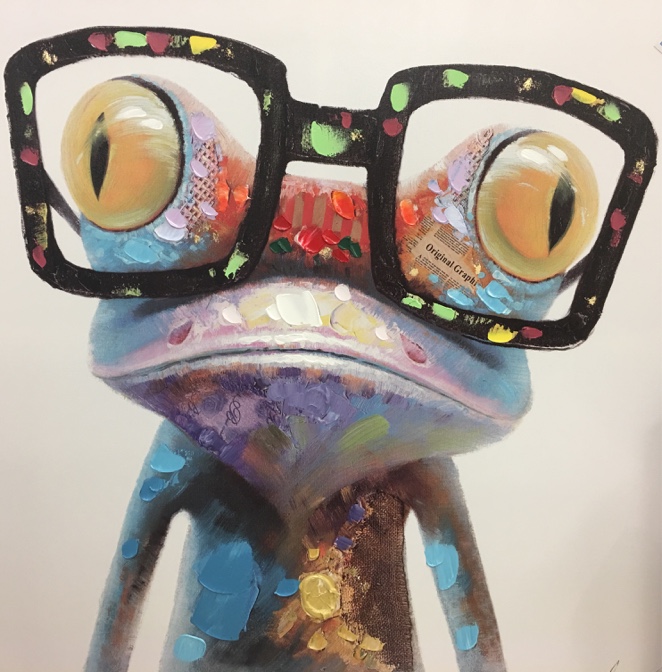
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 08-23-2019 05:18 PM
This is what I use as a template when I want to set different things depending if it's a macbook or not...
# Detects if this Mac is a laptop or not by checking the model ID for the word "Book" in the name.
IS_LAPTOP=$(/usr/sbin/system_profiler SPHardwareDataType | grep "Model Identifier" | grep "Book")
modelName=$(/usr/sbin/system_profiler SPHardwareDataType | awk -F': ' ' /Model Name/{print $NF}')
if [ "$IS_LAPTOP" != "" ]; then
/usr/bin/pmset -b sleep 15 disksleep 10 displaysleep 5 halfdim 1
/usr/bin/pmset -c sleep 0 disksleep 0 displaysleep 30 halfdim 1
else
/usr/bin/pmset sleep 0 disksleep 0 displaysleep 30 halfdim 1
fi
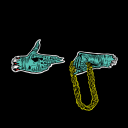
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 08-23-2019 08:37 PM
I like reading the system_profiler
data into Python's plistlib
because then you can just parse the dictionary outputs pretty easily, an example I just whipped up:
#!/usr/bin/python
import subprocess
import plistlib
def get_device_model():
cmd = ['/usr/sbin/system_profiler', 'SPHardwareDataType', '-xml']
proc = subprocess.Popen(cmd, stdout=subprocess.PIPE, stderr=subprocess.PIPE)
out, err = proc.communicate()
data = plistlib.readPlistFromString(out)
model = data[0]['_items'][0]['machine_model']
return model
model = get_device_model()
if 'MacBook' in model:
print('MacBook Found')
When I run the code on my MacBook Pro:
/usr/bin/python2.7 /Users/tlarkin/IdeaProjects/test/get_model.py
MacBook Found
All of these items could be parsed and tested against in code using a simple dictionary as well. This is truncated output in plist format from the shell, it puts them into a dictionary with the key as _items
and then key value for the rest of the data
<key>_items</key>
<array>
<dict>
<key>_name</key>
<string>hardware_overview</string>
<key>boot_rom_version</key>
<string>220.270.99.0.0 (iBridge: 16.16.6568.0.0,0)</string>
<key>cpu_type</key>
<string>Intel Core i9</string>
<key>current_processor_speed</key>
<string>2.9 GHz</string>
<key>l2_cache_core</key>
<string>256 KB</string>
<key>l3_cache</key>
<string>12 MB</string>
<key>machine_model</key>
<string>MacBookPro15,1</string>
<key>machine_name</key>
<string>MacBook Pro</string>
<key>number_processors</key>
<integer>6</integer>
<key>packages</key>
<integer>1</integer>
<key>physical_memory</key>
<string>32 GB</string>
<key>platform_UUID</key>
<string>53685B89-8388-55AF-A129-3B8EC1ED00C1</string>
<key>platform_cpu_htt</key>
<string>htt_enabled</string>
<key>serial_number</key>
<string>C02XG4K6JGH5</string>
</dict>
</array>
Hope this helps some of y'all
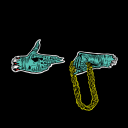
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 08-23-2019 08:40 PM
For waiting for a prompt you can build a loop around that and break the loop once you have desired input, and for bonus points you can count how many times the loop runs, or give it a time out before calling break in code and moving forward.
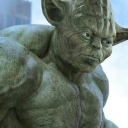
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 08-25-2019 01:01 AM
This is what we use for renaming.
#!/bin/bash
##############################################################################################################
# renameMacCSV.sh
# Modified by Reggie Santos
# Last modified 05/31/2018
# add auto-prefix function using system_profiler SPHardwareDataType | grep "Model Identifier" if not using Custom Prefix in parameter $4
#Written by: Frederick Abeloos | Field Technician | Jamf
#Created On: March 8th, 2018 / script is provided "AS IS" /
#Purpose: Jamf Pro @enrollment script to rename the mac prior to binding to AD (optional)
#Put this script in a policy with option AFTER (important when using .pkg)
#Upload a pkg in JamfPro which puts an assettag.csv file in /tmp/assettags.csv
#First column serialnumber and second column the desired assettag
#OR use CURL to download any .csv from a public link
# Optional: Create a policy with the directory binding payload and custom trigger 'bindToAD'
##############################################################################################################
### RENAMING SETTINGS ########################################################################################
# Don't change any settings here. Use $4-$9 parameters in Jamf Pro script payload options.
# First choose a naming convention for your Mac.
# Custom Prefix can be set via the $4 parameter in the Jamf Pro policy.
# Leave the parameter $4 in Jamf Pro empty if you like to auto-prefix computername base on "Model Identifier", e.g. type "MBP-" (without the quotes).
prefix="$4"
# Suffix will be set via the $5 parameter in the Jamf Pro policy (script payload).
# Leave the parameter empty if no suffix is required. e.g. type "Mac_" (without the quotes).
suffix="$5"
# Choose serialnumber or assettag via the $6 parameter in the Jamf Pro policy (script payload).
# Set it to "serialnumber" or "assettag" (without the quotes).
namingType="$6"
# Define if you are adding the .pkg with the /tmp/assettags.csv file or using curl to download any .csv file.
# When using curl, the script below will download and rename the file to /tmp/assettags.csv
# Type will be set via $7 paremeter in the Jamf Pro Policy. Type "curl" or "package" (without the quotes).
csvType="$7"
# Define the public link to the .csv file. Leave blank when using .pkg
# Link will be set via th $8 parameter in the Jamf Pro policy. Add the full "https://URL" (without the quotes).
csvURL="$8"
# Path to local csv file, via package in same policy: pkg putting assettags.csv in /tmp/
csvPath="/tmp/assettags.csv"
# Do you want to bind or not? Set to "bind" or leave blank
bindSetting="$9"
### RENAMING SCRIPT ########################################################################################
MBA=`/usr/sbin/system_profiler SPHardwareDataType | grep "Model Identifier" | grep "BookAir"`
MBP=`/usr/sbin/system_profiler SPHardwareDataType | grep "Model Identifier" | grep "BookPro"`
if [ "$4" == "" ] && [ "$MBP" == "" ] && [ "$MBA" != "" ]; then
prefix="MBA"
elif [ "$4" == "" ] && [ "$MBA" == "" ] && [ "$MBP" != "" ]; then
prefix="MBP"
elif [ "$4" == "" ] && [ "$MBA" == "" ] && [ "$MBP" == "" ]; then
prefix="MAC"
fi
if [ "$csvType" = "curl" ]; then
curl -L -o $csvPath $csvURL
fi
if [ "$namingType" = "serialnumber" ]; then
echo "using serialnumber"
jamf setComputerName -useSerialNumber -prefix $prefix -suffix $suffix
elif [ "$namingType" = "assettag" ]; then
echo "using assettag"
jamf setComputerName -fromFile "$csvPath" -prefix $prefix -suffix $suffix
fi
### AD BINDING ###########################################################################################
if [ "$bindSetting" = "bind" ]; then
echo "Binding to AD"
jamf policy -event bindToAD
else echo "Not binding"
fi
### CLEAN UP #############################################################################################
#delete the assettags.csv
rm "$csvPath"
#update Jamf Pro inventory
jamf recon
