Temporary Admin Elevation Script for macOS with Automatic Reversion
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 03-10-2025 08:16 AM
Hey everyone,
I wanted to share a Bash script that allows standard users to temporarily gain admin rights for 15 minutes via Self Service in Jamf Pro. This script ensures users can perform admin-required tasks while maintaining security, compliance, and auditability.
What This Script Does
- 1.Checks if the user is already an admin and notifies them if they don’t need elevation.
- 2. Prompts the user for a valid ServiceNow Request Number before granting admin access.
- 3.Logs the ServiceNow Request Number along with the username for auditing purposes.
- 4.Grants admin privileges to the user and creates a flag file for tracking.
- 5.Deploys a LaunchDaemon that ensures admin access is revoked after 15 minutes, regardless of reboots or logouts.
- 6.Starts logging all user activity for the duration of admin access, capturing executed commands for review.
- 7. At the end of 15 minutes, the script automatically revokes admin rights, stops logging, and collects logs for audit purposes.
Key Features
• Ensures admin rights are automatically revoked after 15 minutes.
•Tracks user activity during the elevated session to prevent misuse.
• Requires a ServiceNow Request Number, enforcing an approval process.
• Works even if the user logs out or reboots, thanks to the LaunchDaemon.
•Fully automated reversion of admin rights, eliminating manual intervention.
How to Customize the Script
1. Change the Admin Duration
•Modify StartInterval -integer 900 (900 seconds = 15 minutes) in the LaunchDaemon if you want a longer or shorter admin session.
2. Adjust ServiceNow Validation
•The script enforces a specific ServiceNow Request Number format (REQ1234567). You can modify this check to fit your organization’s ticketing system requirements.
3. Modify Logging Behavior
•The script logs all executed commands using log stream --predicate 'eventMessage contains "exec"'. You can adjust this to capture different system events.
4. Customize the End-of-Session Actions
•Currently, the script revokes admin rights and collects logs after 15 minutes. You can modify it to trigger additional actions, such as alerting IT or sending an email notification.
Implementation Steps
- 1.Deploy the script via Jamf Pro Self Service.
- 2.Ensure users can run the script but do not have direct admin rights.
- 3.Deploy it in a test environment first before rolling out to production.
- 4.Monitor logs to ensure compliance and security.
This script strikes a balance between user autonomy and security, allowing temporary admin elevation while ensuring accountability and automatic reversion. Let me know if you have any questions or improvements!
Script:
#!/bin/bash
###############################################
# Temporary Admin Elevation Script for macOS #
# Grants admin rights for 15 minutes and #
# automatically revokes them afterward. #
###############################################
# Get the currently logged-in user
currentUser=$(who | awk '/console/{print $1}')
# Check if the user is already an admin
if id -nG "$currentUser" | grep -qw "admin"; then
echo "User $currentUser already has admin access."
osascript -e 'display dialog "User '"$currentUser"' already has administrative access.\n\nNo further action is needed." buttons {"OK"} default button "OK"'
exit 0
fi
# Prompt for ServiceNow Request Number
serviceNowNumber=""
while [[ -z "$serviceNowNumber" || ! "$serviceNowNumber" =~ ^[Rr][Ee][Qq][0-9]{7}$ ]]; do
serviceNowNumber=$(osascript -e '
try
set userInput to text returned of (display dialog "You will be granted administrative rights for 15 minutes. DO NOT ABUSE THIS PRIVILEGE.\n\nAll activity will be logged during this time. If misuse is detected, this privilege will not be granted again.\n\nA ServiceNow request ticket is required. Please enter your ServiceNow Request Number below to proceed." default answer "" buttons {"Cancel", "Agree and Continue"} default button "Agree and Continue")
if userInput is "" then return ""
else return userInput
end if
on error return "CANCELLED"
end try
')
# Exit if user cancels
if [[ "$serviceNowNumber" == "CANCELLED" ]]; then
echo "User cancelled admin access request."
exit 0
fi
# Validate ServiceNow Request Number format
if [[ -z "$serviceNowNumber" || ! "$serviceNowNumber" =~ ^[Rr][Ee][Qq][0-9]{7}$ ]]; then
osascript -e 'display dialog "ServiceNow requests must start with ‘REQ’ and have a total of 10 digits.\n\nEnsure that you opened a request, not an incident (INC)." buttons {"OK"} default button "OK"'
serviceNowNumber=""
fi
done
# Log the request
echo "User $currentUser provided ServiceNow Request Number: $serviceNowNumber" | tee -a /var/log/makemeadmin.log
# Create LaunchDaemon for automatic admin removal
sudo defaults write /Library/LaunchDaemons/removeAdmin.plist Label -string "removeAdmin"
sudo defaults write /Library/LaunchDaemons/removeAdmin.plist ProgramArguments -array -string /bin/sh -string "/Library/Application Support/JAMF/removeAdminRights.sh"
sudo defaults write /Library/LaunchDaemons/removeAdmin.plist StartInterval -integer 900
sudo defaults write /Library/LaunchDaemons/removeAdmin.plist RunAtLoad -boolean yes
sudo chown root:wheel /Library/LaunchDaemons/removeAdmin.plist
sudo chmod 644 /Library/LaunchDaemons/removeAdmin.plist
launchctl load /Library/LaunchDaemons/removeAdmin.plist
sleep 10
# Create tracking directory
if [ ! -d /private/var/userToRemove ]; then
mkdir /private/var/userToRemove
fi
echo $currentUser >> /private/var/userToRemove/user
# Grant admin privileges
/usr/sbin/dseditgroup -o edit -a $currentUser -t user admin
# Start logging user activity
logFile="/var/log/makemeadmin-$(date '+%Y-%m-%d_%H-%M-%S').log"
sudo log stream --predicate 'eventMessage contains "exec"' --style syslog > "$logFile" 2>&1 &
echo $! > /private/var/userToRemove/loggingPID
# Script for revoking admin rights
cat << 'EOF' > /Library/Application\ Support/JAMF/removeAdminRights.sh
if [[ -f /private/var/userToRemove/user ]]; then
userToRemove=$(cat /private/var/userToRemove/user)
echo "Removing $userToRemove's admin privileges"
/usr/sbin/dseditgroup -o edit -d $userToRemove -t user admin
rm -f /private/var/userToRemove/user
launchctl unload /Library/LaunchDaemons/removeAdmin.plist
rm /Library/LaunchDaemons/removeAdmin.plist
log collect --last 15m --output /private/var/userToRemove/$userToRemove.logarchive
# Stop logging user activity
if [[ -f /private/var/userToRemove/loggingPID ]]; then
kill $(cat /private/var/userToRemove/loggingPID)
rm -f /private/var/userToRemove/loggingPID
fi
fi
EOF
exit 0
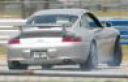
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 03-10-2025 12:02 PM
Not to dissuade sharing this approach but https://github.com/SAP/macOS-enterprise-privileges is somewhat easier to configure for different classes of users (and if you're a Jamf Connect customer the option to allow temporary elevation to admin is built-in).
- Mark as New
- Bookmark
- Subscribe
- Mute
- Subscribe to RSS Feed
- Permalink
- Report Inappropriate Content
Posted on 03-11-2025 07:36 AM
This is great and easy if you don't need InfoSec approval to allow admin control per user, which we do. That's why it asked for the Service Now request number and logged everything the user did.
